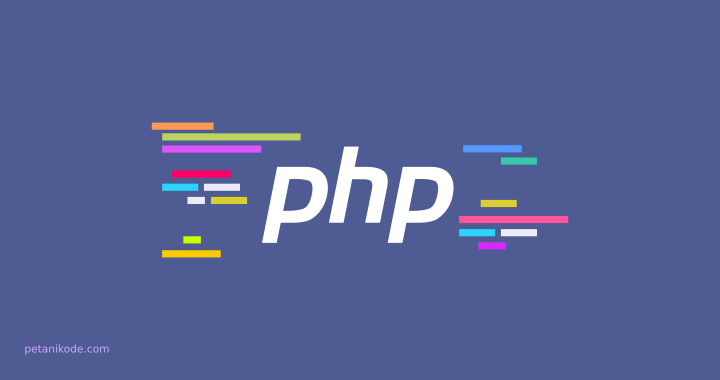
Imagine now that we are making a web application, then want to display a list of product names.
We can make it like this:
<?php
$produk1 = "Modem";
$produk2 = "Hardisk";
$produk3 = "Flashdisk";
echo "$produk1<br>";
echo "$produk2<br>";
echo "$produk3<br>";
Can it be like this?
It is allowed. But it is less effective.
Why?
What if there were 100 products, would we make 100 variables and do
echo
100x?
I'm tired.
Therefore, we must use Arrays.
On this occasion, we will discuss:
- What is an Array?
- How to make an array in PHP and fill it
- How to display Array values
- How to delete the contents of an array
- How to Add Array Contents
- Associative Array
- Multidimensional Arrays
Let's start ....
1. What is an Array?
An array is a data structure that contains a set of data and has an index. Index is used to access array values.
The array index always starts from zero (
0
).
Example:
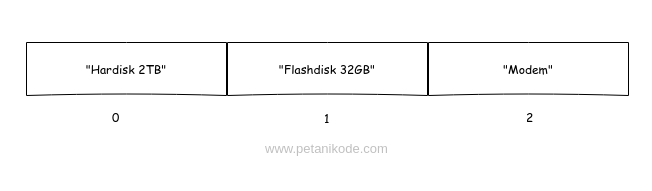
So, if we want to display "2TB Hardisk", then we have to display the 0th index.
For more details, let's try ...
2. Create an Array in PHP
We can create arrays in PHP with
array()
square functions and square brackets []
.
Example:
<?php
// membuat array kosong
$buah = array();
$hobi = [];
// membuat array sekaligus mengisinya
$minuman = array("Kopi", "Teh", "Jus Jeruk");
$makanan = ["Nasi Goreng", "Soto", "Bubur"];
// membuat array dengan mengisi indeks tertentu
$anggota[1] = "Dian";
$anggota[2] = "Muhar";
$anggota[0] = "Petani Kode";
It's easy, isn't it?
Anyway, we can fill arrays with any data type. Even mixed may be.
Example:
<?php
$item = ["Bunga", 123, 39.12, true];
3. Display the contents of the array
To display the contents of an array, we can access it through an index.
Example:
<?php
// membuat array
$barang = ["Buku Tulis", "Penghapus", "Spidol"];
// menampilkan isi array
echo $barang[0]."<br>";
echo $barang[1]."<br>";
echo $barang[2]."<br>";
The result:
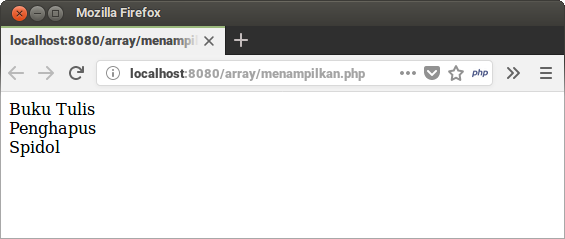
But this method is not effective, because we print one by one. Later, if the data is 1000, it means you have
echo
to type 1000 peritnah .
Then what?
Usually we use looping.
Example:
<?php
// membuat array
$barang = ["Buku Tulis", "Penghapus", "Spidol"];
// menampilkan isi array dengan perulangan for
for($i=0; $i < count($barang); $i++){
echo $barang[$i]."<br>";
}
We can use a function
count()
to calculate the number of contents of an array. In the example above the array contents are 3, then the loop will be 3x.
The result:
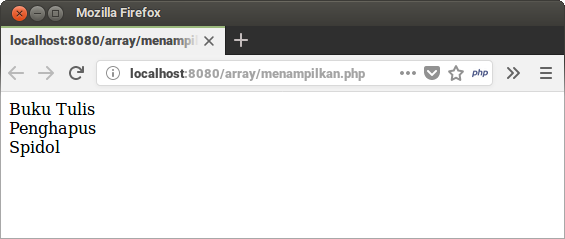
Besides using looping
for
, we can also use looping while
and foreach
.
Example:
<?php
// membuat array
$barang = ["Buku Tulis", "Penghapus", "Spidol"];
// menampilkan isi array dengan perulangan foreach
foreach($barang as $isi){
echo $isi."<br>";
}
echo "<hr>";
// menampilkan isi array dengan perulangan while
$i = 0;
while($i < count($barang)){
echo $barang[$i]."<br>";
$i++;
}
The result:
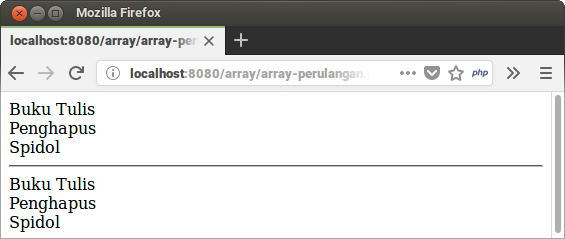
4. Erase the contents of the array
To delete the contents of an array, we can use the function
unset()
. This function can also be used to delete variables.
Example:
<?php
// membuat array
$hewan = [
"Burung",
"Kucing",
"Ikan"
];
// menghapus kucing
unset($hewan[1]);
echo $hewan[0]."<br>";
echo $hewan[1]."<br>";
echo $hewan[2]."<br>";
echo "<hr>";
echo "<pre>";
print_r($hewan);
echo "</pre>";
The result:
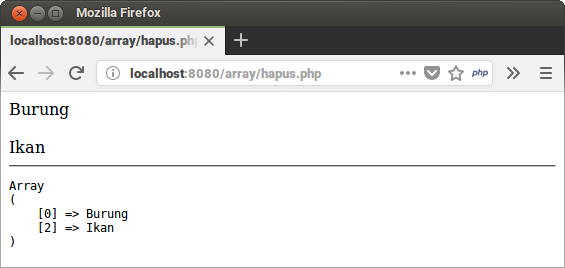
In the example above, we use a function
print_r()
to display raw (raw) arrays . Usually this function is used for debugging .5. Add Array contents
There are two ways you can add an array:
- Fill directly into the index number you want to add
- Fill directly into the last index
Let's try both.
<?php
// membuat array
$hobi = [
"Membaca",
"Menulis",
"Ngeblog"
];
// menambahkan isi pada idenks ke-3
$hobi[3] = "Coding";
// menambahkan isi pada indeks terakhir
$hobi[] = "Olahraga";
// cetak array dengan perulangan
foreach($hobi as $hobiku){
echo $hobiku."<br>";
}
?>
The result:
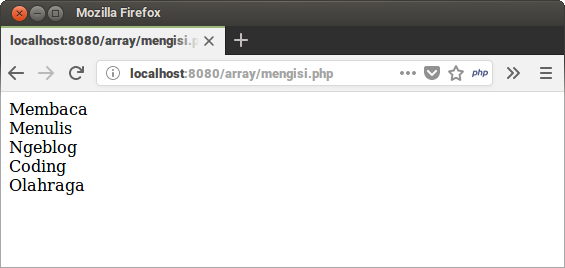
If we add to an index that already has content, then the content will be overwritten with the new one.
Example:
<?php
// membuat array
$user = [
"dian",
"muhar",
"petanikode"
];
// mengisi array pada indek ke-1 ("muhar")
$user[1] = "ardianta";
// mencetak isi array
echo "<pre>";
print_r($user);
echo "</pre>";
?>
The result:
Array
(
[0] => dian
[1] => ardianta
[2] => petanikode
)
6. Associative Arrays
An associative array is an array whose index does not use numbers or numbers. Associative array index in the form of keywords.
Example:
<?php
// membuat array asosiatif
$artikel = [
"judul" => "Belajar Pemrograman PHP",
"penulis" => "petanikode",
"view" => 128
];
// mencetak isi array assosiatif
echo "<h2>".$artikel["judul"]."</h2>";
echo "<p>oleh: ".$artikel["penulis"]."</p>";
echo "<p>View: ".$artikel["view"]."</p>";
The result:
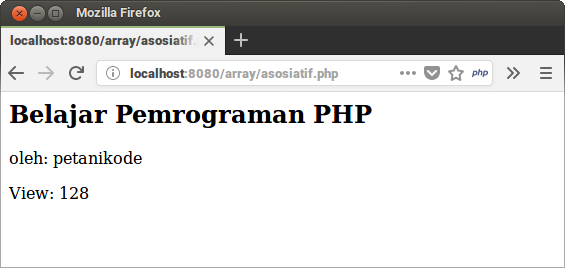
In associative arrays, we use a sign
=>
to associate a keyword with the contents of an array. In addition to using tags =>
, we can also create an associative array like this:<?php
$email["subjek"] = "Apa Kabar?";
$email["pengirim"] = "dian@petanikode.com";
$email["isi"] = "Apa kabar? sudah lama tidak berjumpa";
echo "<pre>";
print_r($email);
echo "</pre>";
The result:
Array
(
[subjek] => Apa Kabar?
[pengirim] => dian@petanikode.com
[isi] => Apa kabar? sudah lama tidak berjumpa
)
7. Multi Dimensional Arrays
Multi-dimensional arrays are arrays that have more than one dimension. Usually used to make matrices, graphs, and other complicated data structures.
Example:
<?php
// ini adalah array dua dimensi
$matrik = [
[2,3,4],
[7,5,0],
[4,3,8],
];
// cara mengakses isinya
echo $matrik[1][0]; //-> output: 7
We still try another example:
<?php
// membuat array 2 dimensi yang berisi array asosiatif
$artikel = [
[
"judul" => "Belajar PHP & MySQL untuk Pemula",
"penulis" => "petanikode"
],
[
"judul" => "Tutorial PHP dari Nol hingga Mahir",
"penulis" => "petanikode"
],
[
"judul" => "Membuat Aplikasi Web dengan PHP",
"penulis" => "petanikode"
]
];
// menampilkan array
foreach($artikel as $post){
echo "<h2>".$post["judul"]."</h2>";
echo "<p>".$post["penulis"]."<p>";
echo "<hr>";
}
The result:
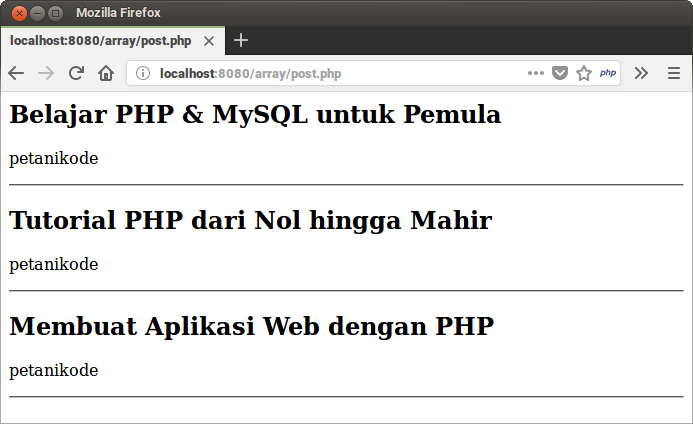
Reference: https://www.petanikode.com/php-array/
0 Komentar untuk "Learning PHP: 7 Things You Need to Know About Arrays in PHP"
Silahkan berkomentar sesuai artikel