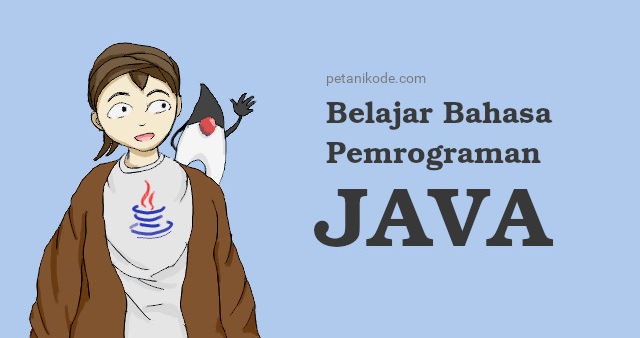
Keywords
this
and super
often appear in Java programs.
What is the function?
On this occasion, we will discuss it ...
Because it is very related to our discussion here.
OK.
Let's start tips ...
Functions this
in Java
In the previous tutorial, we learned about setter and gettermethods .
There we use keywords
this
to fill variables.
Example:
class User {
private String username;
private String password;
// ini method setter
public void setUsername(String username){
this.username = username;
}
public void setPassword(String password){
this.password = password;
}
// ini method getter
public String getUsername(){
return this.username;
}
public String getPassword(){
return this.password;
}
}
See!
this
we use on each method.
Consider one method:
public void setUsername(String username){
this.username = username;
}
What if we delete
this
this method?public void setUsername(String username){
username = username;
}
What will happen?
Yak! the computer will be confused ...
Because they don't know
username
which variable is meant.
Is the variable in class (
private String username
) or the variable username
in the parameter.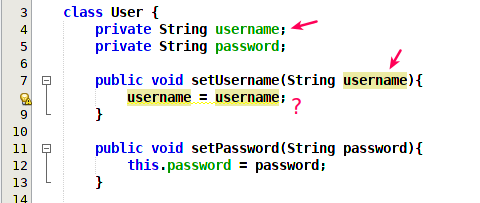
If we touch the warning balloon on the side, we will get a message: "Assignment To Itself" .
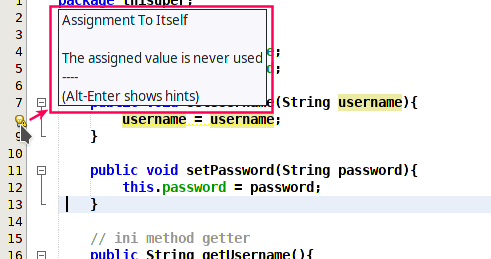
This means that the variable fills itself.
Therefore, we must use
this
to declare the variable in question is the variable that is in the class.public void setUsername(String username){
this.username = username;
}
So…
The keyword is
this
used as a reference from the class itself.
Still confused?
Okay, then ... every time you
this
try to use this view:this
means the class itself.
For example there is another class:
class Person {
private String name;
public void setName(String name){
this.name = name;
}
}
So
this
what is meant by that class is class Person
.
Can we use
this
outside the class?
Not.
this
can only be used in class.
Try making a function
main()
then use it this
there. Then what will happen is ...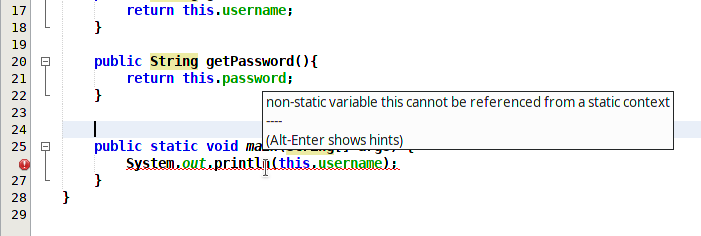
Yak! the program will error.
Because the variable we are trying to access from
main()
is a non-static variable.
... and
this
cannot be used on main()
.
Functions super
in Java
If it
this
represents an object of the class itself, it super
will represent the object of the parent class.
Consider this example:
We try to make the class
Person
that will be the parent class or super class.public class Person {
String name = "Petanikode";
int age = 22;
}
Then, we create derived classes (sub classes), namely: class
Employee
.public class Employee extends Person {
float salary = 4000f;
String name = "Dian";
int age = 23;
public void showInfo(){
System.out.println("Name: " + super.name);
System.out.println("Age: " + super.age);
System.out.println("Salary: $" + salary);
}
}
Next we create a class
Demo
to create objects and methods main()
.public class Demo {
public static void main(String[] args) {
Employee dian = new Employee("Dian", 23, 4000f);
dian.showInfo();
}
}
Now try executing the class
Demo
, what is the output?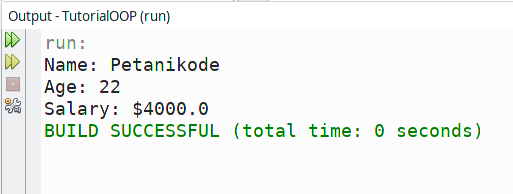
Why are the outputs like this?
Because in the method
showInfo()
we use keywords super
to take the value of the variables that are in the parent class (super class) .public void showInfo(){
System.out.println("Name: " + super.name);
System.out.println("Age: " + super.age);
System.out.println("Salary: $" + salary);
}
Try changing
super
to this
...public void showInfo(){
System.out.println("Name: " + this.name);
System.out.println("Age: " + this.age);
System.out.println("Salary: $" + salary);
}
... then the output will be like this.
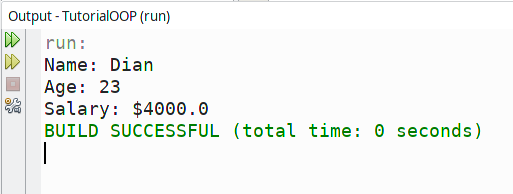
The value taken is the value of the class
Employee
, because itthis
represents the class Employee
while super
representing the parent classes, namely: Person
.
In this case, we use
super
as an object to take value.
The keyword
super()
can also be used to call the ternetu method from the parent class.
Example:
// mengeksekusi konstruktor induk
super();
// mengeksekusi sebuah method dari class induk
super.namaMethod();
Let's try ...
We change the class
Person
to something like this:public class Person {
public Person() {
System.out.println("Eksekusi konstruktor Person()");
}
public void showMessage(){
System.out.println("Hello, ini pesan dari class Person...");
}
}
Then
Employee
we change the class to something like this:public class Employee extends Person {
public Employee() {
// eksekusi konstruktor induk
super();
System.out.println("Ekekusi konstruktor Employee()");
}
public void info(){
super.showMessage();
}
}
Then in the class
Demo
, we change it to something like this:public class Demo {
public static void main(String[] args) {
Employee dian = new Employee();
dian.info();
}
}
Now try executing the class
Demo
, the output will look like this: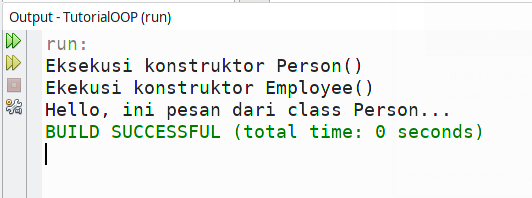
Notice the class
Employee
, in the constructor we execute the master constructor with super()
.
Then, in the method
info()
, we execute the method showMessage()
from the parent with super.showMessage()
.
Then the results, will be as above.
How is the Application this
and super
the Real World?
The examples above might sound abstract, because it is impossible for us to make such a program in the real world.
Well, we will try to look at some examples of programs in the real world that use
this
and super
.import javax.swing.JFrame;
public class MainWindow extends JFrame {
public MainWindow(){
this.setTitle("Jendela Utama");
this.setSize(600,320);
}
public static void main(String[] args) {
MainWindow mWindow = new MainWindow();
mWindow.setVisible(true);
}
}
In the program, we create a class
MainWindow
by extends from the class JFrame
.
In this program, it is
this
used to represent the classMainWindow
in the constructor.public MainWindow(){
this.setTitle("Jendela Utama");
this.setSize(600,320);
}
Method
setTitle()
and setSize()
obtained from the Parent class ( JFrame
). Because we extends from there.
Why not use
super
?
Because what we want to change
title
and size
is class MainWindow
, not class JFrame
.public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Toast.makeText(this, "Activity: onCreate()", Toast.LENGTH_SHORT).show();
}
}
Pay attention ...
In classes
MainActivity
, we use super
to call methodsonCreate()
from the parent class ( AppCompatActivity
).super.onCreate(savedInstanceState);
Then we use it
this
on Toast.makeText()
. this
in this case it will represent the class MainActivity
.
There are many more examples of use
this
and super
in the real world.
Please find yourself ... 
0 Komentar untuk "Learning Java OOP: What are the functions of 'this' and 'super' in Java?"
Silahkan berkomentar sesuai artikel