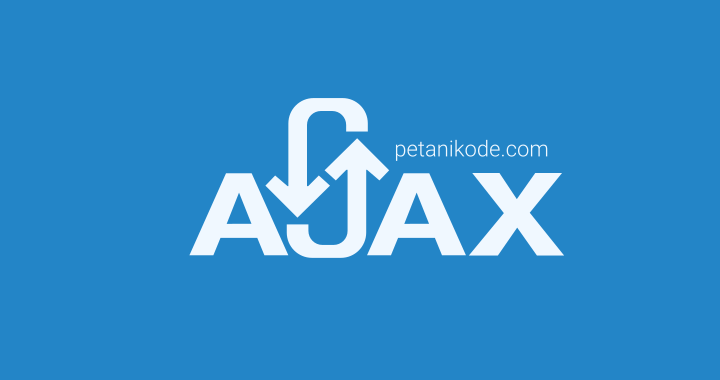
What is AJAX?
AJAX stands for A synchronous J JavaScript A and X ML.
AJAX serves to:
- Retrieve data from the server in the background ;
- Update web views without having to reload the browser;
- Send data to the server in the background .
Basically AJAX only uses objects
XMLHttpRequest
to communicate with the server.
We can see the AJAX process through inspecting elements in a web browser , then open the Network tab and activate the XHR (XML HTTP Request) filter .
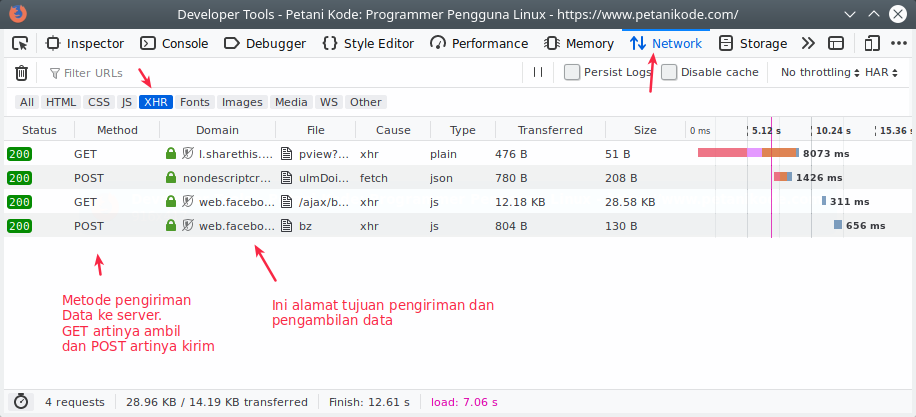
How to use Ajax in JavaScript
The steps to using AJAX like this:
- Create Ajax Objects
var xhr = new XMLHttpRequest();
- Determine the Function of the Handler for Events
xhr.onreadystatechange = function() { ... }; xhr.onload = function() { ... }; xhr.onerror = function() { ... }; xhr.onprogress = function() { ... };
- Determine the Method and URL
xhr.open("GET", url, true);
- Send Request
xhr.send();
Okay…
Let's try it.
Make an HTML file with the contents as follows:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Belajar Dasar Ajax</title>
</head>
<body>
<h1>Tutorial Ajax</h1>
<div id="hasil"></div>
<button onclick="loadContent()">Load Content</button>
<script>
function loadContent(){
var xhr = new XMLHttpRequest();
var url = "https://api.github.com/users/petanikode";
xhr.onreadystatechange = function(){
if(this.readyState == 4 && this.status == 200){
document.getElementById("hasil").innerHTML = this.responseText;
}
};
xhr.open("GET", url, true);
xhr.send();
}
</script>
</body>
</html>
The result:
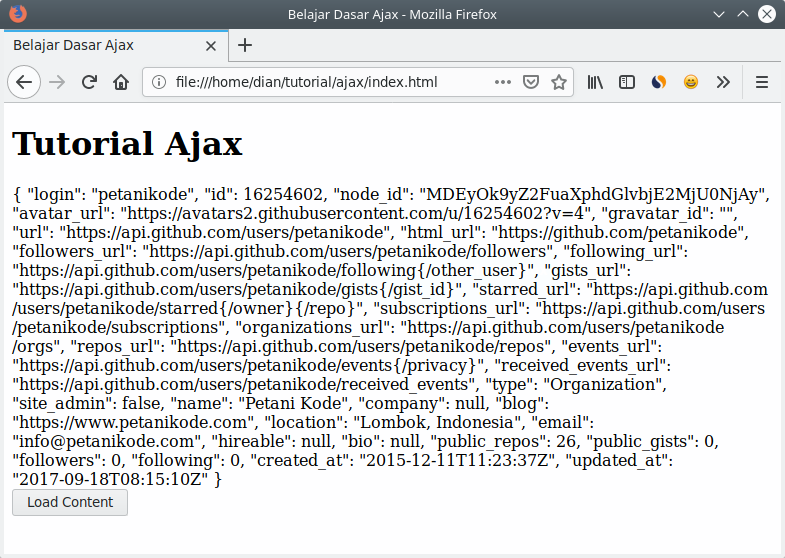
In the example above, we retrieve data from the Github server using the GET method. Then the results are directly entered into the element
<div id="hasil">
.
The event we use is
onreadystatechange
, in this event we can check the AJAX state and status.if(this.readyState == 4 && this.status == 200){
//...
}
Code | State | Information |
---|---|---|
0 | UNSENT | An AAJAX object has been created but has not called the method open() . |
1 | OPENED | The method open() has been called. |
2 | HEADERS_RECEIVED | The method send() has been called, and here is a status header. |
3 | LOADING | Downloading; is downloading data. |
4 | DONE | AJAX operation is complete. |
While for the status header
200
is the status of HTTP Request. Usually the above code 200
means good and below 200
means bad.
Then try to pay attention to this code:
xhr.open("GET", url, true);
There are three parameters that we give to the method
open()
:GET
is the request method to be used;url
is the destination URL address;true
is to execute AJAX asynchronously .
Let's continue with the other example. Please change the contents of the HTML file that was like this:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Belajar Dasar Ajax</title>
</head>
<body>
<h1>Tutorial Ajax <button id="btn-clear" onclick="clearResult()">Clear</button></h1>
<div id="hasil"></div>
<button id="button" onclick="loadContent()">Load Content</button>
<script>
function loadContent() {
var xhr = new XMLHttpRequest();
var url = "https://api.github.com/users/petanikode";
xhr.onloadstart = function () {
document.getElementById("button").innerHTML = "Loading...";
}
xhr.onerror = function () {
alert("Gagal mengambil data");
};
xhr.onloadend = function () {
if (this.responseText !== "") {
var data = JSON.parse(this.responseText);
var img = document.createElement("img");
img.src = data.avatar_url;
var name = document.createElement("h3");
name.innerHTML = data.name;
document.getElementById("hasil").append(img, name);
document.getElementById("button").innerHTML = "Done";
setTimeout(function () {
document.getElementById("button").innerHTML = "Load Lagi";
}, 3000);
}
};
xhr.open("GET", url, true);
xhr.send();
}
function clearResult() {
document.getElementById("hasil").innerHTML = "";
}
</script>
</body>
</html>
The result:
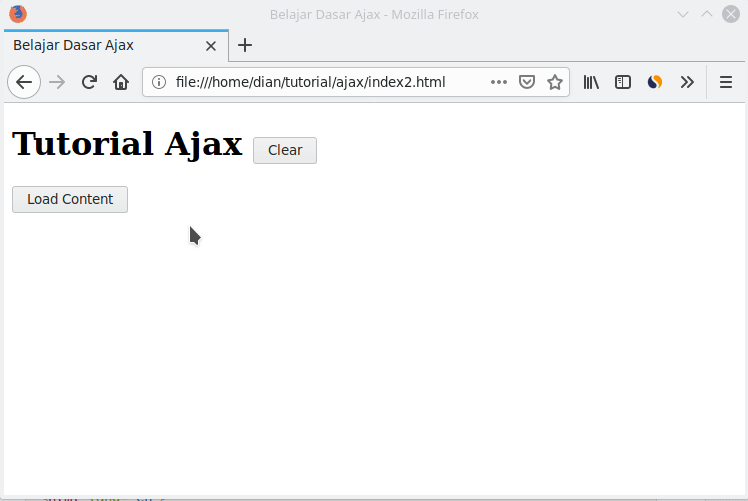
Sending Data to Server with AJAX
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Belajar Dasar Ajax</title>
</head>
<body>
<h1>Kirim Data dengan Ajax</h1>
<form method="POST" onsubmit="return sendData()">
<p>
<label>Title</label>
<input type="text" id="title" placeholder="judul artikel">
</p>
<p>
<label>Isi Artikel</label><br>
<textarea id="body" placeholder="isi artikel..." cols="50" rows="10"></textarea>
</p>
<input type="submit" value="Kirim" />
</form>
<script>
function sendData() {
var xhr = new XMLHttpRequest();
var url = "https://jsonplaceholder.typicode.com/posts";
var data = JSON.stringify({
title: document.getElementById("title").value,
body: document.getElementById("body").value,
userId: 1
});
xhr.open("POST", url, true);
xhr.setRequestHeader("Content-Type", "application/json;charset=UTF-8");
xhr.onload = function () {
console.log (this.responseText);
};
xhr.send(data);
return false;
}
</script>
</body>
</html>
The result:
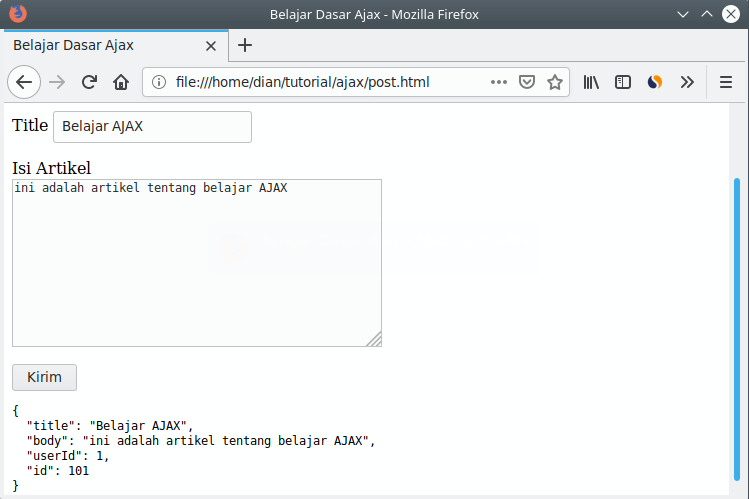
The data transmission is only for simulation. Data will not actually be sent to the server https://jsonplaceholder.typicode.com/posts .
AJAX Using JQuery
JQuery is a Javascript library that simplifies Javascript functions. In JQuery, AJAX can be created like this:
// load data ke elemen tertentu via AJAX
$(selector).load(URL,data,callback);
// ambil data dari server
$.get(URL,callback);
// kirim data dari Server
$.post(URL,data,callback);
Let's try ...
Create a new file named
ajax-jquery.html
then fill in the following code:<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Belajar AJAX dengan JQuery</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.0/jquery.min.js"></script>
</head>
<body>
<h1>Load Data</h1>
<pre id="result"></pre>
<script>
$("#result").load("https://api.github.com/users/petanikode");
</script>
</body>
</html>
The result:
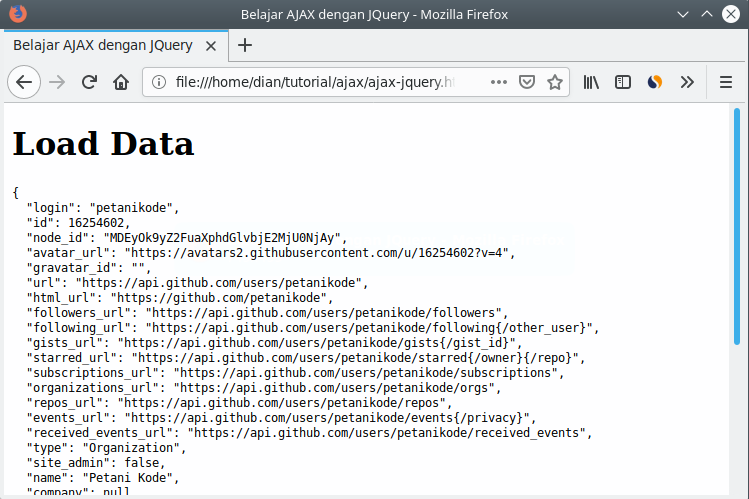
With the function
$("#result").load()
, we can retrieve data with AJAX and directly display it on the selected element.
The JQuery function is
load()
suitable for taking parts of HTML to display.
Another example uses the GET method:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Belajar AJAX dengan JQuery</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.0/jquery.min.js"></script>
</head>
<body>
<h1>Load Data</h1>
<img id="avatar" src="" width="100" height="100">
<br>
<h3 id="name"></h3>
<p id="location"></p>
<script>
var url = "https://api.github.com/users/petanikode";
$.get(url, function(data, status){
$("#avatar").attr("src", data.avatar_url);
$("#name").text(data.name);
$("#location").text(data.location);
});
</script>
</body>
</html>
The result:
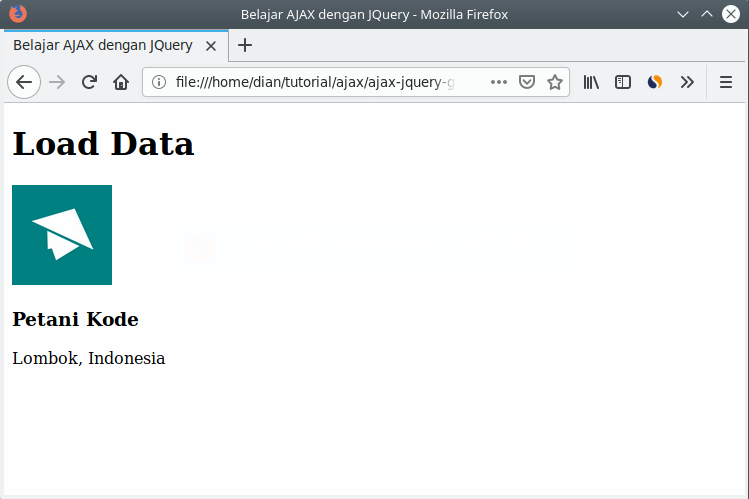
Now to send data with AJAX in JQuery, the way is almost the same as taking data with
$.get()
.<script>
var url = "https://jsonplaceholder.typicode.com/posts";
var data = {
title: "Tutorial AJAX dengan JQuery",
body: "Ini adalah tutorial tentang AJAX",
userId: 1
};
$.post(url, data, function(data, status){
// data terkkirim, lakukan sesuatu di sini
});
</script>
AJAX uses the Fetch API
Fetch means to take. The fetch method can be an alternative to AJAX.
This method starts to appear in the ES6 version of JavaScript.
The difference between
XMLHttpRequest
and JQuery is:- Fetch will return a promise;
- By default (default), fetch will not send or receive cookies from the server. 2
- Fetch can be used in web browsers as well as Nodejs with modules
node-fetch
.
The following is the basic syntax of using Fetch .
fetch('http://example.com/movies.json')
.then(function(response) {
return response.json();
})
.then(function(myJson) {
console.log(JSON.stringify(myJson));
});
And for sending data using the POST method, it looks like this:
fetch(url, {
method: "POST", // *GET, POST, PUT, DELETE, etc.
mode: "cors", // no-cors, cors, *same-origin
cache: "no-cache", // *default, no-cache, reload, force-cache, only-if-cached
credentials: "same-origin", // include, *same-origin, omit
headers: {
"Content-Type": "application/json",
// "Content-Type": "application/x-www-form-urlencoded",
},
redirect: "follow", // manual, *follow, error
referrer: "no-referrer", // no-referrer, *client
body: JSON.stringify(data), // body data type must match "Content-Type" header
})
.then(response => response.json()); // parses JSON response into native Javascript objects
Let's try ...
The following is an example of taking data using fetch :
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Belajar Dasar Ajax dengan Fetch</title>
</head>
<body>
<h1>Tutorial Ajax dengan Fetch</h1>
<button onclick="loadContent()">Load Content</button>
<div id="hasil"></div>
<script>
function loadContent(){
var url = "https://jsonplaceholder.typicode.com/posts/";
fetch(url).then(response => response.json())
.then(function(data){
var template = data.map(post => {
return `
<h3>${post.title}</h3>
<p>${post.body}</p>
<hr>
`;
});
document.getElementById("hasil").innerHTML = template.join('<br>');
}).catch(function(e){
alert("gagal mengambil data");
});
}
</script>
</body>
</html>
The result:
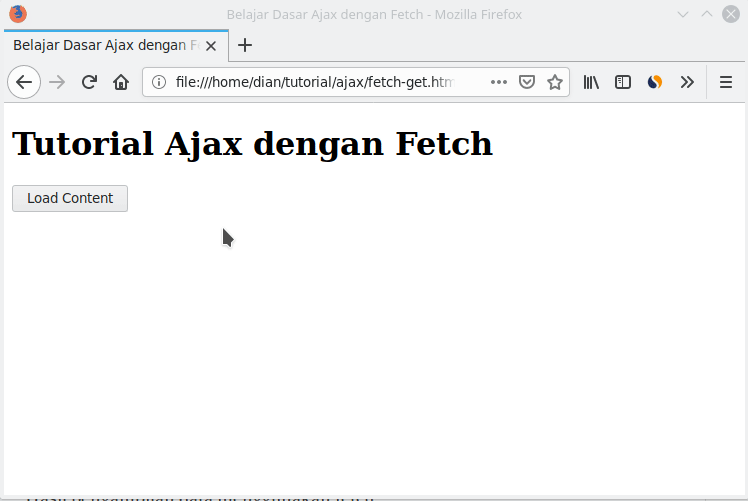
Ajax Using Axios
Axios is almost the same as fetch . The difference is Axios is a library while fetch is an API available in web browsers.
Axios can also be used in both web browsers and Nodejs.
An AJAX example with Axios:
axios.get('https://jsonplaceholder.typicode.com/posts/')
.then(function (response) {
// handle success
console.log(response);
})
.catch(function (error) {
// handle error
console.log(error);
})
.then(function () {
// always executed
});
Let's try it in HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Tutorial AJAX dengan AXIOS</title>
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
</head>
<body>
<h1>Tutorial AJAX dengan AXIOS</h1>
<button id="btn-load" onclick="loadContent()">Load Content</button>
<div id="result"></div>
<script>
function loadContent() {
document.getElementById("btn-load").innerHTML = "loading...";
document.getElementById("btn-load").setAttribute("disabled", "true");
axios.get('https://jsonplaceholder.typicode.com/posts/')
.then(function (response) {
var template = response.data.map(post => {
return `
<h3>${post.title}</h3>
<p>${post.body}</p>
<hr>
`
}).join("");
document.getElementById("result").innerHTML = template;
})
.catch(function (error) {
// handle error
console.log(error);
})
.then(function () {
document.getElementById("btn-load").innerHTML = "Done";
document.getElementById("btn-load").removeAttribute("disabled");
});
}
</script>
</body>
</html>
The result:
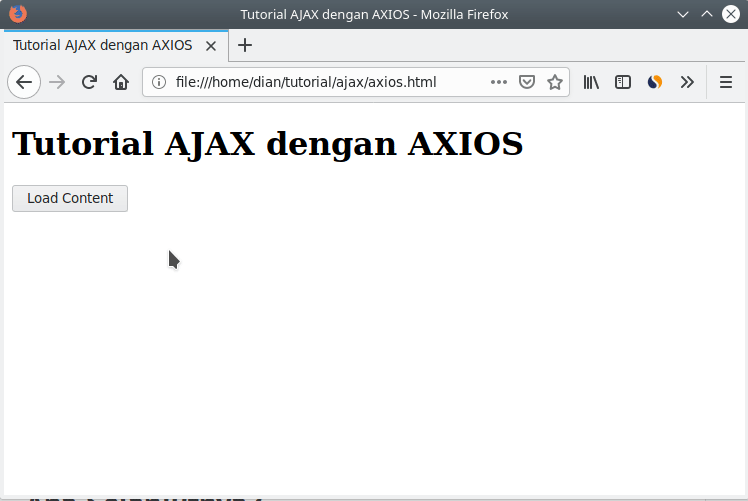
Reference: https://www.petanikode.com/javascript-ajax/
0 Komentar untuk "Learning Javascript: What is AJAX? and How to use it?"
Silahkan berkomentar sesuai artikel