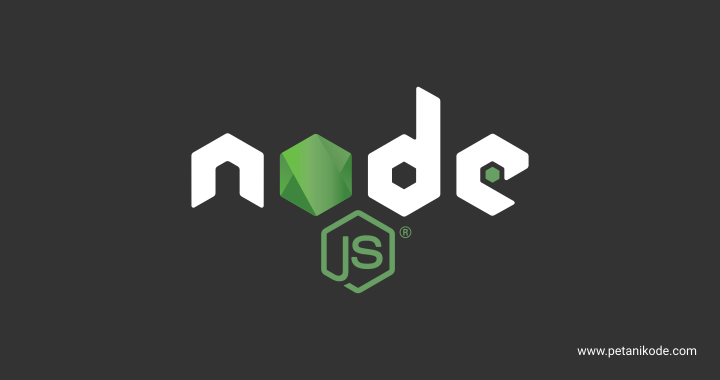
One of the factors that determine the quality of the application that we build is security .
In the previous tutorial:
We write the credential (configuration, username and password) directly in the program code.
Why?
Because it can open a security gap from our application.
One way to prevent this is to use Environmental Variables to store credentials .
What is an Environment Variable?
(Next, it will be easy, we will call it an env variable )
For example, a named env variable
HOME
will help the program find out the directory location home
of the user.
Variable environment is on every computer.
We can also make it ...
The env variable that we make, is usually temporary.
That is, it will only exist when the process occurs or the program is executed.
While the default env variable from the operating system will always be there.
Because it is created when the computer is turned on (boot / startup) .
In the Windows operation system, we can see Env Variables from My Computer-> Properties-> Advenced .
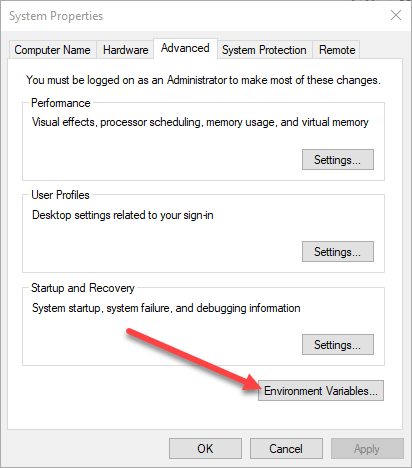
Then to create and view the contents of the Env variable from CMD, we can use the command
echo
and set
.
Example:
set SITE_NAME=Petani Kode
echo %SITE_NAME%
SITE_NAME
is the name of the variable. Then when we want to print the contents, we use the statement echo
with the variable name enclosed with the percent symbol ( %
).
This is a rule that applies to the Windows operating system.
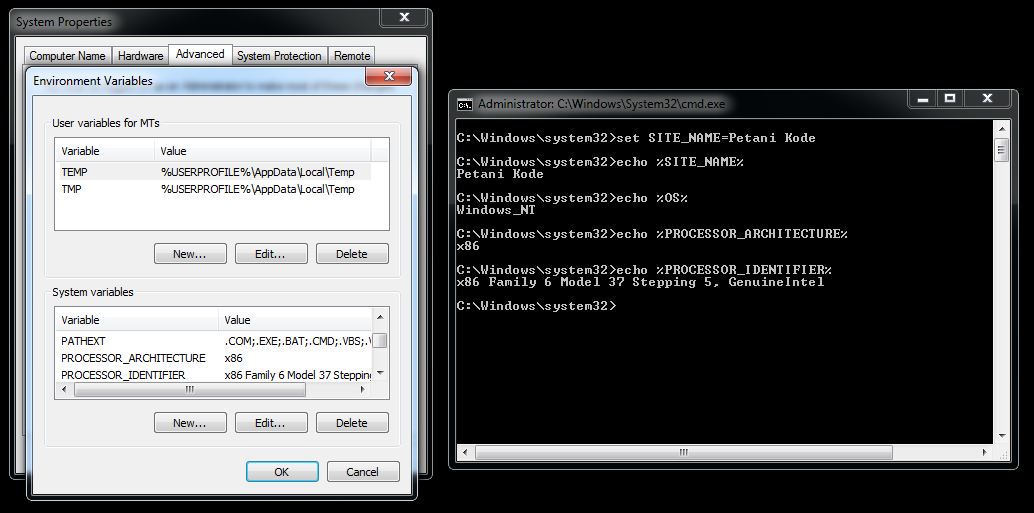
Then on Linux and Unix, how?
Not much different.
In Linux, we use commands
export
to create new variables and echo
to display them.export NAMA_VARIABEL="isi"
echo NAMA_VARIABEL
Example:
export SITE_NAME="Petani Kode"
echo $SITE_NAME
See! when we print a variable
SITE_NAME
, there we use the dollar symbol in front of it ( $
).
This is a rule that applies to the Linux and Unix operating systems.
Let's try to print the contents of variables that already exist, such as
USER
, HOME
, HOSTNAME
, etc.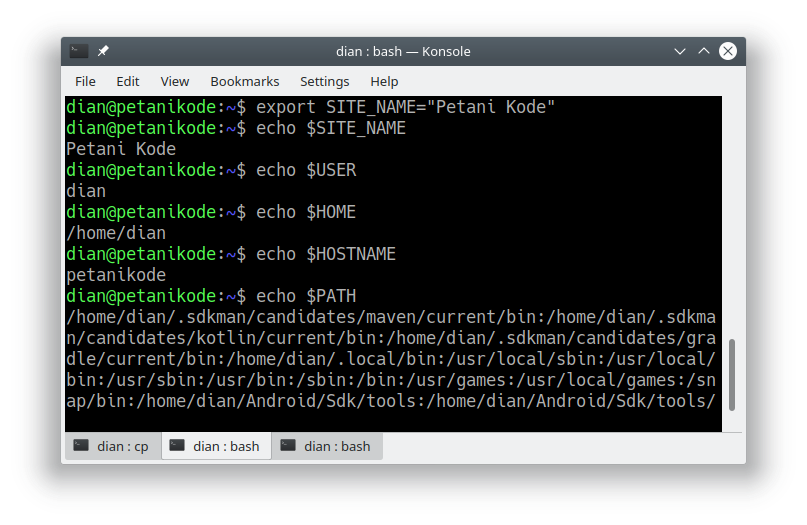
This is what is called an environment variable which we will use to store credentials later .
Accessing Env Variables from Nodejs
Variable environtment on Nodejs, can we access through the object
process
.
This is a global object in Nodejs that can be accessed from any program, without having to import it with a function
require()
.
The object
process
has a property env
that contains all environment variables on the computer.
For example, I want to access a variable
HOME
.
Then later what is written in the code will be like this:
var home = process.env.HOME;
This was actually used by us in making telegram bots at Hook.io and creating simsimi bots to store tokens.
Okay, so I understand better ...
Let's try with practice.
Create a new file with the name
baca_env.js
, then fill in the following code:var username = process.env.USERNAME;
var password = process.env.PASSWORD;
console.log("username anda: " + username);
console.log("password anda: " + password);
In the code above, we try to access variables
USERNAME
and PASSWORD
.
(This env variable does not yet exist on the computer)
Before executing the program, make the variable first with commands
export
(on Linux / Unix) or set
(on Windows).
If we do not create an env variable first, the result will
undefined
or the variable does not yet exist.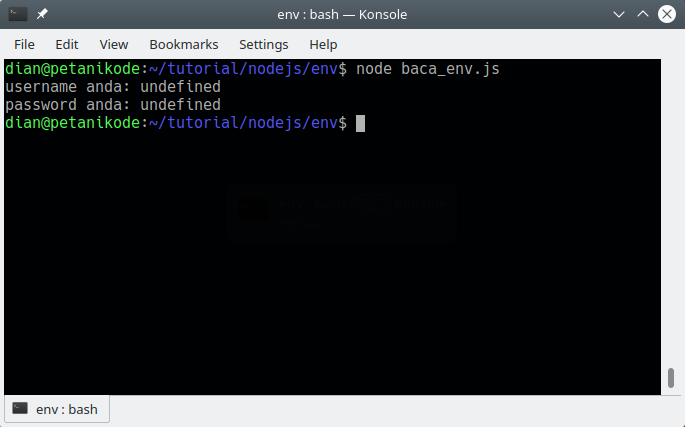
Creating env variables on Linux:
export USERNAME="Petanikode"
export PASSWORD="kopi"
Creating env variables on Windows:
set USERNAME=Petanikode
set PASSWORD=kopi
After creating the env variable, now try executing the program:
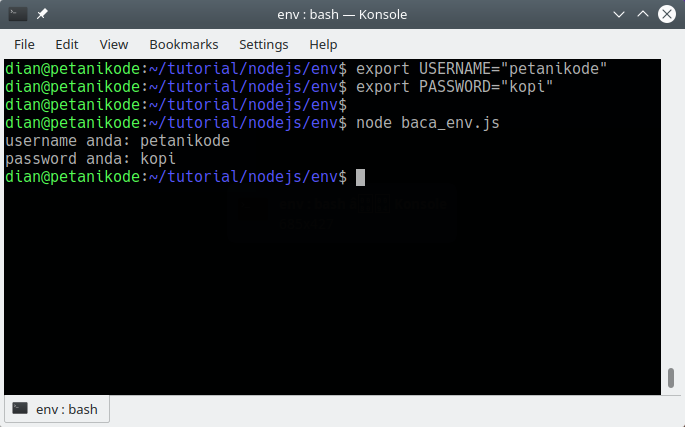
Do we always have to make an env variable during execution?
It doesn't have to be, just env variables are made once.
But, if later the Terminal or CMD is closed, then the variable will disappear.
Bro ... it's complicated,
every time we want to execute a program, we have to make the env variable first.
Not really.
Because we can:
Make Env Variable from Nodejs
Guess what?
Yep, we just have to create a new property in the object
process.env
.
Example:
create_env.js
// membaut variabel env
process.env.APP_VERSION = "1.34.2";
process.env.APP_NAME = "Tutorial Nodejs";
console.log("Variabel env sudah dibuat!");
console.log("APP_VERSION = " + process.env.APP_VERSION);
console.log("APP_NAME = " + process.env.APP_NAME);
In the program code above, we create two env variables with names
APP_VERSION
and APP_NAME
.
Can you use the lowercase variable name?
It's okay, but usually the env variable is made with all capital letters.
Just try to pay attention to the env variable that is already in the system.
USER
, HOME
, OS
, HOSTNAME
... et al.
They all use capital letters.
Continue ...
Now try the program execution
create_env.js
.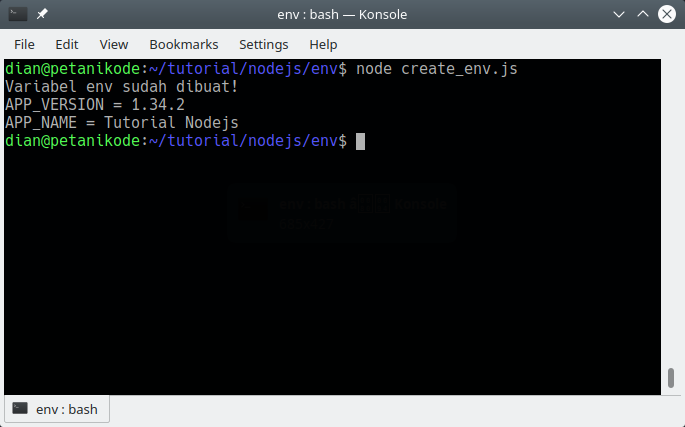
... and try using the command
echo
to print it: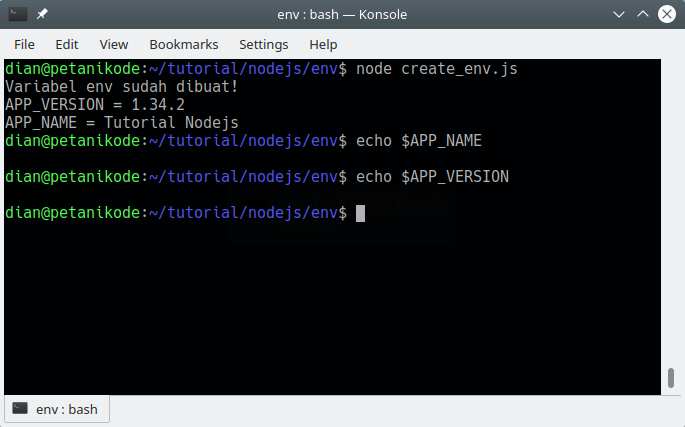
Lah! why not?
This is because the env variable has been deleted from memory.
Why can it be deleted?
Because that's how it is ...
The env variable will only exist when the program is executed.
Just try creating a new variable from Terminal or CMD, then close the Terminal / CMD and reopen it.
Then try the
echo
variable that was made earlier. Is there or not?
Definitely not.
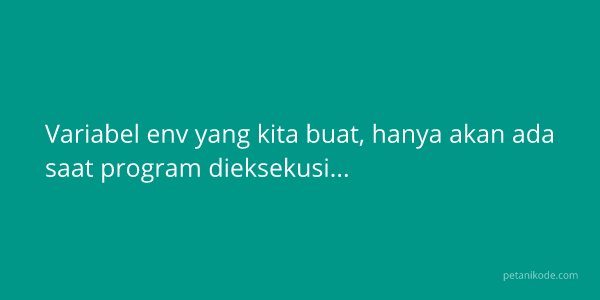
And what about the variables
USER
, HOSTNAME
, HOME
, et al.?
Why are they always there?
Because they are made on startup computers or when Terminal / CMD is opened.
Usually, - on Linux - we create an env variable in the file
.bashrc
so that the variable is always in the Terminal.
... or it could be at
/etc/bashrc/
and /etc/profile
.
Meaning later ... if we open the application on another computer, the computer must be made again without the env label.
Yes, it is true.
When we work with teams, applications will also be opened on other computers.
We have to make the env variable there again.
Sounds complicated ...
But take it easy, this problem can be solved by:
Using Files .env
File
.env
(dotenv) functions to store env variables.
This file contains the declaration or creation of an env variable which we can load from Nodejs.
In order to use files
.env
, we need a module dotenv
.
This module will later help us to load all variables in the file
.env
.
Let's try ...
First, we first install the module
dotenv
into the project with the command:npm install dotenv
Wait until the process is complete.
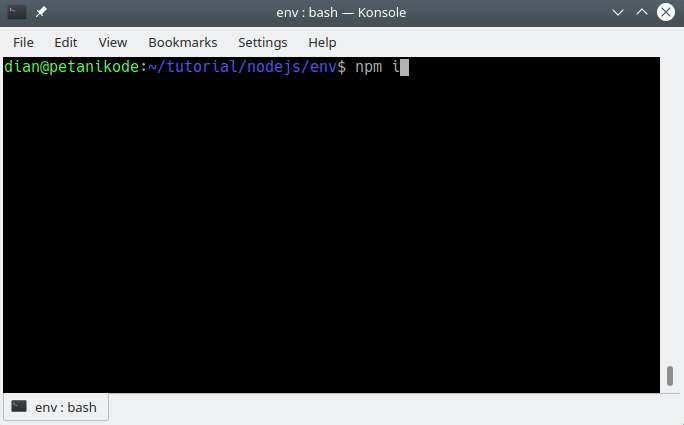
After that, create a new file with the name
dotenv.js
as follows:// load semua variabel env dari file .env
require('dotenv').config();
// mencetak variabel env
console.log("HOST: " + process.env.DB_HOST);
console.log("USER: " + process.env.DB_USER);
console.log("PASS: " + process.env.DB_PASS);
console.log("NAME: " + process.env.DB_NAME);
Then, create a file
.env
with the contents as follows:DB_HOST=localhost
DB_USER=dian
DB_PASS=petanikode
DB_NAME=blog_nodejs
After that, try executing the program
dotenv.js
.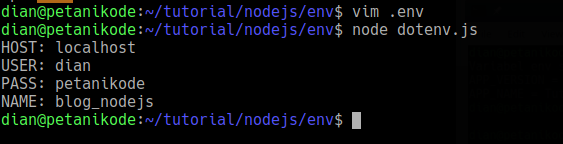
Steady 
When this line is executed, all variables in the file
.env
will be loaded into the program or memory.require('dotenv').config();
The method
config()
can actually be given parameters to specify files .env
specifically.
Example:
require('dotenv').config({path: '/full/custom/path/to/your/env/vars'})
For other parameters, you can read it in the documentation. 3
Anyway, if you use Git , make sure to enter the file
.env
inside .gitignore
so that it doesn't get recorded by Git.
When we will host or deploy applications to the server ...
... files
.env
do not need to be uploaded.
Later we create another env variable on the server.
How to?
The method depends on the hosting server used.
Reference: https://www.petanikode.com/nodejs-env/
0 Komentar untuk "Learning Nodejs # 11: Use Environment Variables to Secure Your Apps"
Silahkan berkomentar sesuai artikel