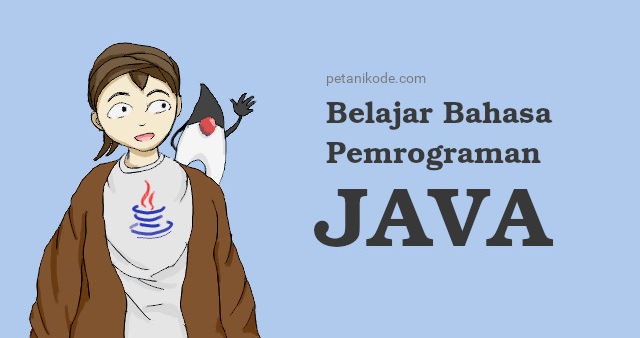
What is a Constructor?
A constructor is a special method that will be executed during an instance .
Usually this method is used to initialize or prepare data for objects.
Example of a constructor and how to make it
The following is a sample constructor:
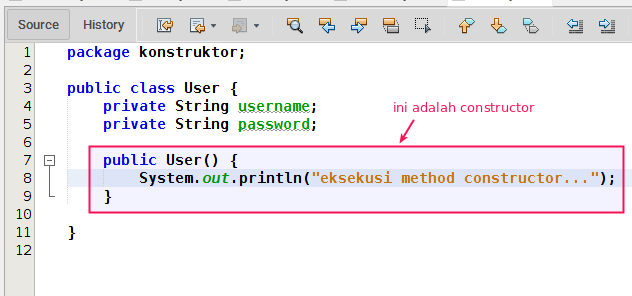
The way to make a constructor is to write the name of the constructor method the same as the class name.
In the example above the constructor is written like this:
public User() {
System.out.println("eksekusi method constructor...");
}
Make sure to provide a modifier
public
to the constructor, because he will be executed when the object creation (instance) .
Let's try to create a new object from the class
User
:User petani = new User();
So now we have a complete code like this:
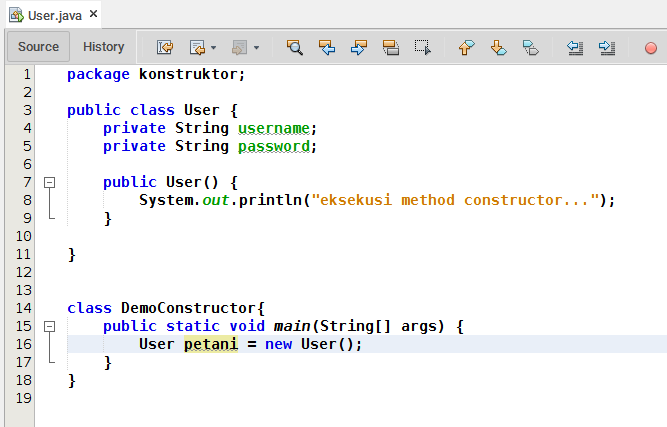
The result when executed:
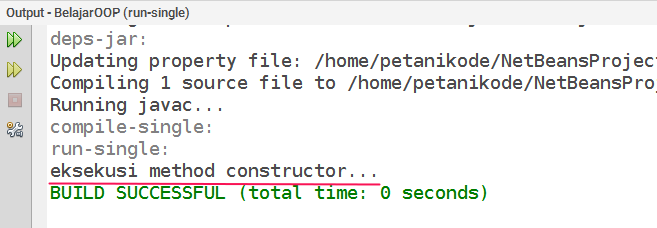
Parameter Constructor
A constructor is usually used to initialize data for classes.
To do this, we must make parameters as input for the constructor.
Example:
public class User {
public String username;
public String password;
public User(String username, String password){
this.username = username;
this.password = password;
}
}
In the class code
User
above, we add parameters username
and password
into the constructor.
Then later when we create an object, we must add a parameter value like this:
User petani = new User("petanikode", "kopi");
Sample complete code:
package konstruktor;
public class User {
public String username;
public String password;
public User(String username, String password){
this.username = username;
this.password = password;
}
}
class DemoConstructor{
public static void main(String[] args) {
User petani = new User("petanikode", "kopi");
System.out.println("Username: " + petani.username);
System.out.println("Password: " + petani.password);
}
}
The output results:
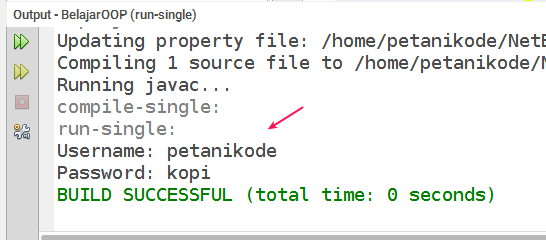
Destructor in Java
Destructor is a special method that will be executed when the object is deleted from memory.
Java itself does not have a destructor method, because Java uses a gerbage collector for memory management.
So the gerbage collector will automatically delete unused objects.
As for other programming languages, like C ++ we can make destructors themselves like this:
class User {
public:
User( String *username ); // <-- ini constructor
~User(); // <-- ini destructor.
private:
String username;
String password;
};
Sumber:
https://www.petanikode.com/java-oop-constructor
0 Komentar untuk "Learning Java OOP: Getting to know a constructor & destructor in Java"
Silahkan berkomentar sesuai artikel