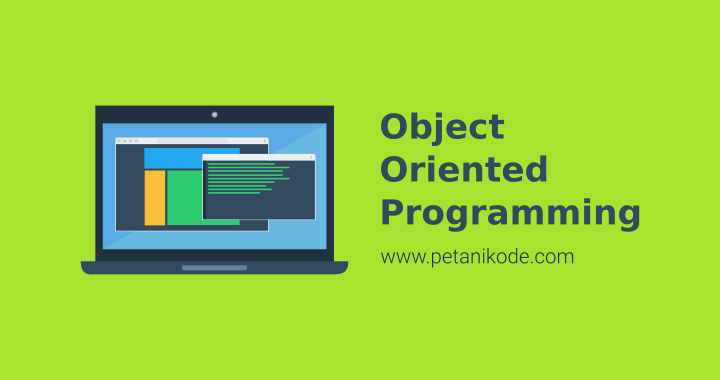
One form of the relationship is inheritance . This relationship is like a family relationship between parents and children.
A class in Java, can have one or more offspring or child classes. Child classes will have inheritance properties and methods from the mother class.
In this tutorial we will learn:
- Why do we have to use inheritance ?
- Examples of inheritance programs
- Method Overriding
Let's start…
Why Should We Use Inheritance?
For example, in Games, we will create enemy classes with different behaviors.
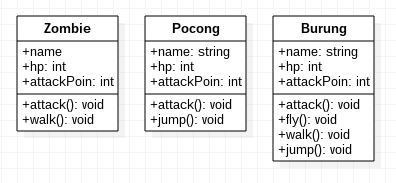
Then we make the code for each class like this:
File:
Zombie.java
class Zombie {
String name;
int hp;
int attackPoin;
void attack(){
// ...
}
void walk(){
//...
}
}
File:
Pocong.java
class Pocong {
String name;
int hp;
int attackPoin;
void attack(){
// ...
}
void jump(){
//...
}
}
File:
Burung.java
class Burung {
String name;
int hp;
int attackPoin;
void attack(){
// ...
}
void walk(){
//...
}
void jump(){
//...
}
void fly(){
//...
}
}
Can it be like this?
Yes, it's okay. But it won't work, because we repeatedly write the same properties and methods.
What's the solution?
We must use inheritance .
Let's look at the same class:
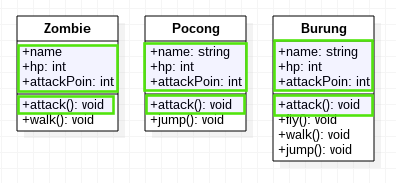
After using inheritance , it will be like this:
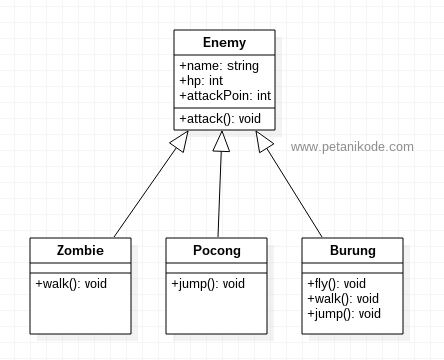
Anyway, inheritance in StarUML is described by Generalization linkages .
Class
Enemy
is a class parent who has a child Zombie
, Pocong
andBurung
. Whatever property is in the parent class, it will also be owned by the child class.
Then what is the code in Java?
The code will look like this:
File:
Enemy.java
class Enemy {
String name;
int hp;
int attackPoin;
void attack(){
System.out.println("Serang!");
}
}
In the child class, we use keywords
extends
to declare that they are derived from Enemy
.
File:
Zombie.java
class Zombie extends Enemy {
void walk(){
System.out.println("Zombie jalan-jalan");
}
}
File:
Pocong.java
class Pocong extends Enemy {
void jump(){
System.out.println("loncat-loncat!");
}
}
File:
Burung.java
class Burung extends Enemy {
void walk(){
System.out.println("Burung berjalan");
}
void jump(){
System.out.println("Burung loncat-loncat");
}
void fly(){
System.out.println("Burung Terbang...");
}
}
Then, if we want to create objects from these classes, we can make it like this:
Enemy monster = new Enemy();
Zombie zumbi = new Zombie();
Pocong hantuPocong = new Pocong();
Burung garuda = new Burung();
Example of Inheritance Program
After understanding the concept of inheritance , now let's make an example of a simple program.
The program we will create to function is to calculate the area and circumference of a flat building.
Form the class diagram like this:
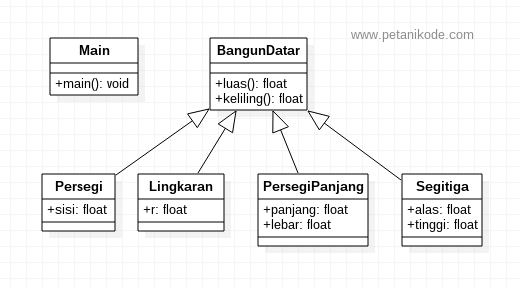
Okay, let's make all the classes.
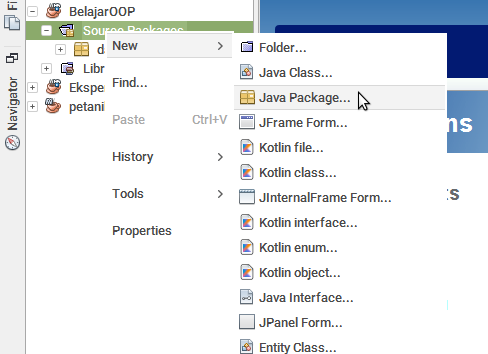
Fill in the package name with inheritance .
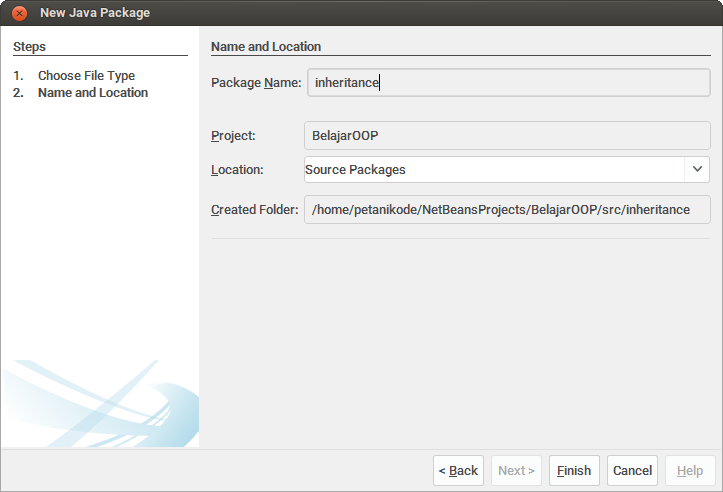
Next make new classes based on the diagram above.
File:
inheritance/BangunDatar.java
package inheritance;
public class BangunDatar {
float luas(){
System.out.println("Menghitung laus bangun datar");
return 0;
}
float keliling(){
System.out.println("Menghitung keliling bangun datar");
return 0;
}
}
File:
inheritance/Persegi.java
package inheritance;
public class Persegi extends BangunDatar {
float sisi;
}
File:
inheritance/Lingkaran.java
package inheritance;
public class Lingkaran extends BangunDatar{
// jari-jari lingkaran
float r;
}
File:
inheritance/PersegiPanjang.java
package inheritance;
public class PersegiPanjang extends BangunDatar {
float panjang;
float lebar;
}
File:
inheritance/Segitiga.java
package inheritance;
public class Segitiga extends BangunDatar {
float alas;
float tinggi;
}
File:
inheritance/Main.java
package inheritance;
public class Main {
public static void main(String[] args) {
// membuat objek bangun datar
BangunDatar mBangunDatar = new BangunDatar();
// membuat objek persegi dan mengisi nilai properti
Persegi mPersegi = new Persegi();
mPersegi.sisi = 2;
// membuat objek Lingkaran dan mengisi nilai properti
Lingkaran mLingkaran = new Lingkaran();
mLingkaran.r = 22;
// membuat objek Persegi Panjang dan mengisi nilai properti
PersegiPanjang mPersegiPanjang = new PersegiPanjang();
mPersegiPanjang.panjang = 8;
mPersegiPanjang.lebar = 4;
// membuat objek Segitiga dan mengisi nilai properti
Segitiga mSegitiga = new Segitiga();
mSegitiga.alas = 12;
mSegitiga.tinggi = 8;
// memanggil method luas dan keliling
mBangunDatar.luas();
mBangunDatar.keliling();
mPersegi.luas();
mPersegi.keliling();
mLingkaran.luas();
mLingkaran.keliling();
mPersegiPanjang.luas();
mPersegiPanjang.keliling();
mSegitiga.luas();
mSegitiga.keliling();
}
}
After that, try running the class
Main
, the result: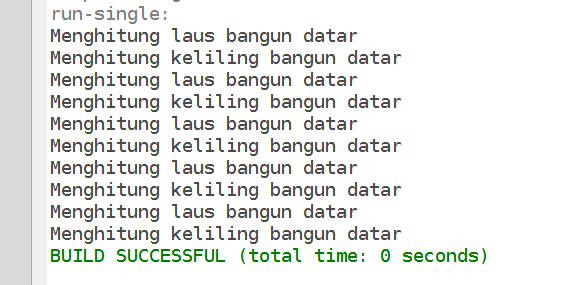
Why are the results so?
Because what we are calling is actually the method
luas()
and keliling()
the parent ( BangunDatar
).
The object of the child
BangunDatar
has not had a method luas()
and keliling()
, finally taking possession of its parent.
Then what if we want to make all child classes have methods
luas()
and keliling()
different from the parent?
The answer: use the overriding method .
Method Overriding
The Overriding method is done when we want to recreate a method in a child sub-class or class.
Overriding method can be created by adding annotations
@Override
above the method name or before the method creation.
Example:
Persegi.java
class Persegi extends BangunDatar {
float sisi;
@Override
float luas(){
float luas = sisi * sisi;
System.out.println("Luas Persegi: " + luas);
return luas;
}
@Override
float keliling(){
float keliling = 4 * sisi;
System.out.println("Keliling Persegi: " + keliling);
return keliling;
}
}
This means that we rewrite the method
luas()
and keliling()
in the child class.
Now let's create an overriding method for all child classes.
File:
Lingkaran.java
package inheritance;
public class Lingkaran extends BangunDatar{
// jari-jari lingkaran
float r;
@Override
float luas(){
float luas = (float) (Math.PI * r * r);
System.out.println("Luas lingkaran: " + luas);
return luas;
}
@Override
float keliling(){
float keliling = (float) (2 * Math.PI * r);
System.out.println("Keliling Lingkaran: " + keliling);
return keliling;
}
}
In the formula for area and circumference of a circle, we can use constants
Math.PI
as PI values. This constant already exists in Java.
File:
PersegiPanjang.Java
package inheritance;
public class PersegiPanjang extends BangunDatar {
float panjang;
float lebar;
@Override
float luas(){
float luas = panjang * lebar;
System.out.println("Luas Persegi Panjang:" + luas);
return luas;
}
@Override
float keliling(){
float kll = 2*panjang + 2*lebar;
System.out.println("Keliling Persegi Panjang: " + kll);
return kll;
}
}
File:
Segitiga.java
package inheritance;
public class Segitiga extends BangunDatar {
float alas;
float tinggi;
@Override
float luas() {
float luas = alas * tinggi;
System.out.println("Luas Segitiga: " + luas);
return luas;
}
}
For classes
Segitiga
, we only override the method luas()
. Because for methods keliling()
, triangles have different formulas.
... or perhaps could be lowered again be the class of this triangle:
SegitigaSiku
, SegitigaSamaKaki
, SegitigaSamaSisi
, etc.
Now let's try executing the class
Main
again: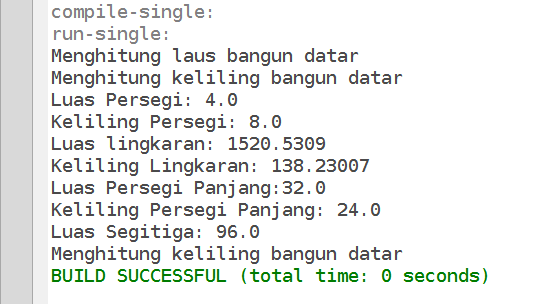
Wait a minute!
Earlier he said that he was not efficient writing repeatedly, is this it?
Yes at the beginning we write attributes of
Enemy
the same repeatedly. Unlike this one, we do write the same method. But the contents or formulas in the method are different.
We will discuss this later in Polymorphism (many forms).
0 Komentar untuk "Learning Java OOP: Understanding Inheritance and Method Overriding"
Silahkan berkomentar sesuai artikel