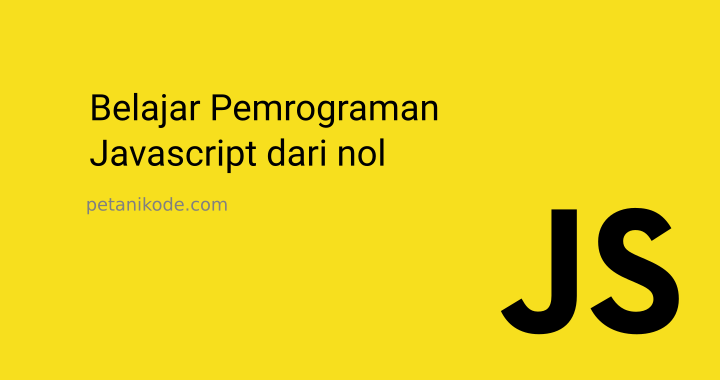
Javascript is a programming language that you must learn if you want to explore the world of web development .
Currently javascript is not only used on the client (browser) side . Javascript is also used on servers, consoles, desktop programs, mobile, IoT, games, and others.
This makes javascript more popular and becomes the most used language on Github.
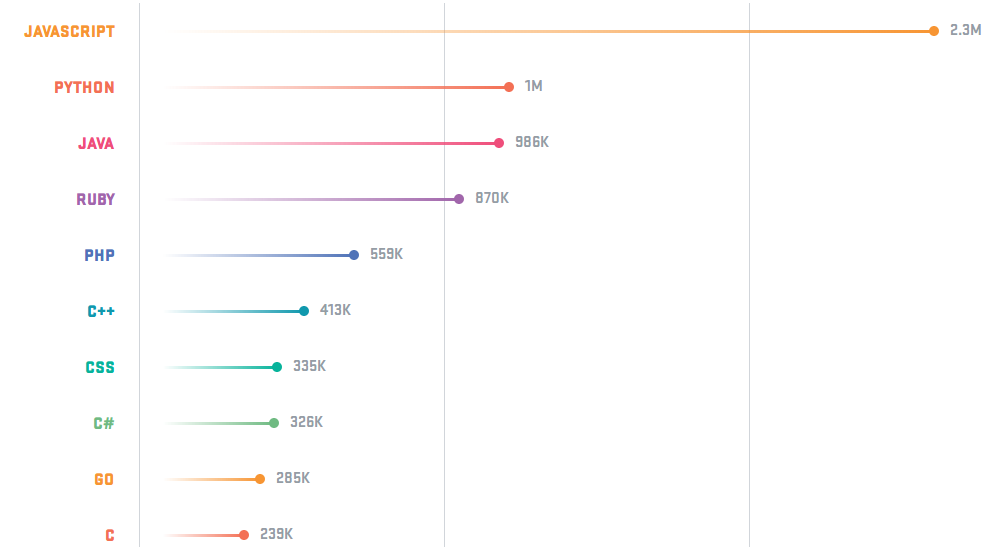
In this article, we will learn Javascript from the ground up. Starting from the introduction of Javascript, to make the first program with Javascript.
Ready?
Let's start…
What is javascript?
Javascript is a programming language that was originally designed to run on a browser.
However, as time goes by, javascript does not only run on the browser. Javascript can also be used on the server side, games, IoT, desktop, etc.
Javascript was originally called Mocha , then changed to LiveScript when the browser Netscape Navigator 2.0 was released in beta (September 1995). However, after that it was renamed Javascript. 1
Inspired by the success of Javascript, Microsoft adopted a similar technology. Microsoft made their own 'Javascript' version called JScript. Then planted on Internet Explorer 3.0.
This has resulted in a ' browser war ', because Microsoft's JScript is different from Netscape's Javascript mix.
Finally in 1996, Netscape sent ECMA-262 standardization to Ecma International . So the standardization of Javascript code was born called ECMAScript or ES. Currently ECMAScript has reached version 8 (ES8). 2
ECMAScript version | Year of Release |
---|---|
ES 1 | June 1997 |
ES 2 | June 1998 |
ES 3 | December 1999 |
ES 4 | Abandoned |
ES 5 | December 2009 |
ES 5.1 | June 2011 |
ES 6 | June 2015 |
ES 7 | June 2016 |
ES 8 | June 2017 |
Tools for Learning Javascript
What are the tools that must be prepared for learning Javascript?
- Web Browser (Google Chrome, Firefox, Opera, etc.)
- Text Editor (recommendation: VS Code )
That is all?
Yes, that's enough. If you want to learn Javacript from Nodejs, please read: Introduction to Nodejs for Beginners .
My recommendation: learn Javascript from the client side first. Later Nodejs later.
Get to know the JavaScript Console
Some say, learning javascript is difficult, because when you see the results in a web browser, the error message does not appear. This opinion is incorrect. Because we can see it through the console .
We can open the Javascript Console through Inspect Element-> Console .
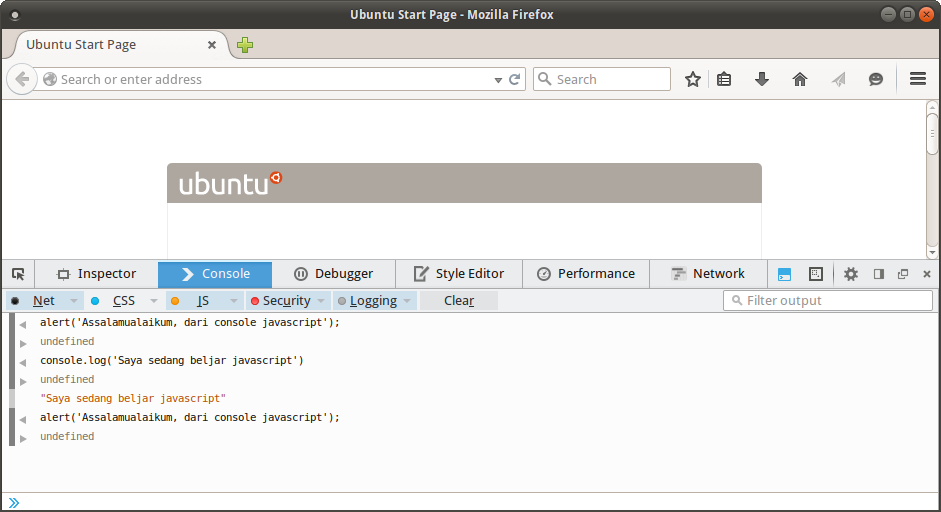
Inside the console , we can write functions or javascript codes and the results will be immediately displayed.
For example, let's try the following code:
console.log("Hi apa kabar!");
alert("Saya sedang belajar javascript");
Then the result:
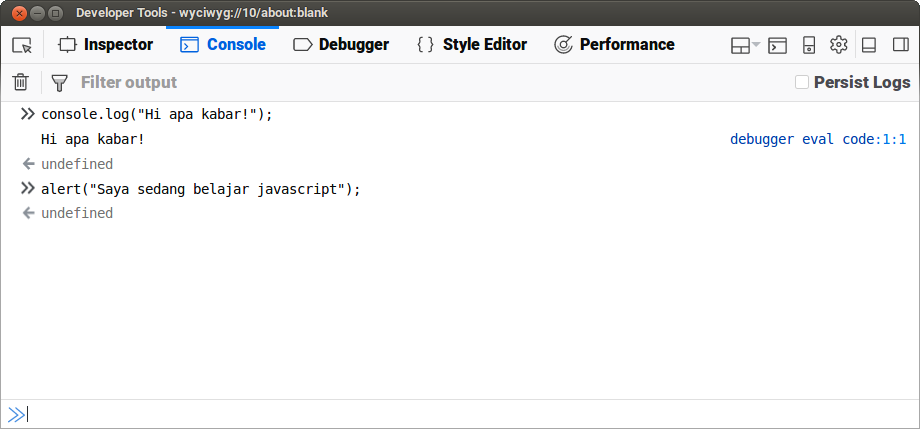
If you use Nodejs, then how to access the console is to type the command
node
in Terminal.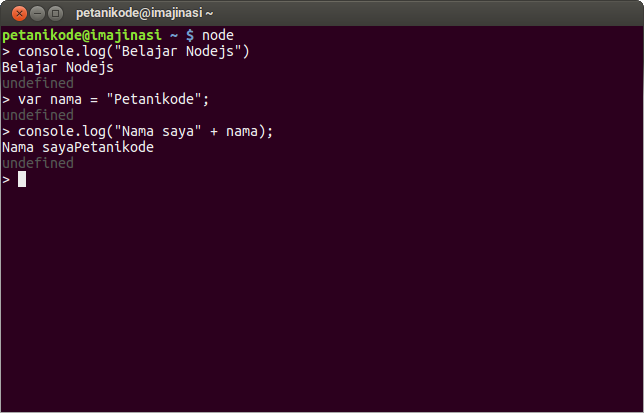
After trying the Javascript console , we can conclude:
- The console can be used to test functions or Javascript code;
- We can use the Console to see error messages when debugging programs.
- ... what else?
Creating the First Javascript Program
Already know how to open and use a javascript console?
Nice…
Then, let's create the first program with Javascript.
Please open the text editor, then create a name file
hello_world.html
and fill in the following code:<!DOCTYPE html>
<html>
<head>
<title>Hello World Javascript</title>
</head>
<body>
<script>
console.log("Saya belajar Javascript");
document.write("Hello World!");
</script>
</body>
</html>
If you use the text editor VS Code, it will look like this:
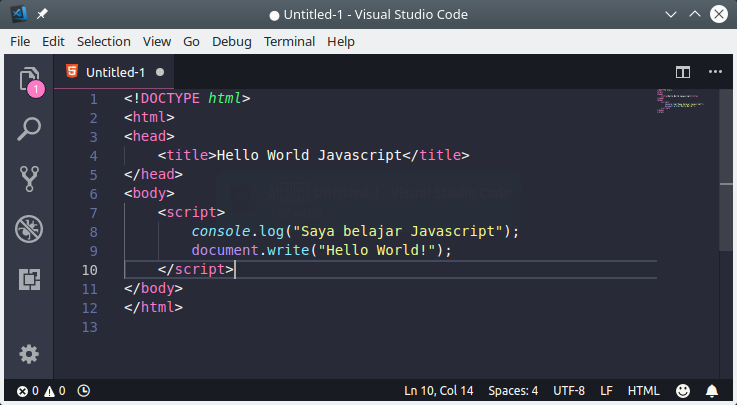
Please save with a name
hello_world.html
, then open the file with a web browser.
Then the result:
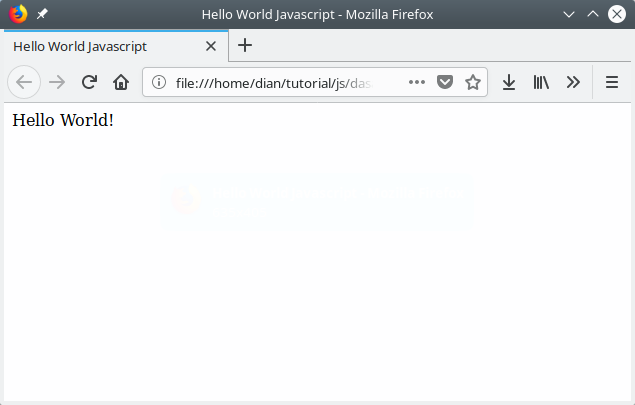
Wait a minute ...
Earlier we wrote the command:
console.log("Saya belajar Javascript");
Why isn't it displayed?
Because the command or function
console.log()
will display the message in the javascript console . While the command document.write()
functions to write to an HTML document, then it will be displayed there.
Now just open the javascript console.
Then we will see a message
"Saya belajar Javascript"
: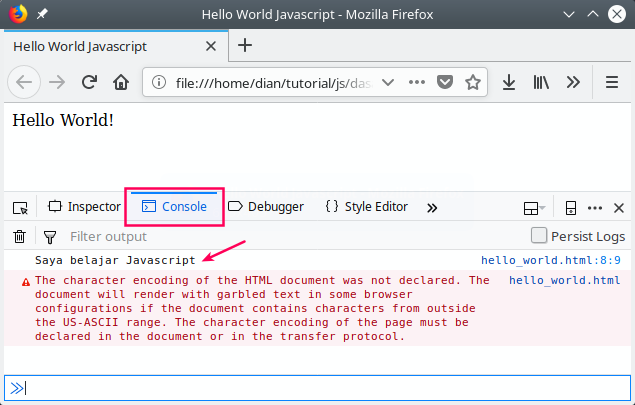
Steady… 
We already know the function
document.write()
and console.log()
, later we will discuss more about this function in:
Now, what we need to know next is:
How to write Javascript code in HTML?
In the example above, we have written javascript code in HTML.
This method is an embeded method .
There are still some more ways that we need to know:
- Embed (Javascript code pasted directly on HTML. Example: the one before)
- Inline (Javascript code written in HTML attributes)
- External (Javascript code is written separately from HTML file)
Let's look at an example ...
Also read: 4 Ways to Write Javascript in HTML
1. Writing javascript code with Embed
In this way, we use tags
<script>
to embed the Javascript code in HTML. This tag can be written in tags <head>
and <body>
.
Example:
<!DOCTYPE html>
<html>
<head>
<title>Belajar Javascript dari Nol</title>
<script>
// ini adalah penulisan kode javascript
// di dalam tag <head>
console.log("Hello JS dari Head");
</script>
</head>
<body>
<p>Tutorial Javascript untuk Pemula</p>
<script>
// ini adalah penulisan kode javascript
// di dalam tag <body>
console.log("Hello JS dari body");
</script>
</body>
</html>
Which is better, written inside
<head>
or <body>
?
Many recommend writing it inside
<body>
, because it will make the web load faster.2. Writing inline javascript code
In this way, we will write the javascript code in the HTML attribute. This method is usually used to call a function for a particular event .
For example: when the link is clicked.
Example:
<a href="#" onclick="alert('Yey!')">Klik aku!</a>
or it could be like this:
<a href="javascript:alert('Yey!')">Klik aku!</a>
The result:
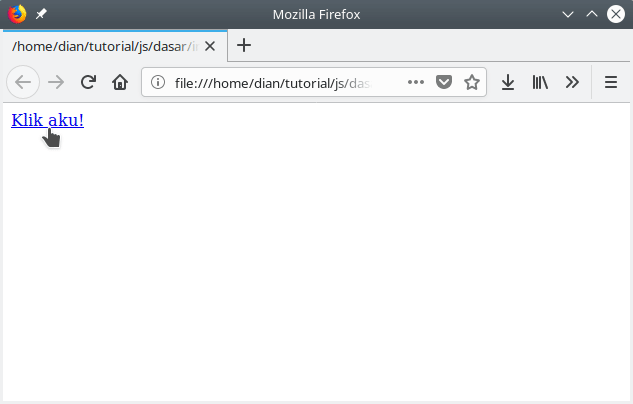
Pay attention ...
On the attribute
onclick
and href
we write the javascript function there.
Attribute
onclick
is an HTML attribute to declare a function that will be executed when the element is clicked.
In the example above, we perform the function
alert()
. This function is a function for displaying dialogs .
Then in the attribute
href
, we also call the functionalert()
preceded javascript:
.
The attribute is
href
actually used to fill in the link address or URL.
Because we want to call the javascript code there, then we change the link address
javascript:
then followed by the function to be called.3. Writing External JavaScript Code
In this way, we will write the javascript code separately with the HTML file.
This method is usually used on large projects, because it is believed - in this way - can more easily manage the project code.
Let's look at an example ...
We create two files, namely: HTML and Javascript files.
belajar-js/
├── kode-program.js
└── index.html
The contents of the file
kode-program.js
:alert("Hello, ini adalah program JS eksternal!");
The contents of the file
index.html
:<!DOCTYPE html>
<html>
<head>
<title>Belajar Javascript dari Nol</title>
</head>
<body>
<p>Tutorial Javascript untuk Pemula</p>
<!-- Menyisipkan kode js eksternal -->
<script src="kode-program.js"></script>
</body>
</html>
The result:
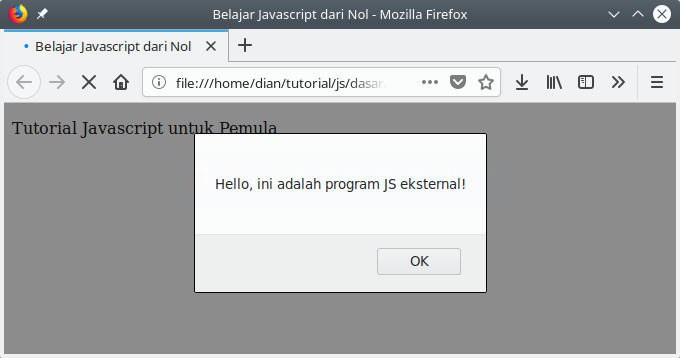
In the example above, we write separate javascript code with HTML code.
Then, in the HTML code ...
We insert it by giving attributes
src
to the tag <script>
.<!-- Menyisipkan kode js eksternal -->
<script src="kode-program.js"></script>
So, anything in the file
kode-program.js
will be read from the file index.html
.
What if the javascript file is in a different folder?
We can write the full address of the folder.
Example:
Suppose we have a folder structure like this:
belajar-js/
├── js/
| └── kode-program.js
└── index.html
So to insert files
kode-program.js
into HTML, we can write them like this:<script src="js/kode-program.js"></script>
Because the file
kode-program.js
is in the directory js
.
We can also insert javascript on the internet by providing the full URL address.
Example:
<script src="https://www.petanikode.com/js/kode.js"></script>
Reference: https://www.petanikode.com/javascript-dasar/
0 Komentar untuk "Javascript Programming: The First Step in Learning Javascript"
Silahkan berkomentar sesuai artikel