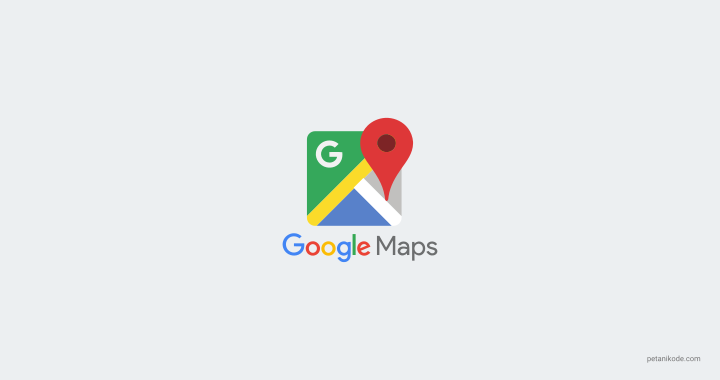
Markers are often used to mark a location. Usually it is often used in creating Geolocation applications.
On this occasion, we will learn to make markers on Google Maps and make some modifications.
Ready?
Let's start…
Know the Marker Object
A marker is an object that we can create with the following code:
var marker = new google.maps.Marker({
position: new google.maps.LatLng(-8.5830695,116.3202515),
map: peta
});
There are two important properties that must be given to the marker :
position
is the position of latitude and longitude marker coordinates on the map.map
object from a map (Google Map).
Example:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Tutorial Google Map - Petani Kode</title>
<script src="http://maps.googleapis.com/maps/api/js"></script>
<script>
function initialize() {
var propertiPeta = {
center:new google.maps.LatLng(-8.5830695,116.3202515),
zoom:9,
mapTypeId:google.maps.MapTypeId.ROADMAP
};
var peta = new google.maps.Map(document.getElementById("googleMap"), propertiPeta);
// membuat Marker
var marker=new google.maps.Marker({
position: new google.maps.LatLng(-8.5830695,116.3202515),
map: peta
});
}
// event jendela di-load
google.maps.event.addDomListener(window, 'load', initialize);
</script>
</head>
<body>
<div id="googleMap" style="width:100%;height:380px;"></div>
</body>
</html>
The result:
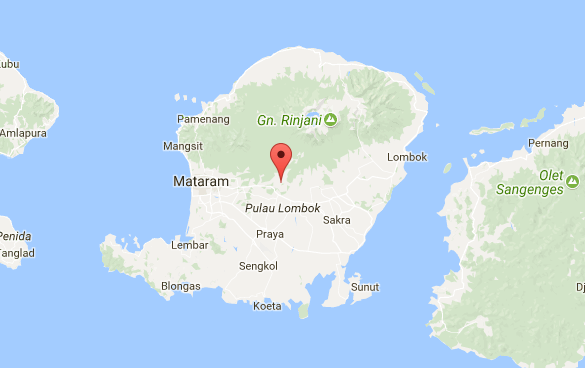
Live Demo: http://jsbin.com/raluzez/7/embed?output
Marker Animation
Apart from the two mandatory properties, there are also optional properties such as
animation
for animating markers .
Example:
var marker=new google.maps.Marker({
position: new google.maps.LatLng(-8.5830695,116.3202515),
map: peta,
animation: google.maps.Animation.BOUNCE
});
We give value
google.maps.Animation.BOUNCE
to property animation
. That is, the animation we want is in the form of a bounce (bounce) .
Sample complete code:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Tutorial Google Map - Petani Kode</title>
<script src="http://maps.googleapis.com/maps/api/js"></script>
<script>
function initialize() {
var propertiPeta = {
center:new google.maps.LatLng(-8.5830695,116.3202515),
zoom:9,
mapTypeId:google.maps.MapTypeId.ROADMAP
};
var peta = new google.maps.Map(document.getElementById("googleMap"), propertiPeta);
// membuat Marker
var marker=new google.maps.Marker({
position: new google.maps.LatLng(-8.5830695,116.3202515),
map: peta,
animation: google.maps.Animation.BOUNCE
});
}
// event jendela di-load
google.maps.event.addDomListener(window, 'load', initialize);
</script>
</head>
<body>
<div id="googleMap" style="width:100%;height:380px;"></div>
</body>
</html>
The result:
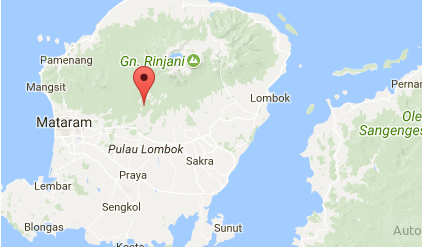
Live demo: http://jsbin.com/raluzez/8/embed
Besides animation
BOUNCE
there is also DROP
( google.maps.Animation.DROP
).Custom Icons
If we don't want to use the default icon from the red marker . we can make custom icons by ourselves with properties
icon
.
The value of the property
icon
can be a URL of the icon image that will be used.
Format icons should not be
.ico
, such as image format jpg
, png
and gif
are also accepted.
Example:
var marker=new google.maps.Marker({
position: new google.maps.LatLng(-8.623592, 116.222123),
map: peta,
icon: "https://developers.google.com/maps/documentation/javascript/examples/full/images/beachflag.png"
});
Complete example:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Tutorial Google Map - Petani Kode</title>
<script src="http://maps.googleapis.com/maps/api/js"></script>
<script>
function initialize() {
var propertiPeta = {
center:new google.maps.LatLng(-8.5830695,116.3202515),
zoom:9,
mapTypeId:google.maps.MapTypeId.ROADMAP
};
var peta = new google.maps.Map(document.getElementById("googleMap"), propertiPeta);
// membuat Marker
var marker=new google.maps.Marker({
position: new google.maps.LatLng(-8.623592, 116.222123),
map: peta,
icon: "https://developers.google.com/maps/documentation/javascript/examples/full/images/beachflag.png"
});
}
// event jendela di-load
google.maps.event.addDomListener(window, 'load', initialize);
</script>
</head>
<body>
<div id="googleMap" style="width:100%;height:380px;"></div>
</body>
</html>
The result:
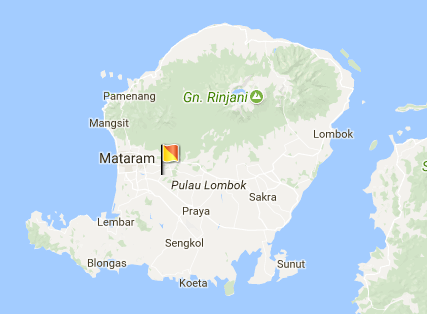
Live demo: http://jsbin.com/menoyixine/1/edit?output
Make sure to use the
32x32
pixel icon size to make it look good.Make a Marker When Map is clicked
What if we want to make a marker when the map is clicked?
This can be done by adding the event listerner, click on the map. Then call the function to make a marker .
Example function for creating markers :
function taruhMarker(peta, posisiTitik){
// membuat Marker
var marker = new google.maps.Marker({
position: posisiTitik,
map: peta
});
}
Then we call the function when the map event is clicked:
google.maps.event.addListener(peta, 'click', function(event) {
taruhMarker(this, event.latLng);
});
The function
taruhMarker()
requires two parameters, namely: peta
and posisiTitik
to be able to make markers .
In the example above, we give
this
for the object peta
and event.latLng
for the position of the marker .
Why use it
this
?
Because the function is called in the map event and
this
already represents the object from the map.
Sample complete code:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Tutorial Google Map - Petani Kode</title>
<script src="http://maps.googleapis.com/maps/api/js"></script>
<script>
function taruhMarker(peta, posisiTitik){
// membuat Marker
var marker = new google.maps.Marker({
position: posisiTitik,
map: peta
});
}
function initialize() {
var propertiPeta = {
center:new google.maps.LatLng(-8.5830695,116.3202515),
zoom:9,
mapTypeId:google.maps.MapTypeId.ROADMAP
};
var peta = new google.maps.Map(document.getElementById("googleMap"), propertiPeta);
// even listner ketika peta diklik
google.maps.event.addListener(peta, 'click', function(event) {
taruhMarker(this, event.latLng);
});
}
// event jendela di-load
google.maps.event.addDomListener(window, 'load', initialize);
</script>
</head>
<body>
<div id="googleMap" style="width:100%;height:380px;"></div>
</body>
</html>
The result:
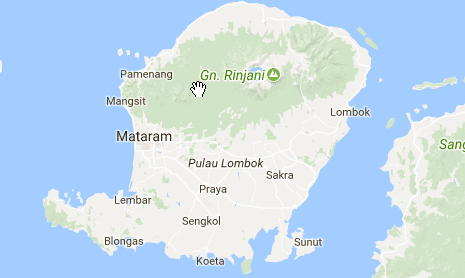
Live demo: http://jsbin.com/raluzez/12/embed?html , output
Make Only One Marker
What if we want to make only one marker when the map is clicked?
We must modify the function
taruhMarker()
like this:var marker;
function taruhMarker(peta, posisiTitik){
if( marker ){
// pindahkan marker
marker.setPosition(posisiTitik);
} else {
// buat marker baru
marker = new google.maps.Marker({
position: posisiTitik,
map: peta
});
}
}
marker
We put objects or variables outside the function to become global variables.
Then in the function
taruhMarker()
we check with IF / ELSE.if(marker){
//...
} else {
//...
}
If there are markers on the map, just move the position. But if it's not there, then make a new one.
To move the position of the marker , we can simply call the method
setPosition()
with the new coordinate parameter:marker.setPosition(posisiTitik);
Sample complete code:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Tutorial Google Map - Petani Kode</title>
<script src="http://maps.googleapis.com/maps/api/js"></script>
<script>
// variabel global marker
var marker;
function taruhMarker(peta, posisiTitik){
if( marker ){
// pindahkan marker
marker.setPosition(posisiTitik);
} else {
// buat marker baru
marker = new google.maps.Marker({
position: posisiTitik,
map: peta
});
}
}
function initialize() {
var propertiPeta = {
center:new google.maps.LatLng(-8.5830695,116.3202515),
zoom:9,
mapTypeId:google.maps.MapTypeId.ROADMAP
};
var peta = new google.maps.Map(document.getElementById("googleMap"), propertiPeta);
// even listner ketika peta diklik
google.maps.event.addListener(peta, 'click', function(event) {
taruhMarker(this, event.latLng);
});
}
// event jendela di-load
google.maps.event.addDomListener(window, 'load', initialize);
</script>
</head>
<body>
<div id="googleMap" style="width:100%;height:380px;"></div>
</body>
</html>
The result:
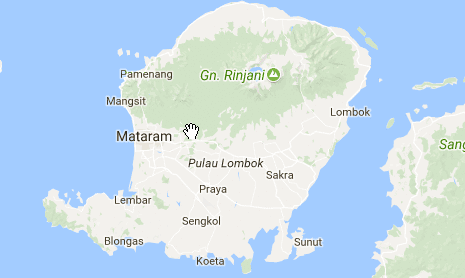
Live demo: http://jsbin.com/raluzez/14/embed?html , output
How to take coordinate points from markers ?
Good question.
Taking the coordinates of the markers can be needed when creating the input form. Then the coordinate value is sent to the database.
We can take the coordinates of the marker from the function
taruhMarker()
or when the map event is clicked.
Example:
function taruhMarker(peta, posisiTitik){
if( marker ){
// pindahkan marker
marker.setPosition(posisiTitik);
} else {
// buat marker baru
marker = new google.maps.Marker({
position: posisiTitik,
map: peta
});
}
console.log("Posisi marker: " + posisiTitik);
}
The code in ata will display the position coordinates from the marker to the console .
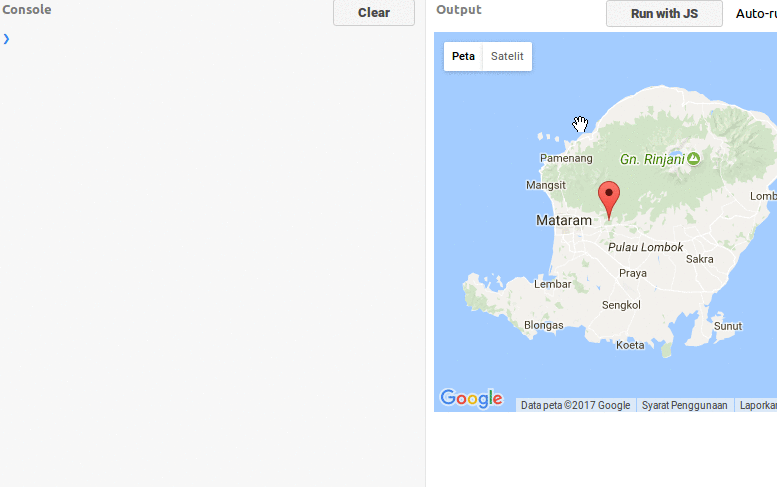
Then how do we put the values of these coordinates in the form?
We can use the DOM to send the value to from.
The sample code form:
<form action="" method="post">
<input type="text" id="lat" name="lat" value="">
<input type="text" id="lng" name="lng" value="">
</form>
Then in the function
taruhMarker()
we assign values to that element.function taruhMarker(peta, posisiTitik){
if( marker ){
// pindahkan marker
marker.setPosition(posisiTitik);
} else {
// buat marker baru
marker = new google.maps.Marker({
position: posisiTitik,
map: peta
});
}
// isi nilai koordinat ke form
document.getElementById("lat").value = posisiTitik.lat();
document.getElementById("lng").value = posisiTitik.lng();
}
Complete code:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Tutorial Google Map - Petani Kode</title>
<script src="http://maps.googleapis.com/maps/api/js"></script>
<script>
// variabel global marker
var marker;
function taruhMarker(peta, posisiTitik){
if( marker ){
// pindahkan marker
marker.setPosition(posisiTitik);
} else {
// buat marker baru
marker = new google.maps.Marker({
position: posisiTitik,
map: peta
});
}
// isi nilai koordinat ke form
document.getElementById("lat").value = posisiTitik.lat();
document.getElementById("lng").value = posisiTitik.lng();
}
function initialize() {
var propertiPeta = {
center:new google.maps.LatLng(-8.5830695,116.3202515),
zoom:9,
mapTypeId:google.maps.MapTypeId.ROADMAP
};
var peta = new google.maps.Map(document.getElementById("googleMap"), propertiPeta);
// even listner ketika peta diklik
google.maps.event.addListener(peta, 'click', function(event) {
taruhMarker(this, event.latLng);
});
}
// event jendela di-load
google.maps.event.addDomListener(window, 'load', initialize);
</script>
</head>
<body>
<div id="googleMap" style="width:100%;height:380px;"></div>
<form action="" method="post">
<input type="text" id="lat" name="lat" value="">
<input type="text" id="lng" name="lng" value="">
</form>
</body>
</html>
The result:
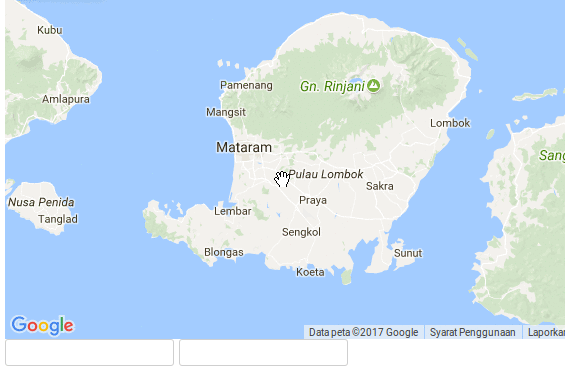
Live demo: http://jsbin.com/wevahox/3/edit?output
Now if the coordinate value is in the form, we just have to send it to the database.
Besides using input type = "text" , we can also use hidden .
<form action="" method="post">
<input type="hidden" id="lat" name="lat" value="">
<input type="hidden" id="lng" name="lng" value="">
</form>
0 Komentar untuk "Google Maps API Tutorial: Creating Markers to Mark Locations"
Silahkan berkomentar sesuai artikel