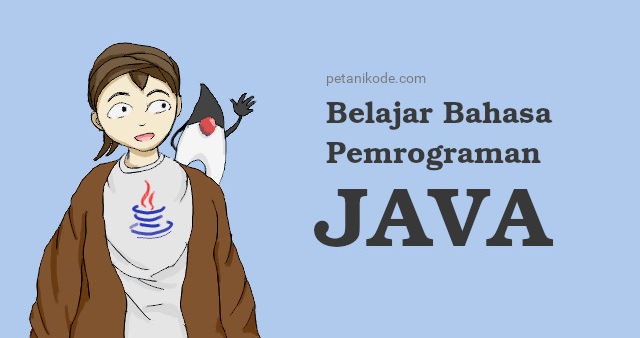
Classes in Java programs can be interconnected by giving access to their members.
Everything in the class (attributes and methods ) is called a member. Usually there will be an access level called a modifier.
In an inheritance relationship , all members in the parent class will be accessible by the child class (subclass), unless the memeber is given a private modifier .
Keep in mind:
Modifiers can not only be given to members. But it can also be given to interfaces, enums, and classes themselves.
Consider the following program code:
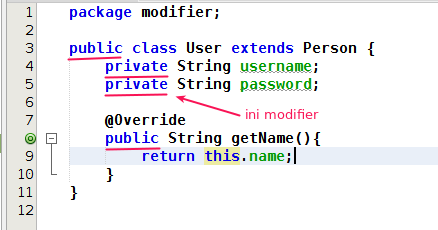
What I underline in the code above is the modifier. This modifier will later determine the member and class access limits.
There are 3 Kinds of Modifiers in Java
In general there are three kinds of modifier used in Java:
public
, private
, and protected
.
If we do not use these three keywords, the member or class does not use a modifier (no modifier) .
Each modifier will determine where members can be accessed.
The following is the range table for each modifier:
Modifier | Class | Package | Subclass | World |
---|---|---|---|---|
public | Y | Y | Y | Y |
protected | Y | Y | Y | N |
modifier no | Y | Y | N | N |
private | Y | N | N | N |
Information:
Y
meaning it can be accessed;N
meaning not accessible;Subclass
means child class;World
meaning all packages in the application.
In the table above ... if we don't use a modifier (no modifier), then the class and member will only be accessible from the Class itself and the package (the class that is in one package with it).
In order to be accessible from anywhere, we must provide a modifier
public
.
Let's look at an example ...
1. Public
Modifier
public
will make members and classes accessible from anywhere.
Example:
package modifier;
class Person {
public String name;
public changeName(String newName){
this.name = newName;
}
}
In the class
Preson
there are two members, namely:- attribute
name
- method
changeName()
We give both modifiers
public
. This means that they will be accessible from anywhere.
However,
Person
we don't give class modifiers. So what will happen is that the class cannot be imported (accessed) from outside the package.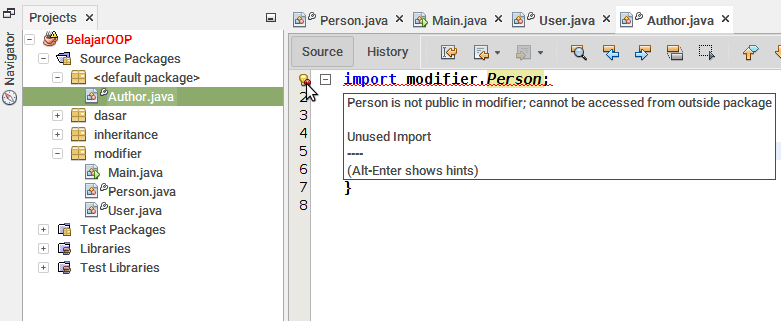
The class
Person
is in the package modifier
, then we try to access it from the default package , so what happens is an error like the picture above.
What is the solution so that it can be accessed from outside the package?
Yes we have to add modifiers
public
to the class Person
.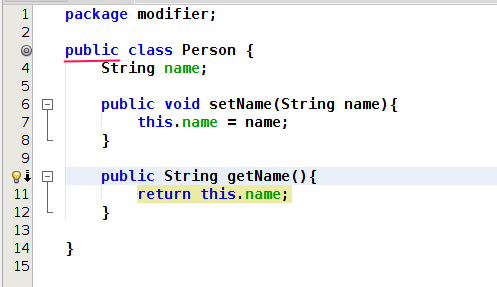
Then the error will disappear and the class
Person
will be imported from any package.
In the class diagram, the modifier is
public
represented by a plus ( +
) symbol .
Example:
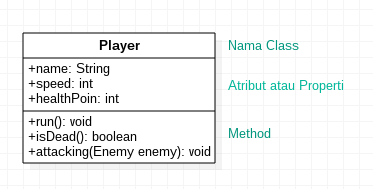
All members in the class
Player
have modifiers public
. Note the symbol +
in front of it.2. Private
Modifier
private
will make members only accessible from within the class itself.
Keep in mind:
Modifiers
private
cannot be given to classes, enums, and interfaces. Modifiers private
can only be given to member classes.
Example:
class Person {
private String name;
public void setName(name){
this.name = name;
}
public String getName(){
return this.name;
}
}
In the example above, we give a modifier
private
to the attributes name
and modifiers public
in the method setName()
and getName()
.
If we try to access the attribute directly
name
like this:Person mPerson = new Person()
mPerson.name = "Petani Kode"; // <- maka akan terjadi error di sini
Do not believe?
Try it in Netbeans:
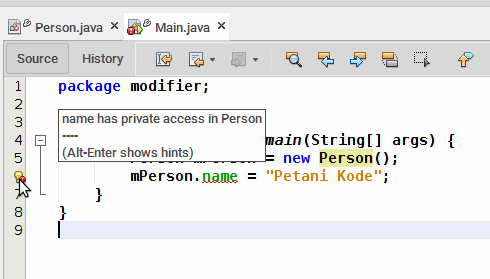
Then, how do you access members
private
from outside the class?
Example:
Person mPerson = new Person();
mPerson.setName("Petani Kode");
System.out.println("Person Name: " + mPerson.getName());
In the class diagram, the modifier is
private
represented by a minus ( -
) symbol .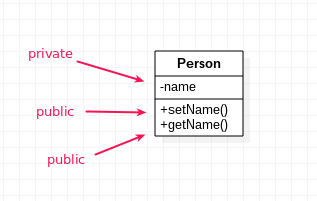
3. Protected
Modifier
protected
will make members and classes only accessible from:- Class itself;
- Sub class or child class;
- Package (class in one package with it).
Modifiers
protected
can only be used on members only.
Example:
package modifier;
public class Person {
protected String name;
public void setName(String name){
this.name = name;
}
public String getName(){
return this.name;
}
}
In the example above, we give a modifier
protected
to the attribute name
.
If we try to access from a class that is one package with it, there will be no error.
However, if we try to access from outside the package like this:
import modifier.Person;
public class Author {
Person p = new Person();
public Author() {
// akan terjadi error di sini karena atribut name
// telah diberikan modifier protected
p.name = "Petani Kode";
}
}
Then an error will occur.
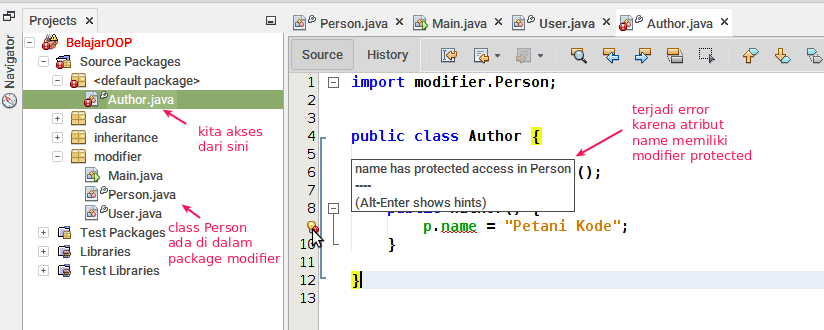
In the class diagram (in StarUML), the modifier is
protected
represented by a fence ( #
).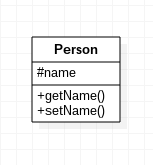
Sumber: https://www.petanikode.com/java-oop-modifier/
0 Komentar untuk "Learning Java OOP: Understanding Levels of Member and Class Access (Modifier)"
Silahkan berkomentar sesuai artikel