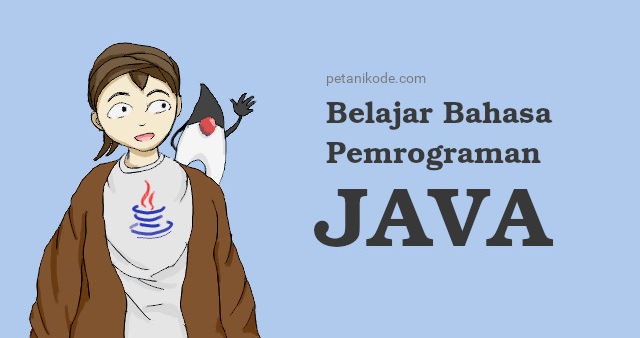
Each programming language has different structure and rules for writing syntax.
Java is a programming language developed from the C language and certainly will follow the writing style of C.
When you first see a Java program, maybe you will ask questions.
What is this? What is that?
Example:
Consider the following program:
package com.petanikode.program;
class Program {
public static void main(String args[]){
System.out.println("Hello World");
}
}
Many things we don't know yet.
What is it
package
?
What is it
class
?
... and why is it written like that?
Therefore, we need to learn the basic syntax and structure of Java programs.
Let's start…
Basic Structure of Java Programs
The structure of the Java program is generally divided into 4 parts:
- Package Declaration
- Import Library
- Class Section
- Main Method
Let's look at an example:
package com.petanikode.program; //<- 1. deklarasi package
import java.io.File; //<- 2. Impor library
class Program { //<- 3. Bagian class
public static void main(String args[]){ //<- 4. Method main
System.out.println("Hello World");
}
}
Let's discuss them one by one…
1. Declaration of Package
Package is a folder that contains a set of Java programs.
Package declarations are usually done when creating a large program or application.
Example package declaration:
package com.petanikode.program;
Usually the package name follows the domain name from a vendor who issued the program.
In the example above, it
com.petanikode
is the domain name of the code farmer.
The rule: the domain name is reversed, then followed by the name of the program.
What if we don't declare a package ?
It's okay and the program will still work.
We must declare the package .
2. Import Section
In this section, we import the library needed for the program.
Library is a set of classes and functions that we can use in creating programs.
Examples of library imports:
import java.util.Scanner;
In this example, we import the class
Scanner
from the package java.util
.3. Class Section
Java is a programming language that uses the OOP (Object Oriented Programming) paradigm .
Each program must be wrapped in a class so that later it can be made into an object.
If you don't understand what OOP is?
Simply understand the class as the program name declaration.
class NamaProgram {
public static void main(String args[]){
System.out.println("Hello World");
}
}
This is a class block.
The class block opens with curly brackets
{
then closes or ends with }
.
In the class block, we can fill it with methods or functions and also variables.
In the example above, there is a method
main()
.4. Play Method
Method
main()
or function main()
is a program block that will be executed first.
This is the program entry point.
The method
main()
we must make. If not, the program will not be executed.
Example method
main()
.public static void main(String args[]){
System.out.println("Hello World");
}
Writing must be like this ...
Method
main()
has parameters args[]
. This parameter will then store a value from the arguments in the command line .
Then in the method
main()
, there is a statement or function:System.out.println("Hello World");
This is a function to display text to the monitor screen.
Statement and Expression in Java
Statements and expressions are the smallest part of the program. Every statement and expression in Java must end with a semicolon (
;
).
Example statement and expression:
System.out.println("Hello World");
System.out.println("Apa kabar?");
var x = 3;
var y = 8;
var z = x + y;
Statements and expressions will be instructions that will be done by the computer.
In the example above, we tell the computer to display text
"Hello World"
, and "Apa kabar?"
.
Then we tell him to calculate the value
x + y
.Java Program Blocks
The program block is a collection of statements and expressions wrapped in one.
The program block is always opened with curly braces
{
and closed with }
.
Example block program:
// blok program main
public static void main(String args[]){
System.out.println("Hello World");
System.out.println("Hello Kode");
// blok program if
if( true ){
System.out.println('True');
}
// blok program for
for ( int i = 0; i<10; i++){
System.out.println("Perulangan ke"+i);
}
}
The bottom line: if you find parentheses
{
and }
, then that is a program block.
The program block can also contain other program blocks (nested) .
In the example above, the program
main()
block contains an ifand for block .
We will study this block at:
Writing Comments on Java
Comments are part of a program that will not be executed by the computer.
Comments are usually used for:
- Give information to the program code;
- Disabling certain functions;
- Making documentation;
- etc.
Writing comments in java, same as in C language. Namely using:
- Double slash (
//
) for single line comments; - Star slash (
/*...*/
) for comments that are more than one line.
Example:
public static void main(String args[]){
// ini adalah komentar satu baris
System.out.println("Hello World");
// komentar akan diabaikan oleh komputer
// berikut ini fungsi yang di-non-aktifkan dengan komentar
// System.out.println("Hello World");
/*
Ini adalah penulisan komentar
yang lebih dari
satu baris
*/
}
String and Character Writing
String is a collection of characters. We often know it with text.
Example string:
"Hello world"
The rules for writing strings in Java, must be enclosed in double quotes as in the example above.
If flanked by single quotes, it will become a character.
Example:
'Hello world'
.
So please distinguish:
- Double quotation marks (
"..."
) for making strings; - While single quotes (
'...'
) to make characters.
Case Sensitive
Java is Case Sensitive , meaning uppercase or capital and lowercase letters are distinguished.
Example:
String nama = "Petani Kode";
String Nama = "petanikode";
String NAMA = "Petanikode.com";
System.out.println(nama);
System.out.println(Nama);
System.out.println(NAMA);
The three variables are three different variables, although they are both named
nama
.
Many beginners are often wrong about this. Because it can't tell which variables use uppercase letters and which ones use lowercase letters.
If we create a variable like this:
String jenisKelamin = "Laki-laki";
Then we have to call it like this:
System.out.println(jenisKelamin);
Not like this:
System.out.println(jeniskelamin);
Note, letters
K
are uppercase letters.Style of Case Writing
The case style used by Java is: camelCase , PascalCase , and ALL UPPER .
The camelCase writing style is used for variable names, object names, and method names.
Example:
String namaSaya = "Dian";
Then for PascalCase it is used for writing class names.
Example:
class HelloWOrld {
//...
}
Notice the class name, we use capital letters at the beginning, and capital letters in letters
W
to separate the two syllables.
Whereas the front letter camelCase uses lowercase letters, and the next syllable prefix uses uppercase letters.
// ini camelCase
belajarJava
// ini PascalCase
BelajarJava
Then, writing ALL UPPER or as desired capital is used to create constant names.
Example:
public final String DB_NAME = "petanikode";
To write two syllables or more, ALL UPPER is separated by the bottom line or underscore (
_
).
May I write carelessly?
For example for the class name using ALL UPPER ?
It's okay, the program will not error. But the program code that you write will look dirty and out of the grid that has been set.
0 Komentar untuk "Learning Java: Understanding the Structure and Rules of Writing Java Syntax"
Silahkan berkomentar sesuai artikel