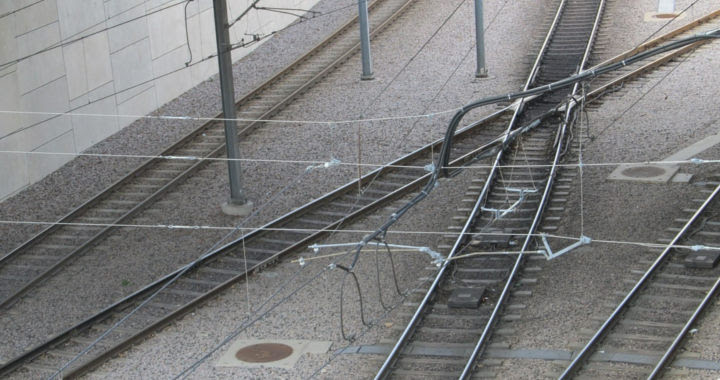
If we notice, the execution path of a program code is done one by one from top to bottom.
Line by line is read, then the computer does what it is told.
For example like this:
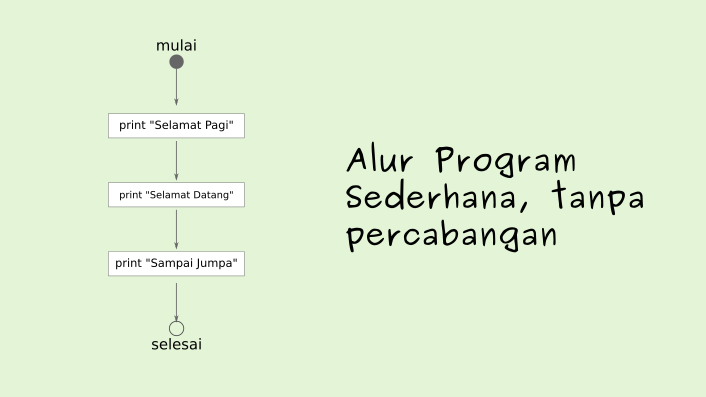
The flow of the program is one, there are no bends or branches.
By the way, what is branching?
Branching is just a term used to refer to a branched program flow.
Branching is also known as "Control Flow", "Structure Condition", "IF Structure", "Decision", etc. Everything is the same.
In the flow chart (Flow Chart) as above, the flow is indeed one.
But after we use branching, the plot will grow to be like this.
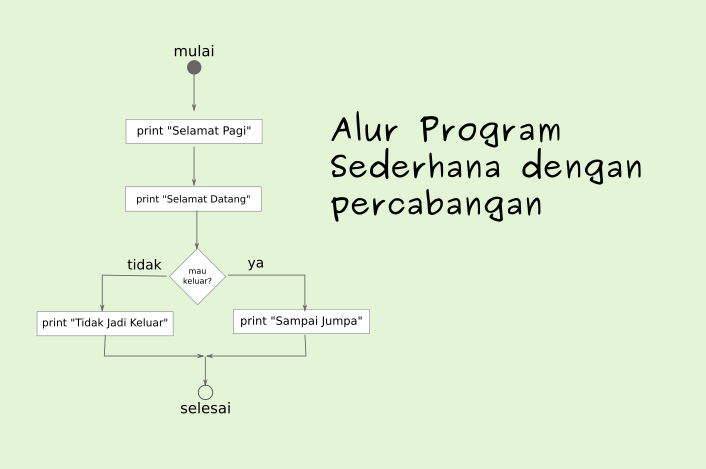
Then how do you write branching code in Java?
The trick: using keywords
if
, else
, switch
, and case
, and the ternary operator.
Examples of IF structure formats like this:
if( suatu_kondisi ) {
// lakukan sesuatu kalau kondisi benar
// Lakukan ini juga
}
suatu_kondisi
only worth true
/ false
just. We can use the relations and logic operators here.
For more details, we will discuss later.
Previously, you need to know the three forms of branching in Java:
- IF branching
- Branching of IF / ELSE
- Branching of IF / ELSE / IF or SWITCH / CASE
Let's discuss them one by one…
1. IF branching
This branching has only one choice. That is, the choices inside the IF will only be done if the conditions are right.
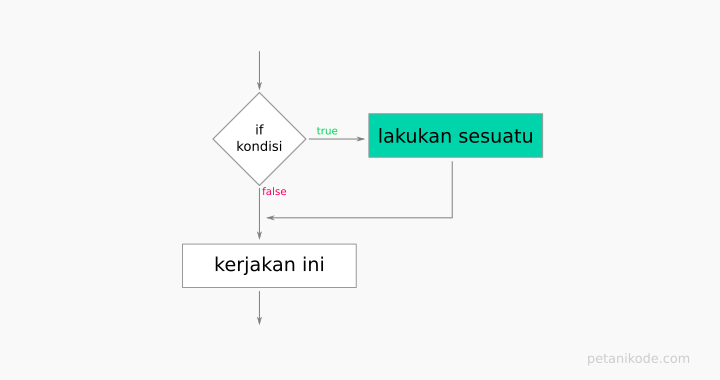
But if it's wrong ... it won't do anything. Then proceed to the next command.
Example:
Have you ever shop at a store, then if you shop above there are thousands of prizes or discounts.
Well! Examples of such cases, we can solve using this branching.
for more details…
Let's Make a Prize Program
Suppose there is a bookstore. They give gifts in the form of school supplies to buyers who spend above Rp. 100,000.
So we can make the program like this:
import java.util.Scanner;
public class Hadiah {
public static void main(String[] args) {
// membuat variabel belanja dan scanner
int belanja = 0;
Scanner scan = new Scanner(System.in);
// mengambil input
System.out.print("Total Belanjaan: Rp ");
belanja = scan.nextInt();
// cek apakah dia belanja di atas 100000
if ( belanja > 100000 ) {
System.out.println("Selamat, anda mendapatkan hadiah!");
}
System.out.println("Terima kasih...");
}
}
Run the program and pay attention to the results.
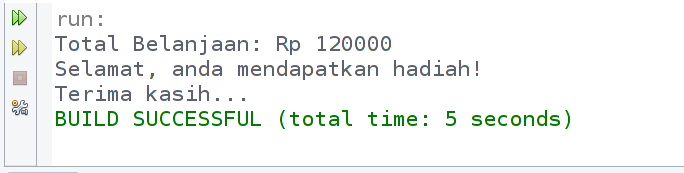
Try to give the value below
100000
and note what will happen.2. Branching of IF / ELSE
Whereas IF / ELSE branching has an alternative choice if the condition is wrong.
IF : "If the conditions are right then do this, if not, please continue"
IF / ESLE : "If the conditions are right then do this, if it's wrong then do the one, after that continue"
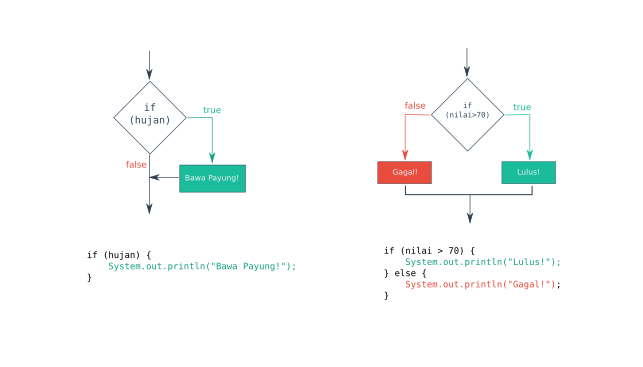
That's the difference between IF and IF / ELSE.
Now let's try it in the program code ...
Graduation Check Program
For example, if the value of a student is greater than 70, then he is declared a graduate. If not, then he fails.
We can make the program like this:
import java.util.Scanner;
public class CekKelulusan {
public static void main(String[] args) {
// membuat variabel dan Scanner
int nilai;
String nama;
Scanner scan = new Scanner(System.in);
// mengambil input
System.out.print("Nama: ");
nama = scan.nextLine();
System.out.print("Nilai: ");
nilai = scan.nextInt();
// cek apakah dia lulus atau tidak
if( nilai >= 70 ) {
System.out.println("Selemat " + nama + ", anda lulus!");
} else {
System.out.println("Maaf " + nama + ", anda gagal");
}
}
}
The output results:
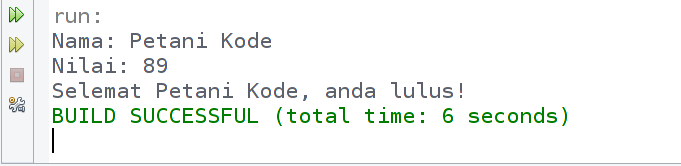
Try to change the entered value and notice what will happen.
IF / ELSE branching with Ternary Operators
In addition to using the structure as above, this store can also use a ternary operator.
As we have learned in the discussion about operators . Ternary operators have the same concept as IF / ELSE partnerships.
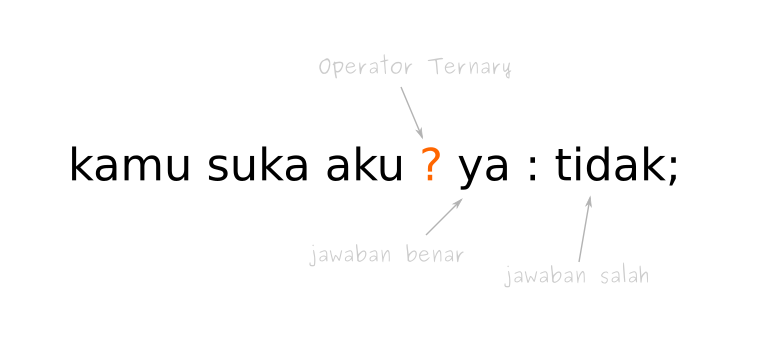
Example program:
public class OperatorTernary {
public static void main(String[] args) {
boolean suka = true;
String jawaban;
// menggunakan operator ternary
jawaban = suka ? "iya" : "tidak";
// menampilkan jawaban
System.out.println(jawaban);
}
}
3. Branching of IF / ELSE / IF and SWITCH / CASE
If IF / ESLE branching only has two choices. So IF / ELSE / IF conversations have more than two choices.
Format it like this:
if (suatu kondisi) {
// maka kerjakan ini
// kerjakan perintah ini juga
// …
} else if (kondisi lain) {
// kerjakan ini
// kerjakan ini juga
// …
} else if (kondisi yang lain lagi) {
// kerjakan perintah ini
// kerjakan ini juga
// …
} esle {
// kerjakan ini kalau
// semua kondisi di atas
// tidak ada yang benar
// …
}
Try to consider an example:
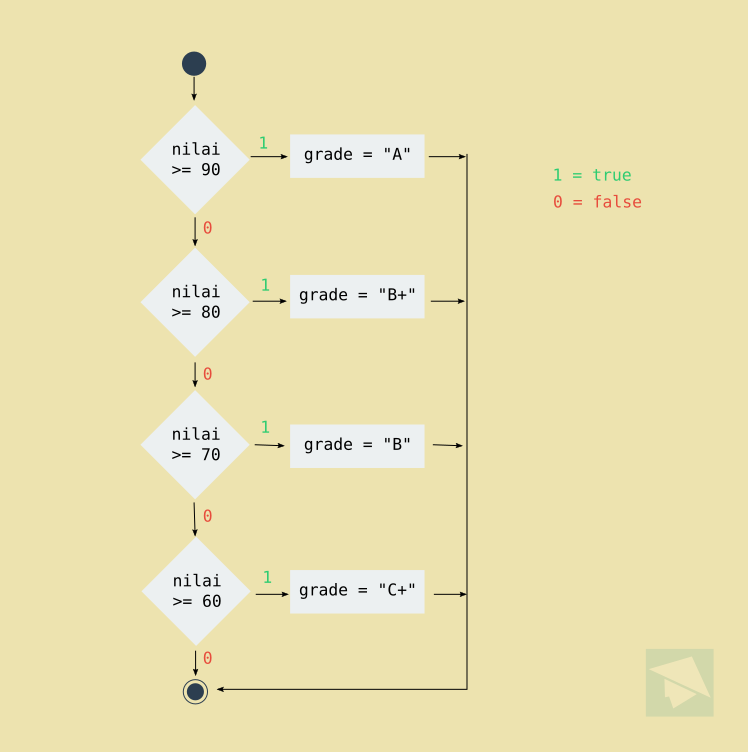
If the value is greater than
90
, then the grade is "A". Whereas if it's bigger than 80
, then "B +". Greater than 70
, then "B", and so on.
For more details, let's make a program.
Calculate the Grade Program
Please create a new class named
HitungGrade
, then follow the following program code.import java.util.Scanner;
public class HitungGrade {
public static void main(String[] args) {
// membuat variabel dan scanner
int nilai;
String grade;
Scanner scan = new Scanner(System.in);
// mengambil input
System.out.print("Inputkan nilai: ");
nilai = scan.nextInt();
// higung gradenya
if ( nilai >= 90 ) {
grade = "A";
} else if ( nilai >= 80 ){
grade = "B+";
} else if ( nilai >= 70 ){
grade = "B";
} else if ( nilai >= 60 ){
grade = "C+";
} else if ( nilai >= 50 ){
grade = "C";
} else if ( nilai >= 40 ){
grade = "D";
} else {
grade = "E";
}
// cetak hasilnya
System.out.println("Grade: " + grade);
}
}
The output results:
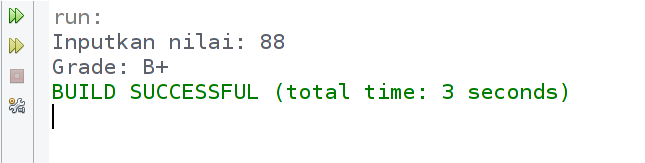
Branching SWITCH / CASE
Branching SWITCH / CASE is actually another form of IF / ELSE / IF.
The difference is, this branching uses keywords
switch
and case
.
The format is also different, but the way it works is the same.
switch(variabel){
case 1:
// kerjakan kode ini
// kode ini juga
break;
case 2:
// kerjakan kode ini
// kode ini juga
break;
case 3:
// kerjakan kode ini
// kode ini juga
break;
default:
// kerjakan kode ini
// kode ini juga
break;
}
Note: it
case 1
means the value variabel
to be compared, is the value equal to 1
or not.
If so, then do the code inside
case 1
.
It can also be different, for example like this:
switch (variabel) {
case 'A':
// lakukan sesuatu
break;
case 'B':
// lakukan ini
break;
default:
// lakukan ini
}
Also note: there are keywords
break
and default
.break
meaning stop. This is to instruct the computer to stop checkingcase
the others.default
meaning if the variable value is not the same as the choice of the case above, then work on the code insidedefault
.
Choice
default
can also not have break
, because he is the last choice. This means that checking will end there.
Examples of programs with branching SWITCH / CASE
import java.util.Scanner;
public class LampuLalulintas {
public static void main(String[] args) {
// membuat variabel dan Scanner
String lampu;
Scanner scan = new Scanner(System.in);
// mengambil input
System.out.print("Inputkan nama warna: ");
lampu = scan.nextLine();
switch(lampu){
case "merah":
System.out.println("Lampu merah, berhenti!");
break;
case "kuning":
System.out.println("Lampu kuning, harap hati-hati!");
break;
case "hijau":
System.out.println("Lampu hijau, silahkan jalan!");
break;
default:
System.out.println("Warna lampu salah!");
}
}
}
The output results:
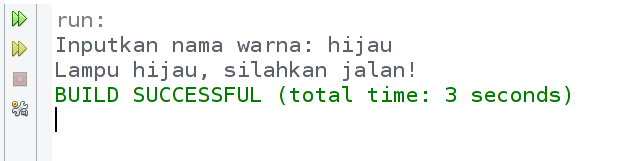
Experiment : Try to eliminate it
break
in one case
and pay attention to the results.Branching in Branching (Nested)
We already know the three basic forms of conversation in Java. Next, we try to discuss the branching that is in the crossing (nesting bridge).
Actually, this discussion I want to separate. However, it's good to just combine it here.
Think of it as a bonus discussion
.
OK…
So, the branching can be made in branching. Sometimes this technique is also called nested if .
Sample case:
For example, there is a business model like this in a shop. When people pay at the checkout, it is usually asked that there is a member card to get discounts and so on.
Apakah anda punya kartu member?
- ya
* Apakah belanjaan anda lebih dari 500rb?
# ya : mendapatkan diskon 50rb
# tidak : tidak mendapatkan diskon
* Apakah belanjaan anda lebih dari 100rb?
# ya : mendapatkan diskon 15rb
# tidak: tidak mendapatkan diskon
- tidak
* Apakah belanjaan anda lebih dari 100rb?
# ya : mendapatkan diskon 10rb
# tidak: tidak mendapatkan diskon
Understand?
If not, try to look at the flow chart:
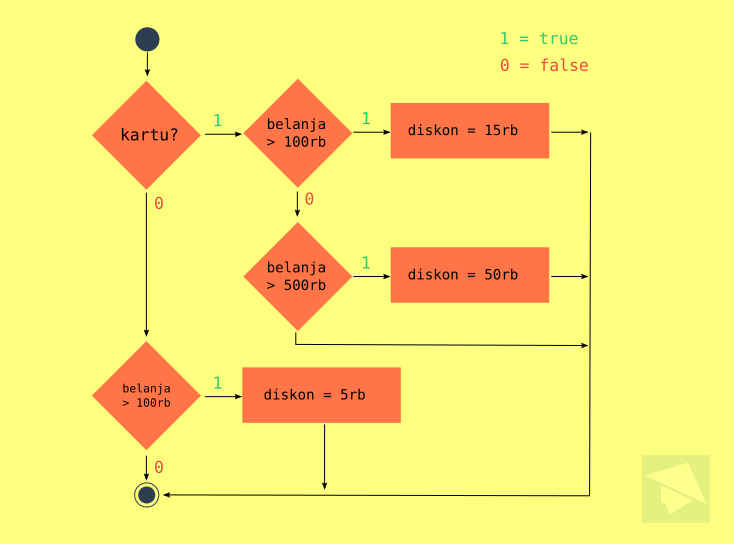
Still don't understand?
Then let's try it in the program.
Please create a new class named
Kasir
and follow the program code below.import java.util.Scanner;
public class Kasir {
public static void main(String[] args) {
// deklarasi variabel dan Scanner
int belanjaan, diskon, bayar;
String kartu;
Scanner scan = new Scanner(System.in);
// mengambil input
System.out.print("Apakah ada kartu member: ");
kartu = scan.nextLine();
System.out.print("Total belanjaan: ");
belanjaan = scan.nextInt();
// proses
if (kartu.equalsIgnoreCase("ya")) {
if (belanjaan > 500000) {
diskon = 50000;
} else if (belanjaan > 100000) {
diskon = 15000;
} else {
diskon = 0;
}
} else {
if (belanjaan > 100000) {
diskon = 5000;
} else {
diskon = 0;
}
}
// total yang harus dibayar
bayar = belanjaan - diskon;
// output
System.out.println("Total Bayar: Rp " + bayar);
}
}
The output results:
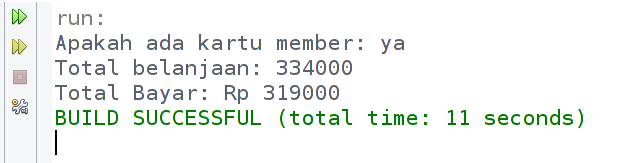
Try to change the value entered and note the results.
Maybe there is something to note:
- The function is
equalsIgnoreCase("ya")
used to compare strings regardless of upper and lower case letters. - There are also functions
equals()
, the same function. Butequals()
will pay attention to the case letters.
Why not use an operator
==
or !=
?
In Java it is like that.
If we want to compare String values, yes ... use the two functions above.
But, if you compare other than String, you can use the operator
==
or !=
.Using Logic Operators in Branching
Logical operators in branching can actually make branching shorter.
Suppose there is a ticket program with logic like this:
public class Tilang {
public static void main(String[] args) {
boolean SIM = false;
boolean STNK = true;
// cek apakah dia akan ditilang atau tidak
if(SIM == true){
if( STNK == true ) {
System.out.println("Tidak ditilang!");
}
} else {
System.out.println("Anda ditilang!");
}
}
}
Note: there we use a nesting branch to check whether it is ticketed or not.
This can actually be abbreviated with a logical operator, so it becomes like this:
public class Tilang {
public static void main(String[] args) {
boolean SIM = false;
boolean STNK = true;
// cek apakah dia akan ditilang atau tidak
if(SIM == true && STNK == true){
System.out.println("Tidak ditilang!");
} else {
System.out.println("Anda ditilang!");
}
}
}
In the above code, we use operator AND (
&&
).
Because of the logic: The driver will not be ticketed if he has a driver's license and vehicle registration.
0 Komentar untuk "Learning Java: Understanding the 3 Forms of Branching in Java"
Silahkan berkomentar sesuai artikel