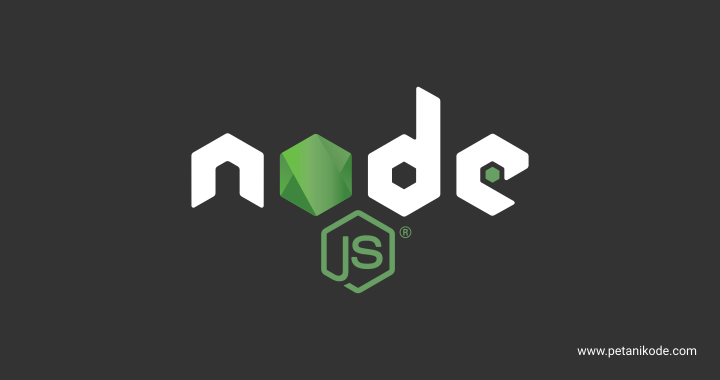
In the previous tutorial, we have studied the HTTP module which is a module for creating web servers and doing HTTP Requests .
The following are examples of programs that have been created:
var http = require('http');
http.createServer(function (request, response) {
response.writeHead(200, {'Content-Type': 'text/html'});
response.write('Hello <b>World</b>!');
response.end();
}).listen(8000);
console.log("server running on http://localhost:8000");
In this code, we do hard coding of HTML code (write directly HTML code in nodejs code).
response.write('Hello <b>World</b>!');
What if there is an HTML file that we want to send in return?
To do this we need help from the module file system .
The process will be like this:
- Receive requests from users;
- Read HTML files on the server;
- Send a reply to the user.
In number two, we will use the file system module .
Okay, for more details ...
Let's start.
Read Files at Nodejs
In Nodejs, there is a file system (
fs
) module that allows us to access the file system .
File system modules are often used for: 1
- Read file;
- Write file;
- Rename file;
- delete file;
- etc.
This module is already in Nodejs, so we don't need to install it via the NPM (Node Package Manager) . 2
To be able to use the module
fs
we must import it first with the function require()
.var fs = require('fs');
Let's try reading the file with the module
fs
. First, please create an HTML file with the name index.html
and contents as follows:<!DOCTYPE html>
<html>
<head>
<title>Tutorial Nodejs</title>
</head>
<body>
<h1>Tutorial Nodejs Petanikode</h1>
<p>Belajar Baca Tulis File di Nodejs</p>
</body>
</html>
Then create a new javacript code named
baca_file.js
with the contents as follows:var fs = require('fs');
var http = require('http');
http.createServer(function (request, response) {
// baca file
fs.readFile('index.html', (err, data) => {
if (err) throw err;
// kirim respon
response.writeHead(200, {'Content-Type': 'text/html'});
response.write(data);
response.end();
});
}).listen(8000);
console.log("server running on http://localhost:8000");
Make sure the JavaScript and HTML files are in one folder.
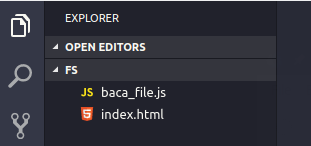
After that, try executing
baca_file.js
:node baca_file.js
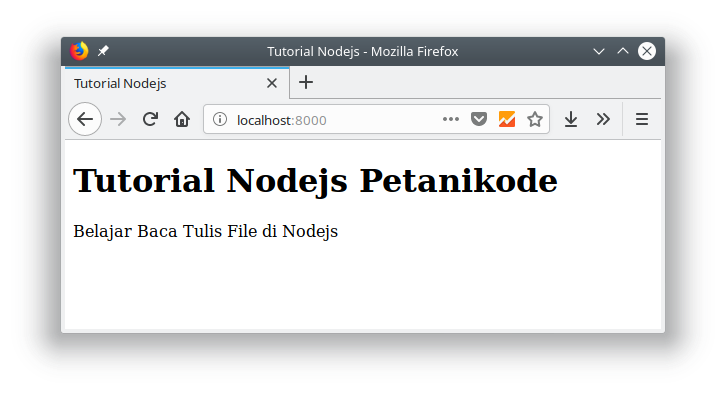
Yep! 
We have successfully created a Nodejs server and sent an HTML file in return.
What should be noted in the code above is in this section:
// baca file
fs.readFile('index.html', (err, data) => {
if (err) throw err;
// kirim respon
response.writeHead(200, {'Content-Type': 'text/html'});
response.write(data);
response.end();
});
Method
readFile()
is a method used to read files. Then this method has two parameters:- File name;
- Functions that will be executed when the file is read.
(err, data) => { ... }
The parameter
err
will contain an error when the file fails to read and the parameter data
will contain data from the file.
Therefore, in this function we send a response to the client with parameters
data
.// kirim respon
response.writeHead(200, {'Content-Type': 'text/html'});
response.write(data);
response.end();
Then the response that will be received by the client will be in accordance with the contents of the file
index.html
.Make a File on Nodejs
In addition to reading files, modules are
fs
also used to create new files. There are several methods that can be used to create files: 1fs.appendFile()
to create and fill in files;fs.open()
to make, open, and write files;fs.writeFile()
to make and write files.
Let's try one by one.
First, please create a javascript file with the name
fs_append.js
as follows:var fs = require('fs');
//create a file named mynewfile1.txt:
fs.appendFile('mynewfile1.txt', 'Hello dari Petanikode!', function (err) {
if (err) throw err;
console.log('Saved!');
});
The program code above will create a file with a name
mynewfile1.txt
with contents Hello dari petanikode!
.
Let's try execution:
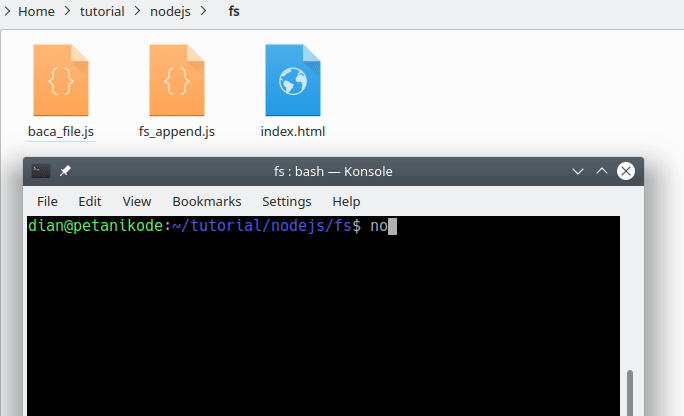
If we execute again
node fs_append.js
, then the contents of the file mynewfile1.txt
will increase to:Hello dari Petanikode!Hello dari Petanikode!
If we execute 10 times, the text
Hello dari Petanikode!
will be added 10 times.
This is the nature of the method
fs.appendFile()
, it will always add the contents of the file to each execution.Make a file with the function fs.open ()
Then the next function / method is
fs.open()
. This function has two functions, namely to open and write files.
Let's try ...
Make a new javascript file with the name
fs_open.js
as follows:var fs = require('fs');
fs.open('mynewfile2.txt', 'w', function (err, file) {
if (err) throw err;
console.log('Saved!');
});
Then try executing:
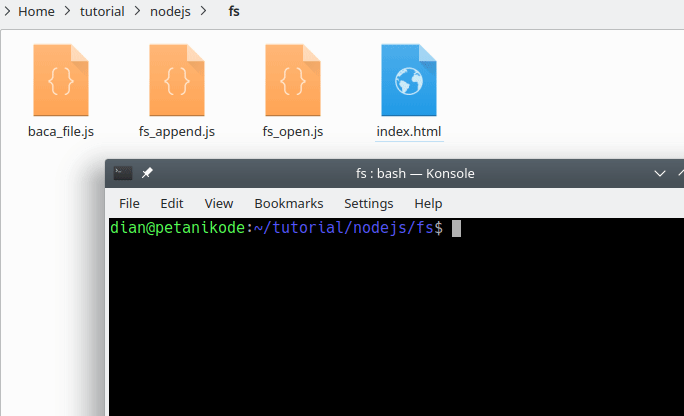
The function
fs.open()
has three parameters:- File name;
- Flag;
- The function that will be executed when the file is opened.
In the example above, we give Flag
w
(write) to tell the function fs.open()
if we want to write or create a new file.
Then the function
fs.open()
will make the file empty if there is no file with the specified name.
However, if there is already a file, the function
fs.open()
will overwrite it.
Then when we want to read only the file, then we can give the flag
r
(read) .r
open file to read. If the file does not exist, an error will occur.r+
open file to read and write. If the file does not exist, an error will occur.rs
open file to read in synchronous mode .rs+
open files to read and write in synchronous mode.w
open e-file for writing.wx
same asw
, but it will error if there is already a file.w+
open the file to be written and read.wx+
same asw+
but it will be an error if there is already a file.a
open file to fill.ax
same asa
but it will be an error if there is already a file.a+
open file to fill.ax+
same asa+
but it will be an error if there is already a file.
To understand it, let's try creating a program to write and read files.
Means the flag that can be used is
w+
, because we will write first and then read it.
Make a file called Beru
fs_open_wr.js
with the contents as follows:var fs = require('fs');
fs.open('mynewfile2.txt', 'w+', function (err, file) {
if (err) throw err;
// kontent yang akan kita tulis ke file
let content = "Hello Petanikode!";
// tulis konten ke file
fs.writeFile(file, content, (err) => {
if (err) throw err;
console.log('Saved!');
});
// baca file
fs.readFile(file, (err, data) => {
if (err) throw err;
console.log(data.toString('utf8'));
});
});
In the code above, we will write to the file
mynewfile2.txt
. There are two functions fs
that we use:fs.writeFile()
to write to file;- and
fs.readFile()
to read files.
In the function
fs.readFile()
, we use a function toString('utf8')
to convert the buffer to text by encoding UTF-8.
Now try executing the program:
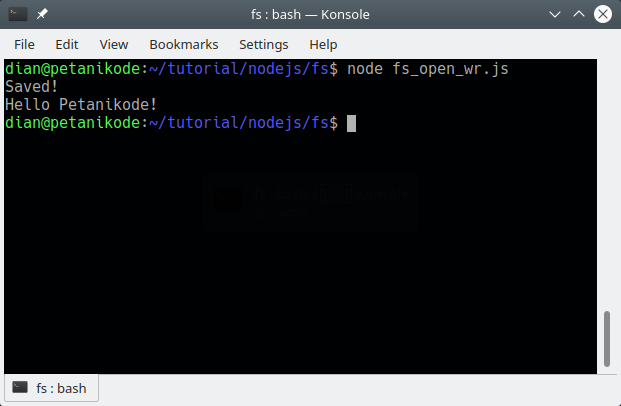
As we expected, the file was successfully written and read.
Change the File Name with Nodejs
After we try to read and write files in Nodejs, next we try to rename the file.
In the module
fs
there is a function rename()
to change the name.
This function has three parameters:
- File name;
- New name;
- The function that will be executed when the name is changed.
Let's try ...
Create a new javascript file with the name
rename.js
and fill in the following code:var fs = require('fs');
fs.rename('mynewfile1.txt', 'myrenamedfile.txt', function (err) {
if (err) throw err;
console.log('File Renamed!');
});
After that, try executing the program:
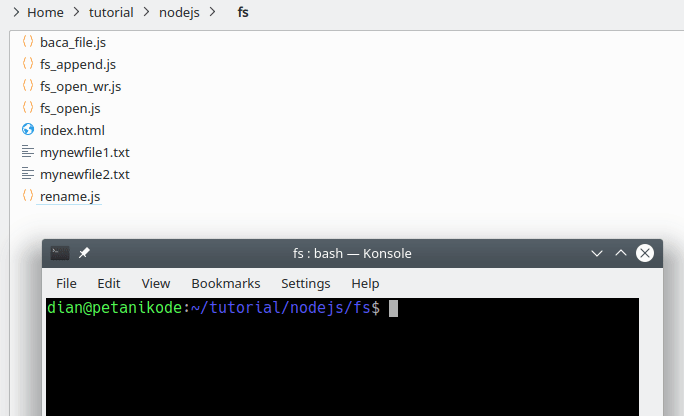
Deleting Files with Nodejs
The module
fs
has a function unlink()
to delete files. This function has two parameters:- The file name to be deleted;
- function that will be executed when the file is deleted.
Let's try ...
Make a new file named
delete.js
, then fill in the following code:var fs = require('fs');
fs.unlink('mynewfile2.txt', function (err) {
if (err) throw err;
console.log('File deleted!');
});
In the program code above, we will delete the file
mynewfile2.txt
. Let's try execution: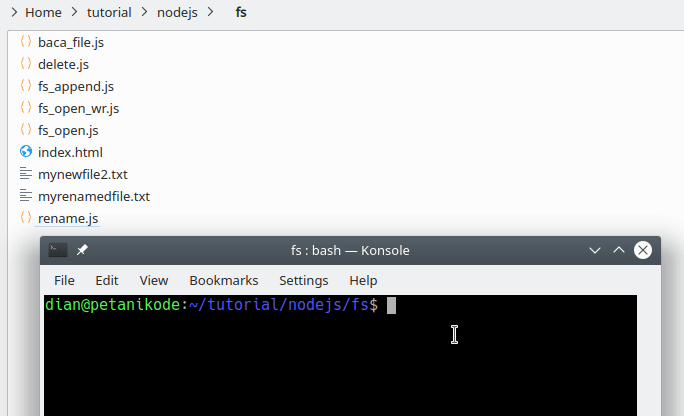
Reference: https://www.petanikode.com/nodejs-file/
0 Komentar untuk "Learning Nodejs # 5: How to Use the File System Module to Read Write Files"
Silahkan berkomentar sesuai artikel