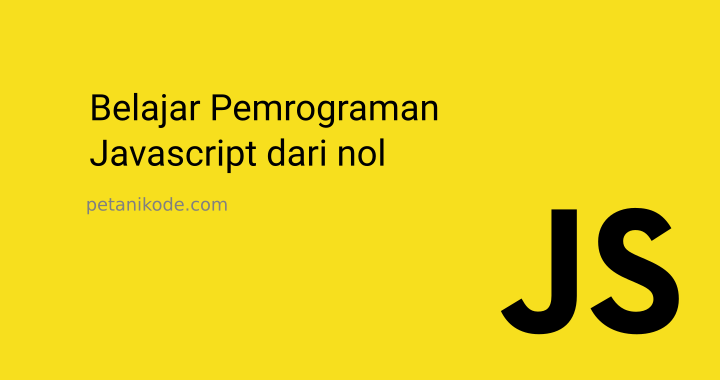
In real life, we often encounter objects. Object is everything that exists in this world.
Whether it's inanimate objects or living things. All objects.
We can model these objects in programming.
Usually using the OOP (Object Oriented Programming) paradigm or object oriented programming.
Now this OOP paradigm is a technique or method in programming where everything is viewed as an object.
These objects can interact so that they form a program.
What are Objects in Javascript and How do I make them?
The object is actually a variable that stores the value (property) and function (method) .
Examples of car objects: 1
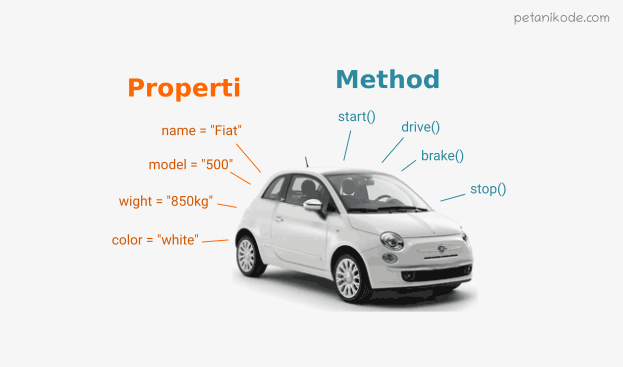
How do we model this car in the program code?
It can be like this:
var car = "Mobil Fiat";
But variables
car
will only store the name of the car. Therefore, we must use objects.
Objects in javascript, can be made with curly brackets with contents in the form of keys and values .
Example:
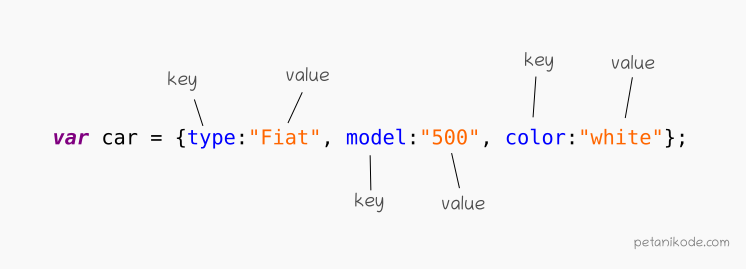
The code above can also be written like this:
var car = {
type:"Fiat",
model:"500",
color:"white"
};
What are Property Differences and Methods?
In the example above, we only make property.
Property is a characteristic of an object (variable). While the method is the behavior of the object (function).
Then how do you make metohd in the object?
Methods can be created by filling in a value with a function.
Example:
var car = {
// properti
type: "Fiat",
model: "500",
color: "white",
// method
start: function(){
console.log("ini method start");
},
drive: function(){
console.log("ini method drive");
},
brake: function(){
console.log("ini method brake");
},
stop: function(){
console.log("ini method stop");
}
};
How to Access Properties and Object Methods
We already know how to make objects ...
Then the question:
How do I access properties and methods of an object?
The method is using a dot or dot (
.
), then followed by the name of the property or method.
Example:
console.log(car.type);
console.log(car.color);
car.start();
car.drive();
note
car.type
, car.color
, car.start()
, and car.drive()
!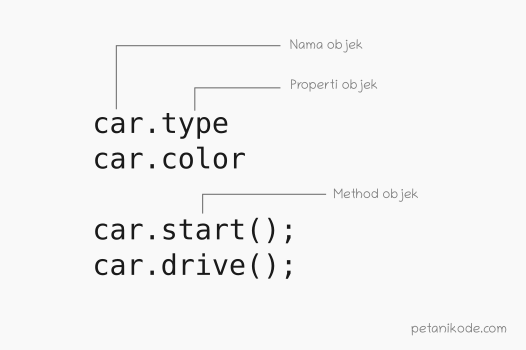
To access properties, we just use the name
objek.properti
. As for the method, we must use parentheses. This states if we want to execute the function.
Using Keyword this
Keywords are
this
used to access properties and methods from within the method.
Example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Belajar Objek Javascript</title>
<script>
var person = {
firstName: "Muhar",
lastName: "Dian",
showName: function(){
alert(`Nama: ${this.firstName} ${this.lastName}`);
}
};
person.showName();
</script>
</head>
<body>
The result:
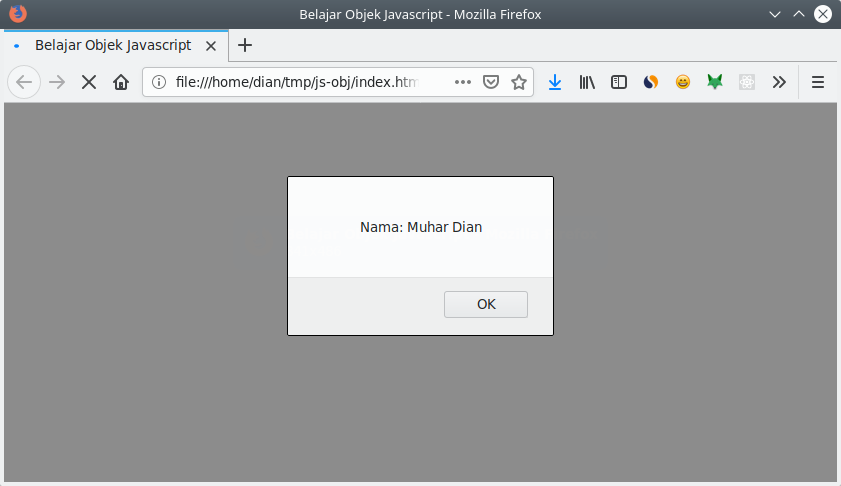
The keyword
this
in the example above will refer to the object person
.
Create an Object Class with this
In object oriented programming, we usually create objects by creating instances of classes .
But in the examples above, we make the object directly.
Then what if we want to create another object with the same property.
You can make two like this:
var person = {
firstName: "Muhar",
lastName: "Dian",
showName: function(){
alert(`Nama: ${this.firstName} ${this.lastName}`);
}
};
var person2 = {
firstName: "Petani",
lastName: "Kode",
showName: function(){
alert(`Nama: ${this.firstName} ${this.lastName}`);
}
};
This is certainly not effective, if we want to make many objects. Because we have to rewrite the same code.
The solution we can use
class
.
Oh yeah, I want to let you know:
In the ES5 version of JavaScript, it
class
doesn't exist yet. This feature has just been added to the ES6 version of JavaScript.
In ES5, we can create classes with functions. Then in it use the keyword this.
Example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Belajar Objek Javascript</title>
<script>
function Person(firstName, lastName){
// properti
this.firstName = firstName;
this.lastName = lastName;
// method
this.fullName = function(){
return `${this.firstName} ${this.lastName}`
}
this.showName = function(){
document.write(this.fullName());
}
}
var person1 = new Person("Muhar", "Dian");
var person2 = new Person("Petani", "Kode");
person1.showName();
document.write("<br>");
person2.showName();
</script>
</head>
<body>
The result:
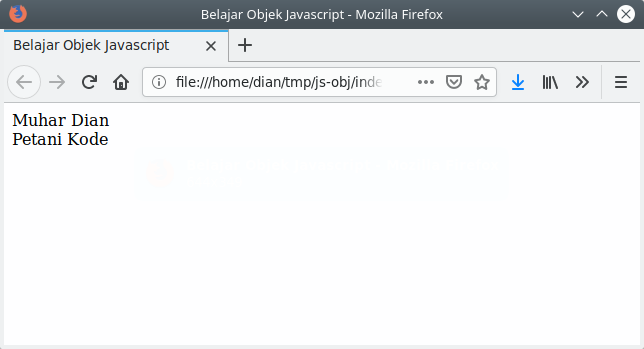
Consider the example above!
We create new objects with keywords
new
, then give the parameter values firstName
and lastName
.var person1 = new Person("Muhar", "Dian");
So, whatever object we want to make ...
... just use keywords
new
.
Reference: https://www.petanikode.com/javascript-objek/
0 Komentar untuk "Learning Javascript: What are Objects? and How to Make It?"
Silahkan berkomentar sesuai artikel