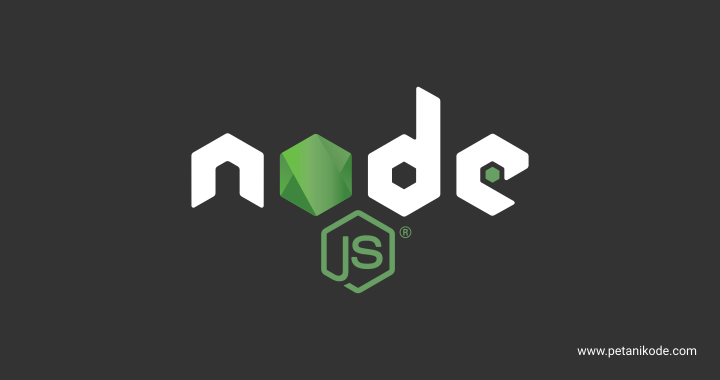
The HTTP module is a module used to work with HTTP protocol, usually used to create servers.
At the beginning of the introduction of Nodejs , we have already discussed this module to only create web servers.
Now we will discuss more deeply. So we can use it to:
- Bolt Server
- Retrieves URLs for routing
- Retrieve String Queries
Let's start…
Creating a Server with an HTTP module
The HTTP module is a build-in module in Nodejs that we can use directly without having to install it from NPM.
Every time we want to use the HTTP module, we have to import it first like this:
var http = require('http');
After that we can make programs for servers like this:
File:
server.js
var http = require('http');
var server = http.createServer(function (request, response) {
response.end("Hi, selamat datang di nodejs");
});
server.listen(8000);
console.log("server running on http://localhost:8000");
Then we execute with the command
node server.js
: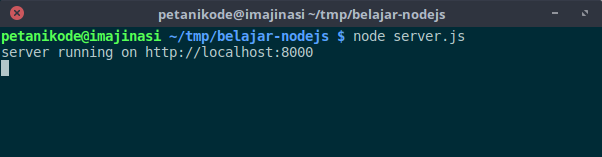
Then the result:
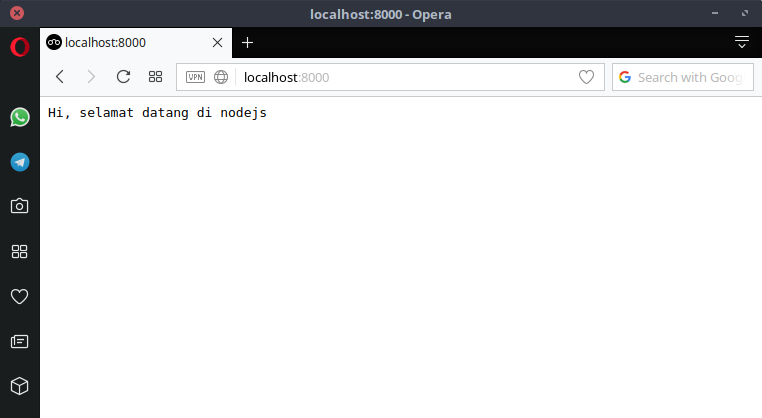
It's easy, isn't it?
Anyway, the default response given by the server is in
text/plain
.
If we want to change it, we can do it in the Response Header .
What is Response Header ?
Continue reading down ...
Modification of Response Body and Headers
Response Body and Response Header is the payload data that we will send to the client .
This data we can modify with objects
response
.
Example:
var http = require('http');
http.createServer(function (request, response) {
response.writeHead(200, {'Content-Type': 'text/html'});
response.write('Hello <b>World</b>!');
response.end();
}).listen(8000);
console.log("server running on http://localhost:8000");
In the example above, we use a method
writeHead()
to change the type of content we will send to the client . Then use a method write()
to write the body or content to be sent.
In the example above, we will send the type of HTML content with the response code
200
, meaning the response is good.
Finally call the method
response.end()
to end the response.
Then the results will be like this:
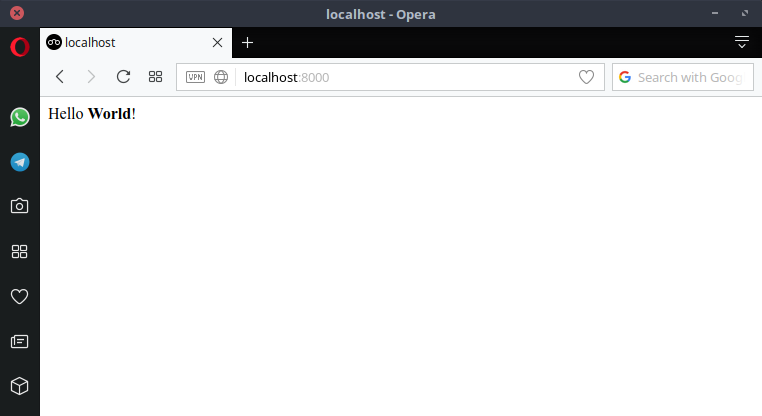
We can also change the type of coent to JSON, XML, PDF, etc.
Example:
// untuk JSON
response.writeHead(200, {'Content-Type': 'application/json'});
// untuk PDF
response.writeHead(200, {'Content-Type': 'application/pdf'});
// untuk XML
response.writeHead(200, {'Content-Type': 'application/xml'});
Let's try sending a response in JSON form.
Change the code to something like this:
var http = require('http');
http.createServer(function (request, response) {
response.writeHead(200, {'Content-Type': 'application/json'});
response.write('{"message": "Hello World!"}');
response.end();
}).listen(8000);
console.log("server running on http://localhost:8000");
Then the result:
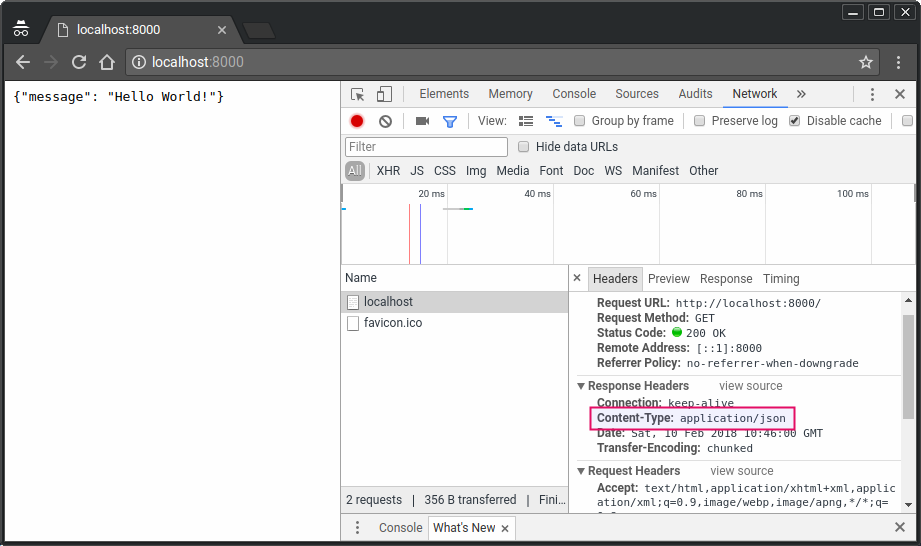
Retrieves the URL for Routing
If we want to take an URI to create a routing URL, then we can take it from the object
request
with the property url
.
Example:
var http = require('http');
http.createServer(function (request, response){
response.writeHead(200, {'Content-Type': 'text/html'});
response.write('URL: ' + request.url);
response.end();
}).listen(8000);
console.log('Server running on http://localhost:8000');
The result:
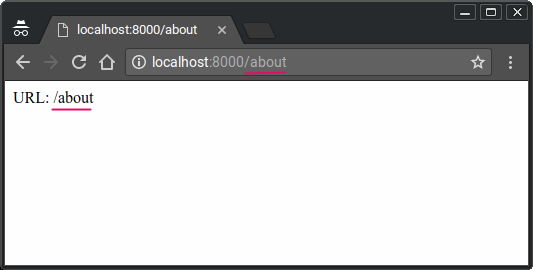
From this value, we can make a router, for example when people open this route, then run this function.
Example:
var http = require('http');
http.createServer(function (request, response){
response.writeHead(200, {'Content-Type': 'text/html'});
switch(request.url){
case '/about':
response.write("Ini adalah halaman about");
break;
case '/profile':
response.write("Ini adalah halaman profile");
break;
case '/produk':
response.write("ini adalah halaman produk");
break;
default:
response.write("404: Halaman tidak ditemukan");
}
response.end();
}).listen(8000);
console.log('Server running on http://localhost:8000');
The result:
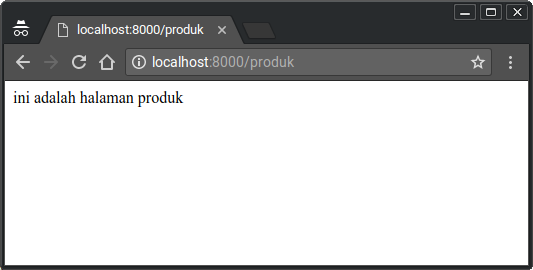
If we open a router that has not been defined, it will be displayed
default
or 404
.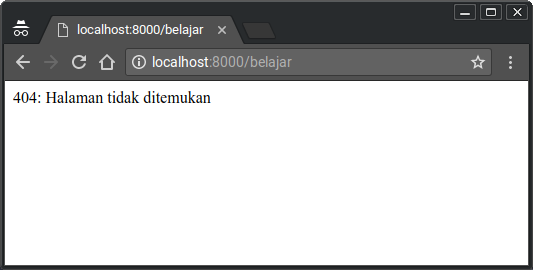
How to Take a String Query at Nodejs
We already know how to make a router from the URL, now what if there is a query string on the router like this:
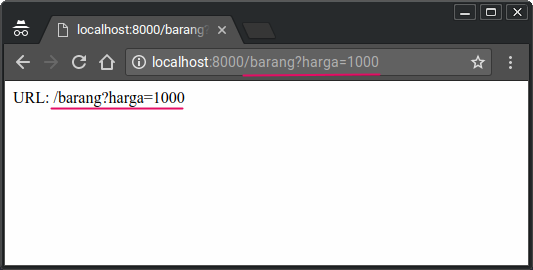
Text that exists after the question mark (
?
) is called a query string.
example:
?harga=1000
How do we take that value?
To do this, we can use the module
url
.
Example:
var http = require('http');
var url = require('url');
http.createServer(function (request, response) {
response.writeHead(200, { 'Content-Type': 'text/html' });
var q = url.parse(request.url, true).query;
var txt = 'Kata kunci: ' + q.keyword;
response.end(txt);
}).listen(8000);
console.log("Server berjalan di http://localhost:8000")
The result:
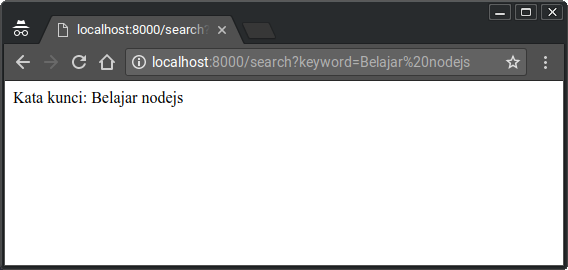
Note these contents:
var q = url.parse(request.url, true).query;
On that line, we parse the URL with the module
url
. Then take property query
.
So the variable
q
will be an object that contains something like this:{ keyword: 'Belajar nodejs' }
Thus, we can take value
'Belajar nodejs'
through property keyword
.console.log(q.keyword); // 'Belajar nodejs'
Reference: https://www.petanikode.com/nodejs-http
0 Komentar untuk "Learning Nodejs # 4: Understanding How to Use the HTTP Module"
Silahkan berkomentar sesuai artikel