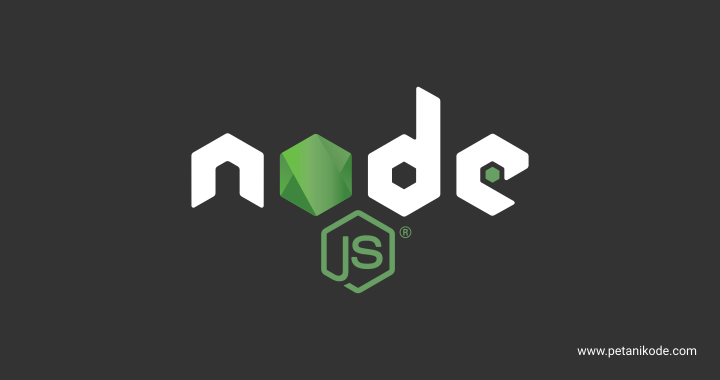
The disadvantages:
This webserver cannot receive data from the client .
For example:
We want to send data to the server via the form .
The question:
How do we retrieve data from the form ?
The body parser is a nodejs module that is used to retrieve data from the form in the Express framework.
However, in order to better understand how the form is processed in Nodejs ...
... we will not use the module.
Isya'allah, we will learn when we enter Expressjs.
Form Data Delivery Method
In general, there are two methods of sending data to the form:
- GET method;
- and the POST method.
We can define this method when creating a form:
<form method="GET" action="/send/">
...
</form>
What is the difference between the GET method and POST?
The GET method will send data through the URL, while the POST method will send data through the background.
The GET method is suitable for searching, because it sends data through a URL.
While the POST method, the match is used on the login form.
For more details.
Let's try ...
Retrieve data Using the GET Method
To retrieve data from the form method using the GET method, we can use the module
url
. Because we will retrieve data from URL parameters.
If you don't know about the module
url
, please read the explanation about the URL module in: "Learning Nodejs # 6: Using the URL Module to Create a Static Webserver"
Continue ...
Please create a new file named
form_get.js
, then fill in the following code:var http = require('http');
var url = require('url');
var fs = require('fs');
http.createServer(function (req, res) {
var q = url.parse(req.url, true);
if(q.pathname == "/search/" && req.method === "GET"){
// ambil parameter dari URL
var keyword = q.query.keyword;
if( keyword ){
// Ambil data dari form dengan metode GET
res.writeHead(200, {'Content-Type': 'text/html'});
res.write("<h3>Search Results:</h3>");
res.write("<p>Anda mencari: <b>" + keyword + "</b></p>");
res.write("<pre>Tidak ada hasil! Maaf website ini masih dalam pengembangan</pre>")
res.end("<a href='/search/'>Kembali</a>");
} else {
// tampilkan form search
fs.readFile('search.html', (err, data) => {
if (err) { // kirim balasan error
res.writeHead(404, {'Content-Type': 'text/html'});
return res.end("404 Not Found");
}
// kirim form search.html
res.writeHead(200, {'Content-Type': 'text/html'});
res.write(data);
return res.end();
});
}
}
}).listen(8000);
console.log('server is running on http://localhost:8000');
After that, create the file for the form with the name
search.html
and contents as follows:<!DOCTYPE html>
<html lang="en">
<head>
<title>Tutorial Form Nodejs</title>
</head>
<body>
<form action="/search/" method="GET">
<label>Cari: </label>
<input type="text" name="keyword" placeholder="kata kunci..." />
<input type="submit" value="Cari" />
</form>
</body>
</html>
Notice there is an input with the name
keyword
. This will be the name of the key in taking parameters.var keyword = q.query.keyword;
The result:
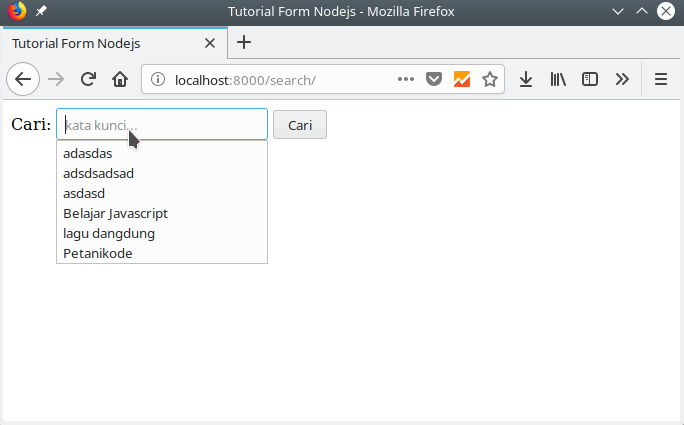
Retrieving data Using the POST Method
Whereas to retrieve data from the form using the POST method, we must use the module
querystring
.
What's the difference with the module
url
?
The module
url
functions for parsing URLs. There is indeed a function for parsing string queries . However, it queries the string from the URL.
By the way…
What is the query string ?
This is called the query string :
foo=bar&abc=xyz&abc=123
You often see it in the URL instead.
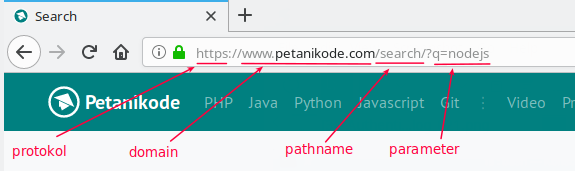
Sometimes query strings are also called parameters.
Well, on the form that uses the POST method ...
... actually it sent string queries too. But sent in background .
This is why we cannot use modules
url
.
The module is
querystring
already in Nodejs, so we don't need to install it with NPM (Node Package Manager) .
To be able to use the module
querystring
, we must import it first with the function require()
.var qs = require('querystring');
For more details, let's try ...
Make a new file named
form_post.js
with the contents as follows:var http = require('http');
var qs = require('querystring');
var fs = require('fs');
http.createServer(function (req, res) {
if(req.url === "/login/" && req.method === "GET"){
// tampilkan form login
fs.readFile("login_form.html", (err, data) => {
if (err) { // kirim balasan error
res.writeHead(404, {'Content-Type': 'text/html'});
return res.end("404 Not Found");
}
// kirim form login_form.html
res.writeHead(200, {'Content-Type': 'text/html'});
res.write(data);
return res.end();
});
}
if(req.url === "/login/" && req.method === "POST"){
// ambil data dari form dan proses
var requestBody = '';
req.on('data', function(data) {
// tangkap data dari form
requestBody += data;
// kirim balasan jika datanya terlalu besar
if(requestBody.length > 1e7) {
res.writeHead(413, 'Request Entity Too Large', {'Content-Type': 'text/html'});
res.end('<!doctype html><html><head><title>413</title></head><body>413: Request Entity Too Large</body></html>');
}
});
// kita sudah dapat datanya
// langkah berikutnya tinggal di-parse
req.on('end', function() {
var formData = qs.parse(requestBody);
// cek login
if( formData.username === "petanikode" && formData.password === "kopi"){
res.writeHead(200, {'Content-Type': 'text/html'});
res.write('<h2>Selamat datang bos!</h2> ');
res.write('<p>username: '+formData.username+'</p>');
res.write('<p>password: '+formData.password+'</p>');
res.write("<a href='/login/'>kembali</a>");
res.end();
} else {
res.writeHead(200, {'Content-Type': 'text/html'});
res.write('<h2>Login Gagal!</h2> ');
res.write("<a href='/login/'>coba lagi</a>");
res.end();
}
});
}
}).listen(8000);
console.log('server is running on http://localhost:8000');
After that, create an HTML file for the form with the name
login_form.html
and contents as follows:<!DOCTYPE html>
<html lang="en">
<head>
<title>Tutorial Form Nodejs</title>
</head>
<body>
<form action="/login/" method="POST">
<label for="username">Username: </label>
<input type="text" name="username" placeholder="username..." />
<br>
<label for="password">Password: </label>
<input type="password" name="password" placeholder="password..." />
<br>
<input type="submit" value="Login" />
</form>
</body>
</html>
The result:
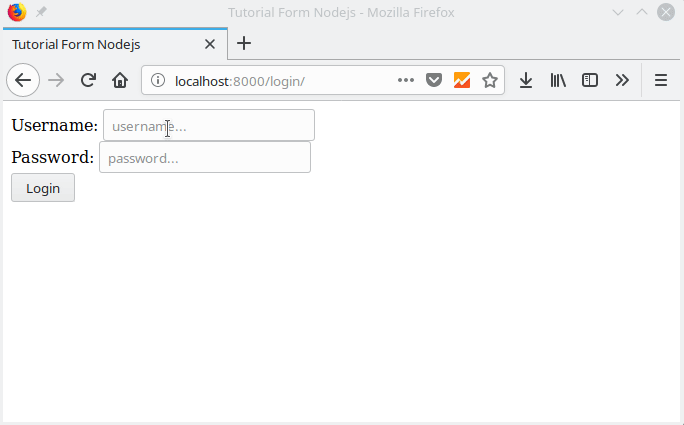
When I enter a username
dian
and password dian
, the login will fail.
... and when I enter my username
petanikode
and password kopi
, the login will succeed.
This is because we've bolted the logic in the program code.
Reference: https://www.petanikode.com/nodejs-form
0 Komentar untuk "Learning Nodejs # 7: How to retrieve data from forms?"
Silahkan berkomentar sesuai artikel