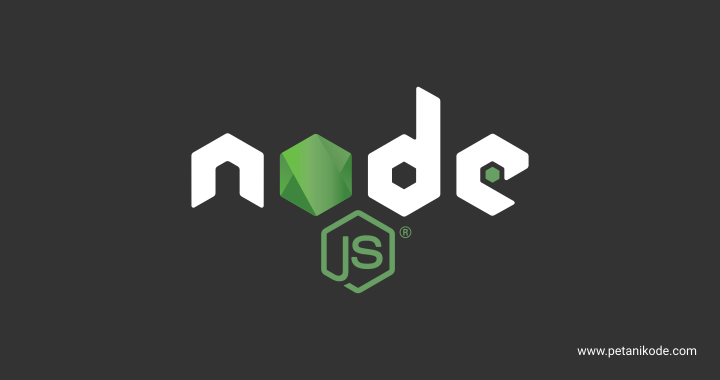
When developing applications with Nodejs, we will not be able to escape the use of Modules.
Sometimes it can also slow down the development process , because there are so many modules to choose from.
(The programmer is upset, because he can't determine the right module
)
Currently Nodejs has millions of modules distributed in packages.
On this occasion, we will learn how to use the Nodejs module starting from:
- Understanding Module Nodejs;
- Using the Build-in Module ;
- Using Modules from NPM;
- Making Your Own Module.
Let's start…
What are Modules in Nodejs?
Modules can be likened to a library that contains functions to be used in an application. So we don't need to make a function from scratch.
Before it can be used, the module must be imported first.
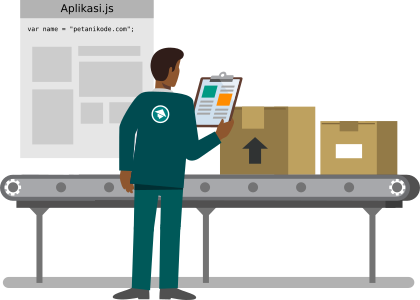
The Nodejs module is distributed in packages that can be downloaded and installed with package managers such as NPM and Yarn.
The module installed with the package manager will be defined in the file
package.json
and package-lock.json
.Using the Build-in Nodejs Module
Nodejs has many built -in modules that we can use to create applications.
We don't need to install these modules with NPM, because it's been provided since we installed Nodejs .
Examples of some default modules:
http
to do an HTTP Request and create an HTTP server.fs
to work with system files.url
for parsing strings from URLs.querystring
to work string queries .os
provide information about the operating system.- etc.
How to import the build-in module into the application is to use the function
require()
.
Example:
var http = require('http');
Meaning : we will import the module
http
and then create an object http
to hold the module.
Once imported, then the module can be used like this:
http.createServer(function (req, res) {
res.writeHead(200, {'Content-Type': 'text/html'});
res.end('Hello World!');
}).listen(8080);
Using Modules from NPM
How to use modules from NPM, just like using the build-in module .
But ...
We must first install the module with NPM, then we can import it with functions
require()
.
Let's try it.
Create a new project with a name
belajar-modul-nodejs
.mkdir belajar-modul-nodejs
cd belajar-modul-nodejs/
npm init --yes
Description : The parameter
--yes
works to create files package.json
by default.
After that, please install the desired module.
npm install moment
Once installed, there will be a new directory named
node_modules
.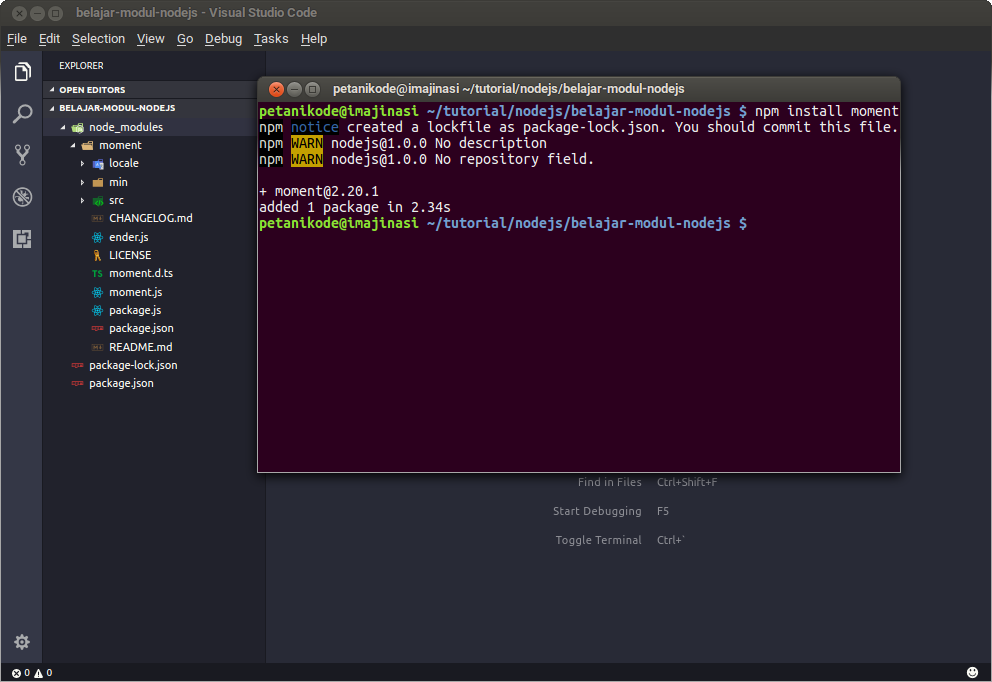
That's where all the modules installed with NPM will be saved.
Next, please create a file
index.js
with the contents as follows.// impor modul mementjs
var moment = require("moment");
// menggunakan modul momentjs
console.log("Sekarang: " + moment().format('D MMMM YYYY, h:mm:ss a'));
Try running with the command:
node index.js
Then the results will be like this:
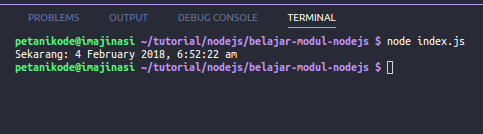
Question: Does the Module object name have to be the same as the Module name?
Not really, the name of the variable or object of the module may not be the same as the name of the module.
Suppose like this:
var server = require("http");
However, to make it easier, it should be equated.
Making Your Own Module
When the modules we need are not in build-in and NPM, then we have to make it ourselves.
How to make a Nodejs module is very easy.
Just make a function and then export.
Example:
salam.js
exports.salamPagi = function () {
return "Selamat Pagi!";
};
We must register functions into object properties
exports
so that they can be used outside the file.
After that, we can import.
For example we will use the file
index.js
.// impor modul mementjs dan salam
var moment = require("moment");
var salam = require("./salam");
// menggunakan modul
console.log(salam.salamPagi());
console.log("Sekarang: " + moment().format('D MMMM YYYY, h:mm:ss a'));
When we execute the file
index.js
, it will produce something like this: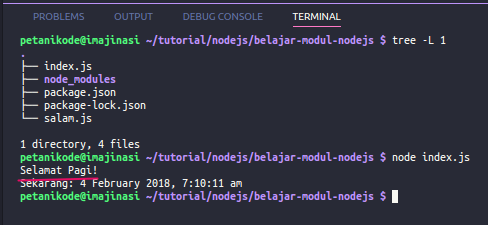
The final word…
There are lots of Nodejs modules that we should try. All Nodejs modules can be found on the NPM website .
Understanding how to use Modules in Nodejs, it is very helpful to learn Nodejs to the next step.
If someone is asked ...
... let's say it through comments!
Reference: https://www.petanikode.com/nodejs-module/
0 Komentar untuk "Learning Nodejs # 3: How to Use Modules in the Nodejs Application"
Silahkan berkomentar sesuai artikel