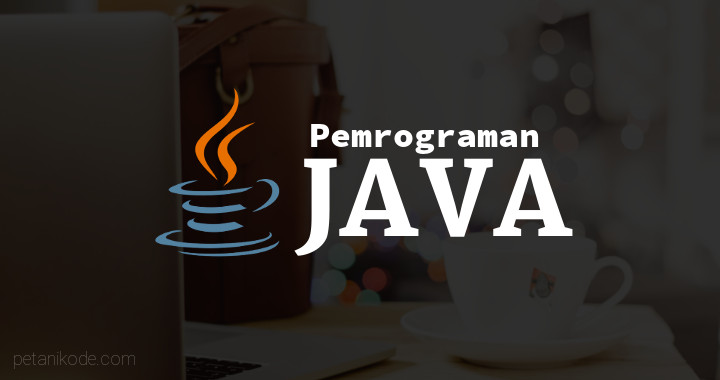
As we know, computer programs consist of three main components, namely: input, process, and output.
- Input: the value we input into the program
- Process: step by step that is done to manage input becomes something useful
- Output: processing results
All programming languages have provided functions for input and output.
Java itself has provided three classes for taking input:
- Class Scanner;
- BufferReader class;
- and Class Console.
The three classes are to take input in a text-based program (console) . While for the GUI, use other classes such as JOptionPane and inputbox on the form.
As for output, Java provides the functions
print()
, println()
, and format()
.Take Input with a Class Scanner
Scanner is a class that provides functions to take input from the keyboard.
So that we can use the Scanner, we need to import it into the code:
import java.util.Scanner;
For further details…
Let's Practice
Now is the 2nd meeting, so make a new package called meetings in source packages .
Right-click on souce packages , then select new package :
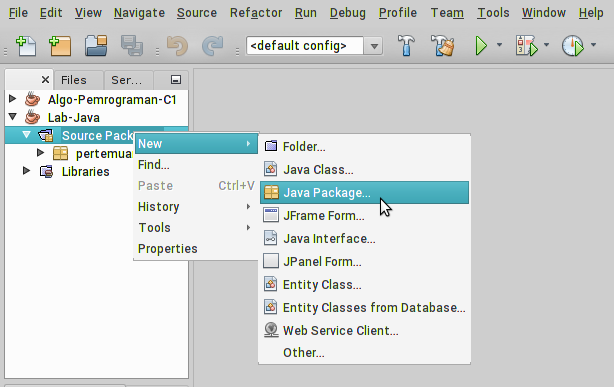
Then fill in the package name with the meeting , then click Finish :
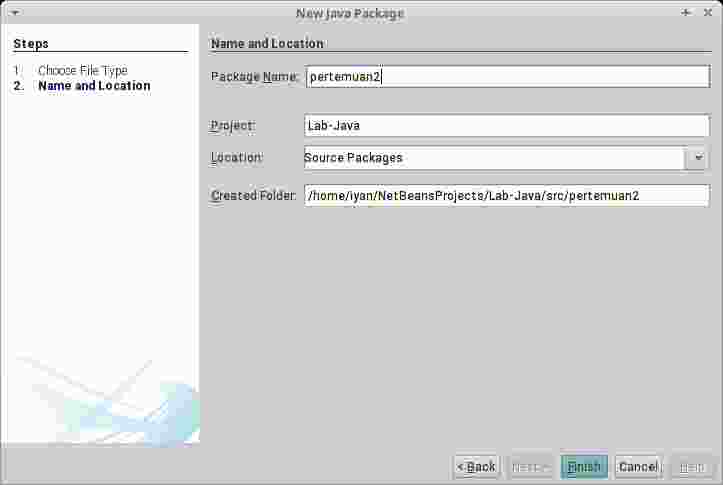
After that, continue by creating a new class (java class) in the meeting package2 :
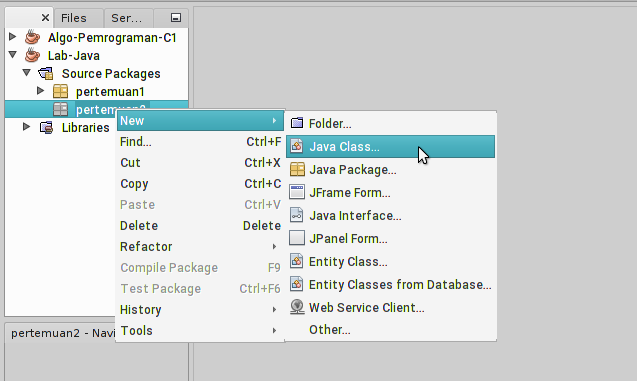
Give name: Employee Data . Then click Finish :
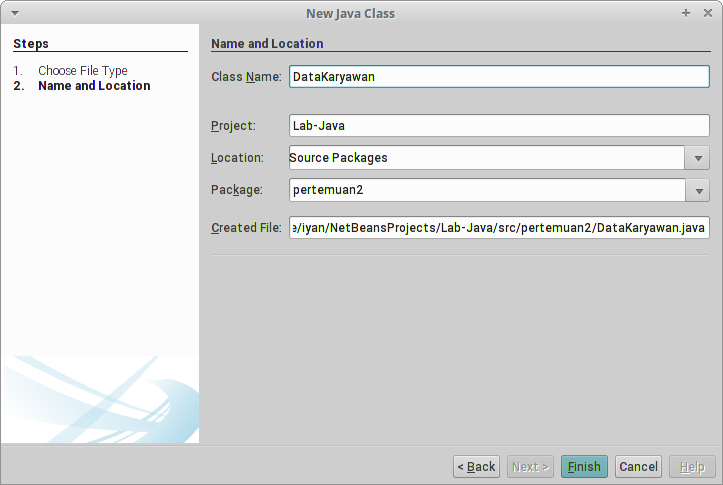
After that, please follow the following code:
package pertemuan2;
// mengimpor Scanner ke program
import java.util.Scanner;
public class DataKaryawan {
public static void main(String[] args) {
// deklarasi variabel
String nama, alamat;
int usia, gaji;
// membuat scanner baru
Scanner keyboard = new Scanner(System.in);
// Tampilkan output ke user
System.out.println("### Pendataan Karyawan PT. Petani Kode ###");
System.out.print("Nama karyawan: ");
// menggunakan scanner dan menyimpan apa yang diketik di variabel nama
nama = keyboard.nextLine();
// Tampilkan outpu lagi
System.out.print("Alamat: ");
// menggunakan scanner lagi
alamat = keyboard.nextLine();
System.out.print("Usia: ");
usia = keyboard.nextInt();
System.out.print("Gaji: ");
gaji = keyboard.nextInt();
// Menampilkan apa yang sudah simpan di variabel
System.out.println("--------------------");
System.out.println("Nama Karyawan: " + nama);
System.out.println("Alamat: " + alamat);
System.out.println("Usia: " + usia + " tahun");
System.out.println("Gaji: Rp " + gaji);
}
}
It should be noted, use the function to retrieve data depending on the data type used.
For example, the data type is a String , then the function or method used is
nextLine()
.
Likewise with other data types, Integers use
nextInt()
, Doubleuses nextDouble()
, etc.
After completing the program, please run. Right-click then select Run File or press the [Shift]+ button [F6].
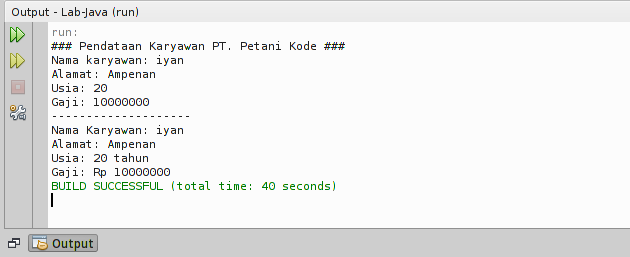
Take Input with the BufferReader Class
The class is
BufferReader
actually not only for taking input from the keyboard.
This class can also be used to read input from files and networks.
This class is located in the package
java.io
.
Please import to use the class
BufferReader
.import java.io.BufferedReader;
Let's try ...
Please create a new class named
ContohBufferReader
, then fill in the following code.package pertemuan2;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class ContohBufferReader {
public static void main(String[] args) throws IOException {
String nama;
// Membuat objek inputstream
InputStreamReader isr = new InputStreamReader(System.in);
// membuat objek bufferreader
BufferedReader br = new BufferedReader(isr);
// Mengisi varibel nama dengan Bufferreader
System.out.print("Inputkan nama: ");
nama = br.readLine();
// tampilkan output isi variabel nama
System.out.println("Nama kamu adalah " + nama);
}
}
It turns out that class
BufferReader
cannot work alone. He also needs friends, namely: class InputStreamReader
and class IOException
.
Now let's try running the program:
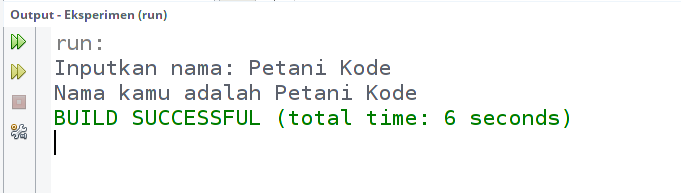
The difference
BufferReader
with is Scanner
seen from the function or method used.Scanner
use next()
, while BufferReader
using readLine()
.
Then for integer data types,
BufferReader
use read()
only functions . We can see this in hint autocomplete .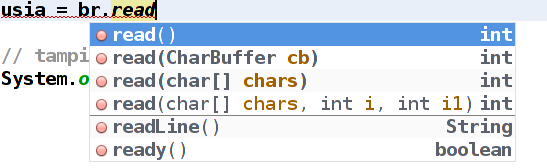
Tip : Press
Ctrl
+ Spasi
when writing code to display hint autocomplete .Take Input with the Class Console
Class is
Console
almost the same as BufferReader
. He also uses a function readLine()
to take input.
However…
This class can only be used in console environments, such as Terminal and CMD.
Class
Console
cannot be used directly in Netbeans.
Therefore, we must compile manually.
To use this class, we need to import it first.
import java.io.Console;
Let's try ...
Make a new file named
InputConsole.java
with the contents as follows:import java.io.Console;
public class InputConsole {
public static void main(String[] args) {
String nama;
int usia;
// membuat objek console
Console con = System.console();
// mengisi variabel nama dan usia dengan console
System.out.print("Inputkan nama: ");
nama = con.readLine();
System.out.print("Inputkan usia: ");
usia = Integer.parseInt(con.readLine());
// mengampilkan isi variabel nama dan usia
System.out.println("Nama kamu adalah: " + nama);
System.out.println("Saat ini berusia " + usia + " tahun");
}
}
Note : in the code above, we use functions
Integer.parseInt(con.readLine())
for integer data types. That is, we change the String data type to Integer.
Because
Console
it has no return value in the form of an integer for the function read()
.
Continue ...
After that, compile through Terminal or CMD. Open the terminal, then go to the directory where the code is stored.
Type the command:
javac InputConsole.java
to compile.
After that, type the command
java InputConsole
to run it: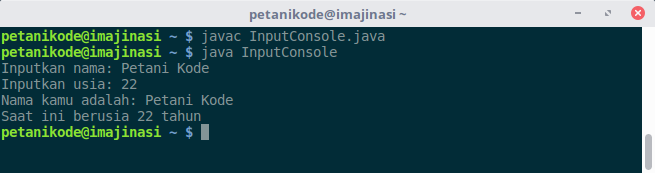
Display Output
We already know several ways to take input from the keyboard for text-based programs.
Now what about the output?
There are several functions that have been provided by Java:
- Function
System.out.print()
- Function
System.out.println()
- Function
System.out.format()
What are the differences in these functions?
Let's discuss ...
print()
Vs functionprintln()
The function
print()
and println()
both are used to display text.
Then what's the difference?
The function
print()
will display text as is. Whereas println()
will display text with added new line.
Let's try in the code:
package eksperimen;
public class PrintVsPrinln {
public static void main(String[] args) {
System.out.print("ini teks yang dicetak dengan print()");
System.out.println("sedangkan ini teks yang dicetak dengan println()");
System.out.print("pake print() lagi");
}
}
Now look at the output:
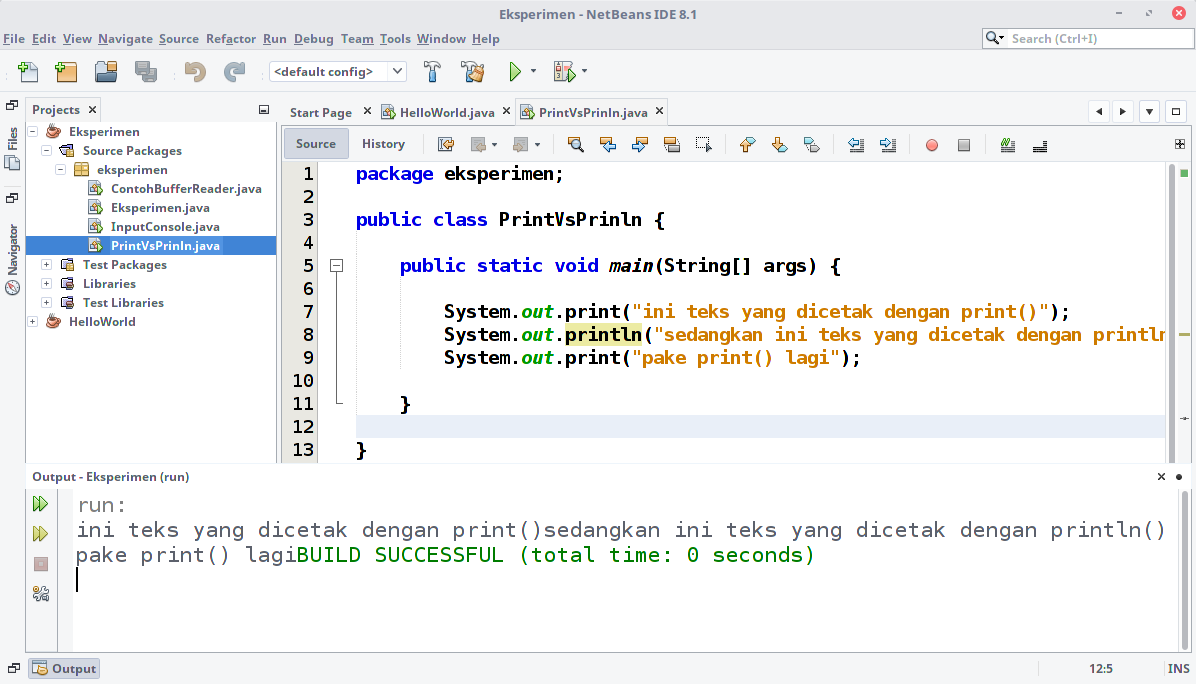
Combining Strings
When using functions
print()
or println()
, sometimes we need to take text from variables and combine them with other text.
For example like this:
We have variables
namaDepan
and namaBelakang
:String namaDepan = "Petani";
String namaBelakang = "Kode";
Then we want to display it with a function
print()
, so we only need to enter it there.System.out.print(namaDepan);
System.out.print(namaBelakang);
The code will produce:
PetaniKode
Actually we don't need to use two functions
print()
, because we can combine them with operators +
.
Example:
System.out.print(namaDepan + namaBelakang);
To have a space, just add a space:
System.out.print(namaDepan + " " + namaBelakang);
String format
Whereas for combining more complex strings, we can use functions
format()
.
Example:
package eksperimen;
public class FormatString {
public static void main(String[] args) {
String namaDepan = "Petani";
String namaBelakang = "Kode";
System.out.format("Nama saya %s %s %n", namaDepan, namaBelakang);
}
}
Note : there we use symbols
%s
to take the value of the variable next to it. %s
meaning string.
Besides
%s
, there are also other symbols:%d
for decimals or numbers;%f
for fractions;%n
for new lines, you can also use it\n
;- and many more, check the java documentation .
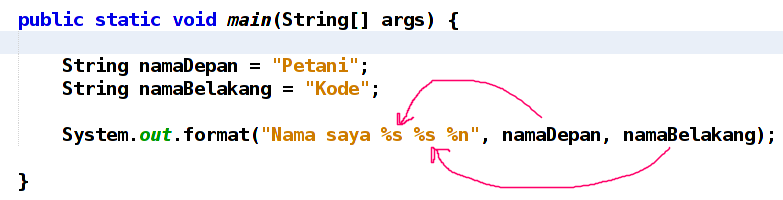
The code above will produce:
Nama saya Petani Kode
0 Komentar untuk "Learning Java: How to Take Input and Display Output"
Silahkan berkomentar sesuai artikel