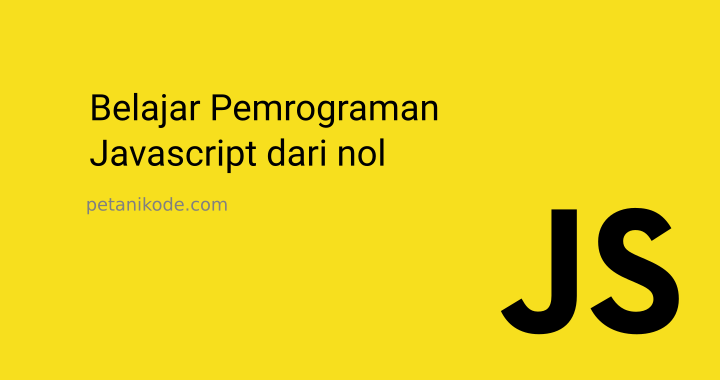
When you decide to learn Javascript, you must understand about DOM.
Because…
One of the main tasks of Javascript on the web is to make web pages look dynamic.
This can be done by Javascript with the help of DOM.
What is the DOM API?
... and how do you use it?
Let's discuss ...
What is the DOM API?
DOM stands for Document Object Model .
That is, documents (HTML) are modeled in an object.
The object of this document provides a set of functions and attributes / data that we can use to create a Javascript program. This is what is called the API (Application Programming Interface) .
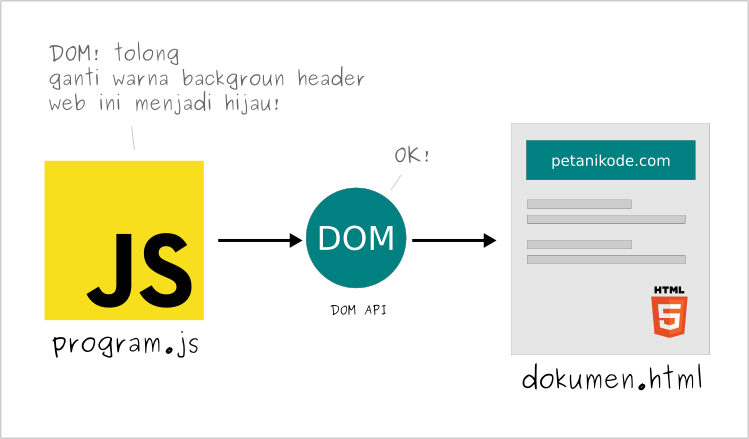
The DOM is not just for HTML documents. DOM can also be used for XML and SVG documents.
... and DOM is not only in Javascript, but also in other programming languages.
How to use DOM?
As we already know, DOM is an object to model HTML documents.
DOM object in javascript named
document
. This object contains everything we need to manipulate HTML.
If we try to type
document
in the Javascript console , the HTML code will appear.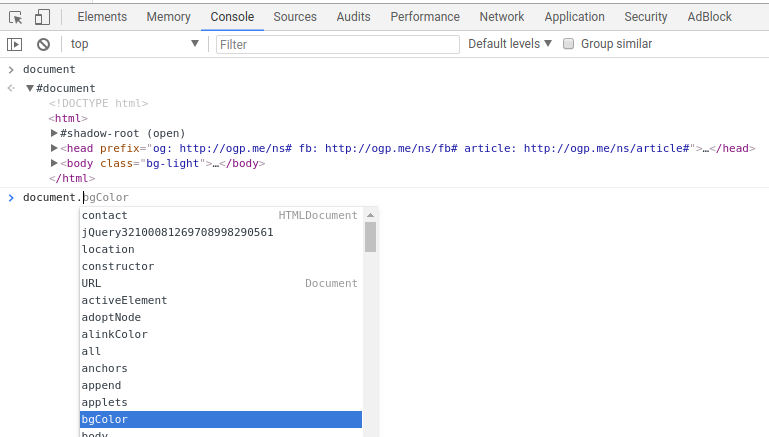
Inside the object
document
, there are functions and attributes that we can use to manipulate HTML documents.
For example a function
documen.write()
.
This function is used to write something to an HTML document.
Example:
Try typing the following code in the Javascript consoel .
document.write("Hello World");
document.write("<h2>Saya Sedang Belajar Javascript</h2>");
The result:
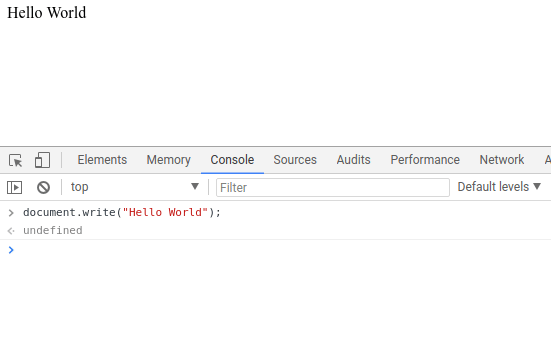
Look!
The results will directly impact the HTML document.
Accessing Certain Elements with DOM
The object
document
is a model of an HTML document. This object contains a collection of functions and attributes in the form of objects from HTML elements that can be described in the form of trees like this: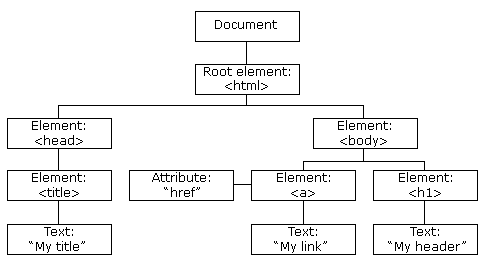
This tree structure will make it easier for us to use certain elements.
Let's try to access the object
<head>
and <body>
.
Try typing the following code in the Console:
// mengakses objek head
document.head;
// mengakses objek body
document.body;
// melihat panakang judul pada objek title
document.title.length
The result:
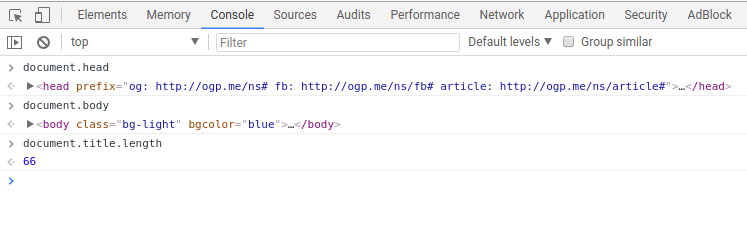
If we want to access specific elements, there are several functions that can be used:
getElementById()
function for selecting elements based on attributesid
.getElementByName()
function for selecting elements based on attributesname
.getElementByClassName()
function for selecting elements based on attributesclass
.getElementByTagName()
function for selecting elements based on tag names.getElementByTagNameNS()
function for selecting elements based on tag names.querySelector()
function for selecting elements based on queries.querySelectorAll()
function for selecting elements based on queries.- and others.
The above functions are often used to access certain elements.
Example:
Suppose we have an HTML code like this:
<div id="tutorial"></div>
So to choose these elements in Javascript, we can use functions
getElementByI()
like this:// memilih elemen dengan id 'tutorial'
var tutorial = document.getElementById('tutorial');
The variable
tutorial
will be a DOM object from the element we choose.
A more complete example:
<!DOCTYPE html>
<html>
<head>
<title>Memilih Elemen Berdasarkan ID</title>
</head>
<body>
<!-- Elemen div yang akan kita pilih dari JS -->
<div id="tutorial"></div>
<script type="text/javascript">
// mengakses elemen tutorial
var tutorial = document.getElementById("tutorial");
// mengisi teks ke dalam elemen
tutorial.innerText = "Tutorial Javascript";
// memberikan CSS ke elemen
tutorial.style.backgroundColor = "gold";
tutorial.style.padding = "10px";
</script>
</body>
</html>
The result:
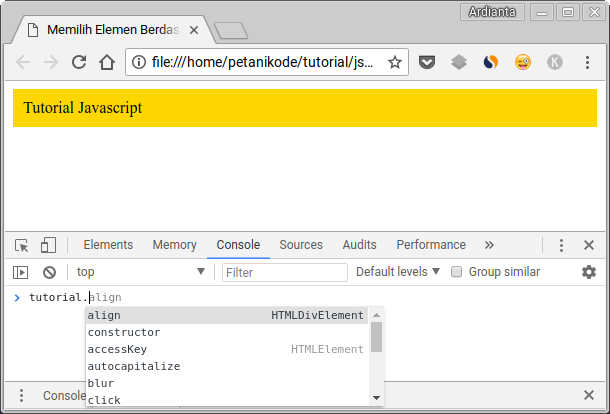
In the example above, we select elements with functions
document.getElementById()
. Then create an object for that element. After that, we can do whatever you want like changing the text and CSS style.
Then the question:
What if there is more than one element selected?
Suppose we choose an element based on the name tag or class attribute.
The answer:
The eelemn to be selected will be an array. Because we choose a set of elements.
The array will contain DOM objects from selected elements.
Example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>DOM API Javascript</title>
</head>
<body>
<p class="paragraf">Lorem ipsum dolor sit amet consectetur adipisicing elit.
Quo quaerat recusandae qui ullam eaque cumque ea fugit,
debitis commodi ipsum illo dolorum consequatur sed laudantium suscipit quis magni,
maiores in?</p>
<p class="paragraf">Lorem ipsum dolor sit amet consectetur adipisicing elit.
Quo quaerat recusandae qui ullam eaque cumque ea fugit,
debitis commodi ipsum illo dolorum consequatur sed laudantium suscipit quis magni,
maiores in?</p>
<p class="paragraf">Lorem ipsum dolor sit amet consectetur adipisicing elit.
Quo quaerat recusandae qui ullam eaque cumque ea fugit,
debitis commodi ipsum illo dolorum consequatur sed laudantium suscipit quis magni,
maiores in?</p>
<script>
var paragraf = document.getElementsByClassName("paragraf");
console.log(paragraf);
</script>
</body>
</html>
In the example above, we have three paragraphs with the class name
paragraf
.
Then we try to select the three paragraphs through javascript with the method or function
getElementByClassName()
.
Then, the results are displayed in the Javascript console .
Then the result:
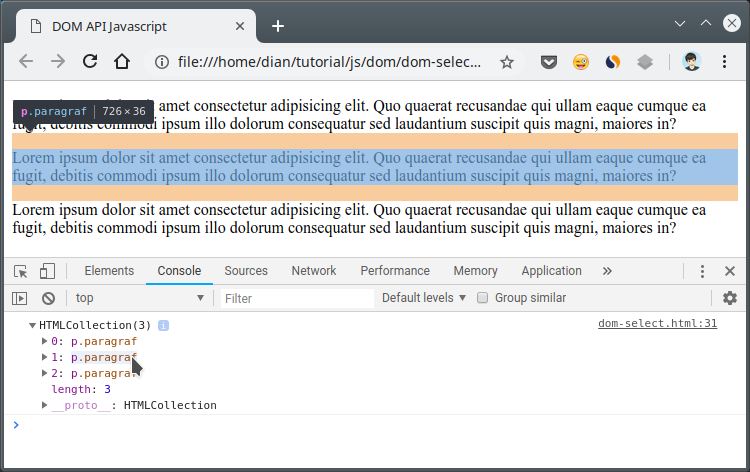
The variable
paragraf
will contain an array in which there are three DOM objects from the paragraph.
Let's try experimenting by changing the color of the text from the first paragraph.
The first paragraph will be in the index position
0
in the array.
Try typing the following command in the Javascript console :
paragraf[0].style.color = "red"
So the result, the first paragraph will be red.
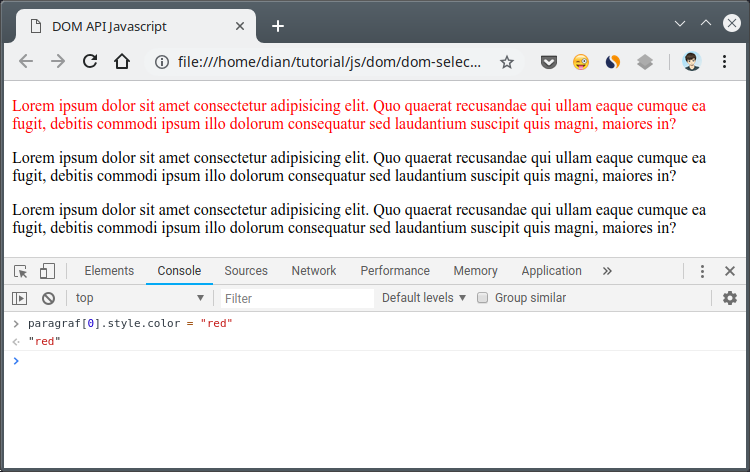
Let's try to create a color animation.
Change the above javascript code to something like this:
<script>
var paragraf = document.getElementsByClassName("paragraf");
setInterval(function () {
paragraf[0].style.color = "red";
paragraf[1].style.color = "green";
paragraf[2].style.color = "blue";
setTimeout(function () {
paragraf[0].style.color = "black";
paragraf[1].style.color = "black";
paragraf[2].style.color = "black";
}, 500)
}, 1000);
</script>
We use functions
setInterval()
and functions setTimeOut()
to determine the animation time.
In the above code, we give milliseconds or 1 second time interval
1000
.
Meanwhile, to change the color to black, we give a
500
millisecond or 0.5 seconds.
Then the result:
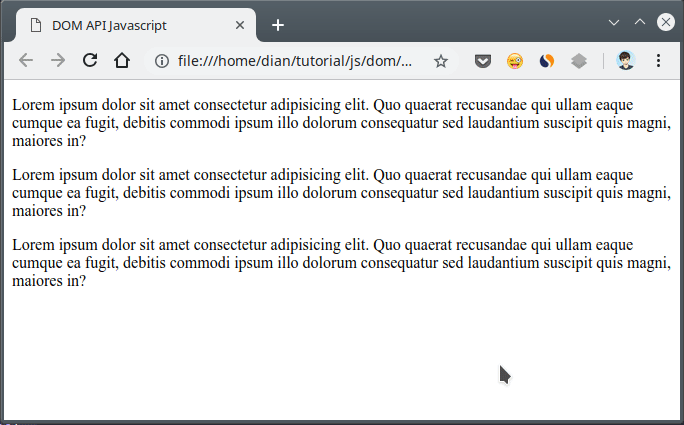
Creating Elements with the DOM API
DOM also provides functions to create HTML elements.
One of them is a function
createElement()
.
Example:
document.createElement('p');
Then, a
<p>
new element will be created . But it will not be displayed on a web page.
Why isn't it displayed?
Because we haven't added it to the body of the document.
How to add it to the body of the document, we can use the function
append()
.
Example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>DOM API Javascript</title>
</head>
<body>
<script>
// membuat element h1
var judul = document.createElement("h1");
// mengisi kontent elemen
judul.textContent = "Belajar Javascript";
// menambahkan elemen ke dalam tag body
document.body.append(judul);
</script>
</body>
</html>
The result:
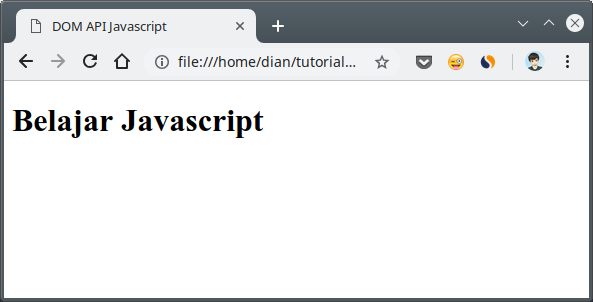
Removing Elements with DOM API
If we used the function
append()
to add elements, then to delete it we use the function remove()
.
The following is an example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>DOM API Javascript</title>
</head>
<body>
<h2 id="judul2">Delete Saya!</h2>
<script>
// memilih elemen berdasarkan ID
var h2 = document.getElementById('judul2');
// menghapus elemen yang sudah dipilih
h2.remove();
console.log("Elemen sudah dihapus");
console.log(h2);
</script>
</body>
</html>
The price:
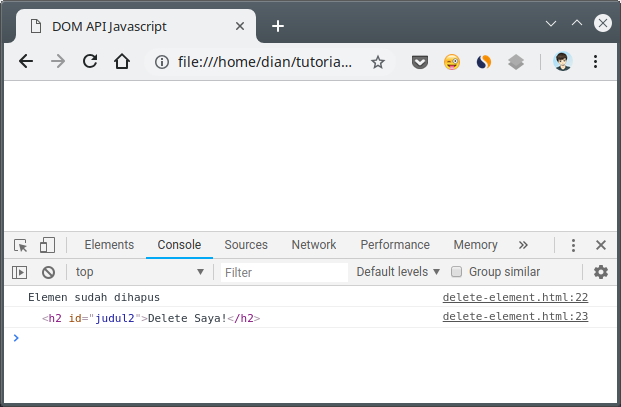
The element is successfully deleted from the web page, but the element is still in memory.
Examples of Programs Using DOM
We already know how to access elements with DOM and we also understand how to add and delete elements.
To make the understanding more stable, try the following example program:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>DOM API Javascript</title>
</head>
<body>
<h1>Aplikasi Ubah Warna</h1>
<label>Warna latar: </label>
<input type="color" id="bg-color" />
<br>
<label>Warna teks: </label>
<input type="color" id="text-color" />
<script>
var bgColor = document.getElementById('bg-color');
var txtColor = document.getElementById('text-color');
bgColor.addEventListener("change", (event) => {
document.body.style.backgroundColor = bgColor.value;
});
txtColor.addEventListener("change", (event) => {
document.body.style.color = txtColor.value;
});
</script>
</body>
</html>
This program functions to change the background color of the element
<body>
and change the color of the text.
We use events
"change"
on elements bgColor
and txtColor
. This means that later each value of this element changes, the code in it will be executed.
The result:
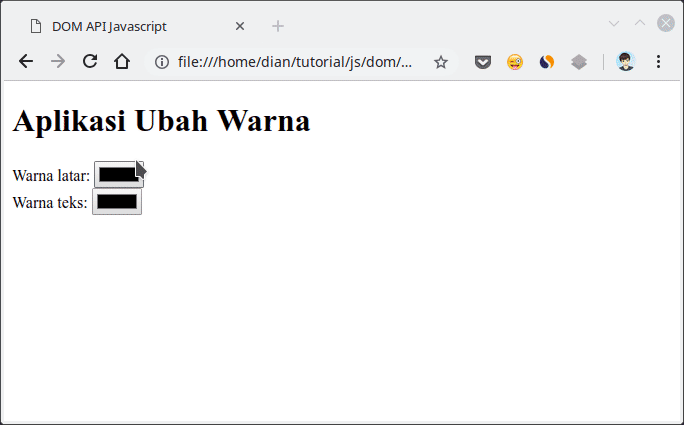
Reference: https://www.petanikode.com/javascript-dom/
0 Komentar untuk "Learning Javascript: What is the DOM API? and How to use it?"
Silahkan berkomentar sesuai artikel