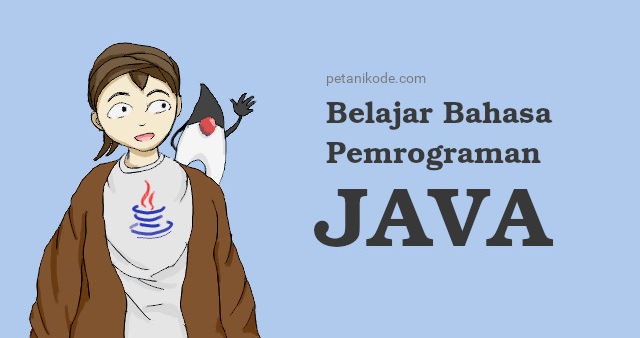
If you have previously learned about Array and ArrayList , I am sure you will easily digest this material.
On this occasion, we will learn about the HashMap. HashMap is often used in making Java applications. Therefore, HashMap is important for us to know.
Ok, just go ahead. Let's start…
What is a HashMap?
Class
HashMap
is a class derived from the class AbstractMap
and the implementation of the interface Map
.
HashMap is a class that contains a set of pairs of values (value)and key (key) .
Values can be in the form of strings, integers, booleans, floats, doubles, and objects. Whereas for keys usually in the form of strings and integers.
HashMap is like an associative array in Java.
Try to look at the following table:
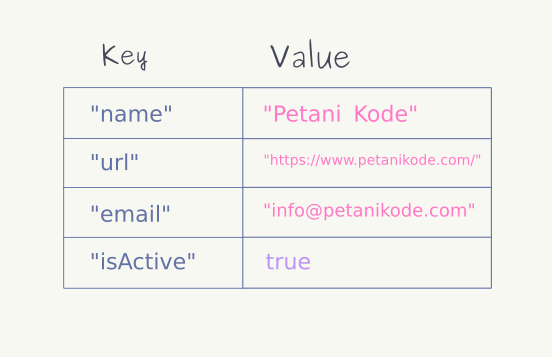
The table above consists of key and value pairs , like this is the contents of the class or HashMap object.
How to make a HashMap
Before you can use the HashMap, you have to import it first:
import java.util.HashMap;
After that we can use it.
Well, to use the HashMap, we must make the object first. Objects from Hashmap can be made with keywords
new
.
However, there is a little extra to determine the data type for the key and the value that will be stored.
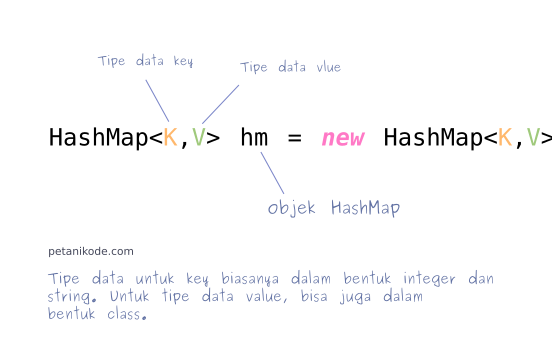
Example:
HashMap<Integer, String> days = new HashMap<Integer,String>
In the example above, we made the HashMap object named
days
. We can use this object to store data collections.
The data type used for the key is
Integer
and the value is String
.
Meaning : the key must be of type
Integer
and the stored value must be of type String.
For more details, please continue ...
Fill in the Value to the HashMap
Earlier we made a hashmap object named
days
with a data type:- K (key): Integer
- V (value): String
To fill values into objects
days
, we can use methods put
like this:days.put(1, "Minggu");
days.put(2, "Senin");
days.put(3, "Selasa");
days.put(4, "Rabu");
days.put(5, "Kamis");
days.put(6, "Jum'at");
days.put(7, "Sabtu");
Pay attention!
The HashMap object
days
contains the names of days with keys 1
- 7
. If you don't believe it, please try compiling an example of this program:import java.util.HashMap;
public class CobaHashMap {
public static void main(String[] args) {
// membuat objek hashmap
HashMap<Integer, String> days = new HashMap<Integer,String>();
// mengisi nilai ke objek days
days.put(1, "Minggu");
days.put(2, "Senin");
days.put(3, "Selasa");
days.put(4, "Rabu");
days.put(5, "Kamis");
days.put(6, "Jum'at");
days.put(7, "Sabtu");
// mencetak semua isi dari objek days
System.out.println("Isi objek days: " + days);
}
}
The result:
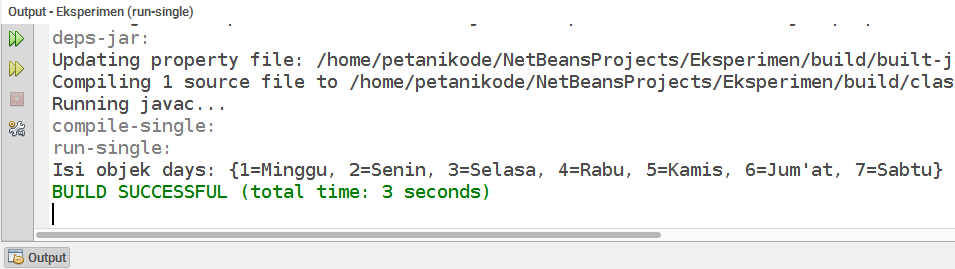
Retrieving Values from HashMap
To retrieve values from HashMap, we can use methods
get()
with key parameters.
Example:
// mengambil hari senin
days.get(2)
Pay attention!
Why do we fill in the parameters there
2
?
The answer is because we want to take Monday's value and that value has been associated with the HashMap object with the key
2
.
Complete code example:
import java.util.HashMap;
public class CobaHashMap {
public static void main(String[] args) {
// membuat objek hashmap
HashMap<Integer, String> days = new HashMap<Integer,String>();
// mengisi nilai ke objek days
days.put(1, "Minggu");
days.put(2, "Senin");
days.put(3, "Selasa");
days.put(4, "Rabu");
days.put(5, "Kamis");
days.put(6, "Jum'at");
days.put(7, "Sabtu");
// mencetak semua isi dari objek days
System.out.println("Isi objek days: " + days);
// mengambil hari senin
System.out.println("Hari kedua: " + days.get(2));
}
}
The result:
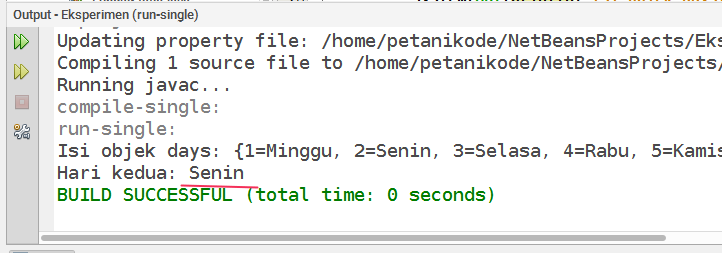
Try also to take other values, such as Wednesday, Thursday and Friday.
Deleting Value from HashMap
There are two methods that can be used to delete values from HashMap:
remove()
delete one value;clear()
delete all values;
Let's try:
import java.util.HashMap;
public class CobaHashMap {
public static void main(String[] args) {
// membuat objek hashmap
HashMap<Integer, String> days = new HashMap<Integer,String>();
// mengisi nilai ke objek days
days.put(1, "Minggu");
days.put(2, "Senin");
days.put(3, "Selasa");
days.put(4, "Rabu");
days.put(5, "Kamis");
days.put(6, "Jum'at");
days.put(7, "Sabtu");
// mencetak semua isi dari objek days
System.out.println("Isi objek days: " + days);
System.out.println("Hari kedua: " + days.get(2));
// menghapus malam minggu <-- jomblo detected :D
days.remove(1);
System.out.println("Isi objek days: " + days);
// menghapus semua hari <-- oh tidak kiamat donk!
days.clear();
System.out.println("Isi objek days: " + days);
}
}
The result:
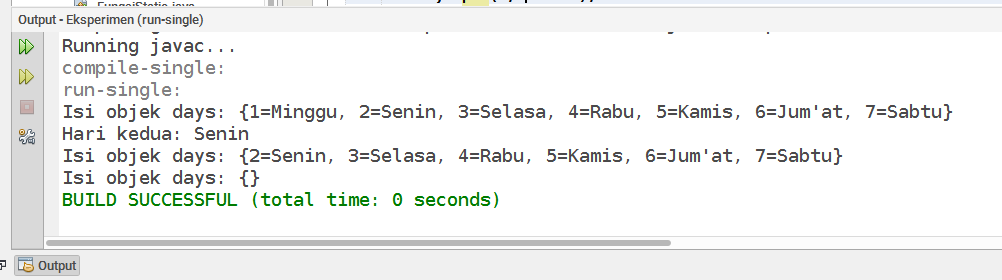
Changing Values and Keys from a HashMap
There are two methods that can be used to change values in a HashMap:
- Method
put()
- Method
replace()
What is the difference?
I don't think there is a difference. Both can be used to change values.
However, it looks like for the method
replace()
...
... the value to be changed must already be in the HashMap.
As for the method
put()
, he will add a new one if it isn't already in the HashMap.
Let's try:
import java.util.HashMap;
public class CobaHashMap {
public static void main(String[] args) {
// membuat objek hashmap
HashMap<Integer, String> days = new HashMap<Integer,String>();
// mengisi nilai ke objek days
days.put(1, "Minggu");
days.put(2, "Senin");
days.put(3, "Selasa");
days.put(4, "Rabu");
days.put(5, "Kamis");
days.put(6, "Jum'at");
days.put(7, "Sabtu");
// mengubah hari menggu menjadi hari ahad
days.put(1, "Ahad");
// mengubah hari rabu menjadi rabo
days.replace(4, "Rabo");
// mencetak semua isi dari objek days
System.out.println("Isi objek days: " + days);
}
}
The result:
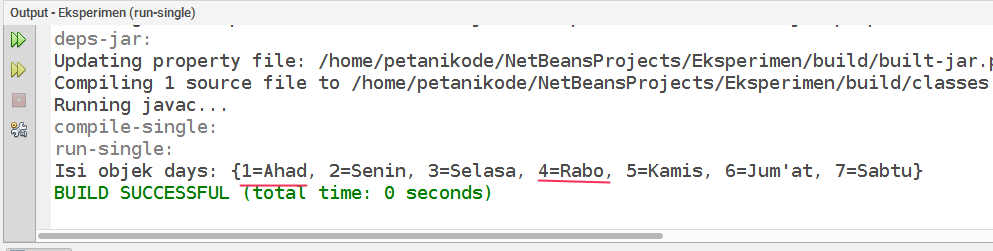
HashMap methods
The methods above are methods commonly used in the HashMap. Actually there are many other methods that we try.
To see it, please press the
Ctrl
+ button Spasi
on Netbeans when using the HashMap object.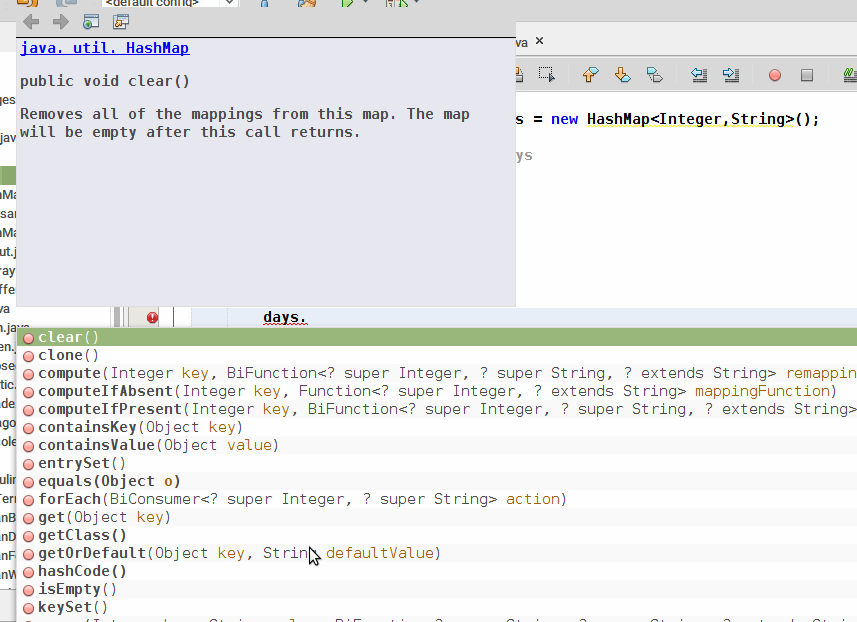
The following is an explanation of several methods:
clear()
to clean the contents of the HashMap;isEmpty()
to check whether the HashMap is empty or not;size()
to take the HashMap size (number of items in the hashmap);values()
to retrieve all values in the HashMap;keySet()
to retrieve all keys in the HashMap;clone()
to duplicate the HashMap object;- etc.
Bonus: Example of a program with a HashMap
This program consists of two classes, namely:
Buku.java
and BukuHashMap.java
. These two classes are in one package.
In this program, you will find an example of a HashMap that contains a set of objects.
Here is the contents of the class
Buku.java
:public class Buku {
private String title;
private String author;
public Buku(String title, String author) {
this.title = title;
this.author = author;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
}
Here is the contents of the class
BukuHashMap.java
:import java.util.HashMap;
import java.util.Map;
public class BukuHashMap {
public static void main(String[] args) {
// membuat objek hashmap
HashMap<String, Buku> books = new HashMap<String, Buku>();
// membuat objek buku
Buku bukuJava = new Buku("Tutorial Java", "Petani Kode");
Buku bukuKotlin = new Buku("Pemrograman Kotlin", "Petani Kode");
Buku bukuAndroid = new Buku("Pemrograman Android", "Petani Kode");
// mengisi objek hashmap dengan objek buku
books.put("java", bukuJava);
books.put("kotlin", bukuKotlin);
books.put("android", bukuAndroid);
// cetak semua buku
for(Map.Entry b: books.entrySet()){
Buku buku = (Buku) b.getValue();
System.out.println(b.getKey() + ": "+ buku.getTitle());
}
}
}
Please try compiling it yourself and understand the intent of the program code above.
The following are the outputs:
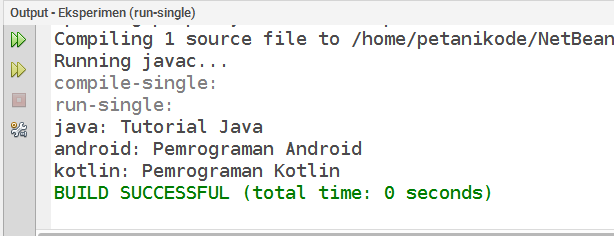
Sumber: https://www.petanikode.com/java-hashmap/
0 Komentar untuk "Learning Java: Knowing and Understanding HashMap Classes in Java"
Silahkan berkomentar sesuai artikel